
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
storage.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __STORAGE_H__ 00018 #define __STORAGE_H__ 00019 00020 #include <inttypes.h> 00021 #include "key_config_manager.h" 00022 #include "kcm_internal.h" 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 // FIXME: change to esfs define when a new esfs release is out with ESFS_MAX_NAME_LENGTH defined in esfs.h 00029 /* 00030 * #include "esfs.h" 00031 * #define STORAGE_FILENAME_MAX_SIZE ESFS_MAX_NAME_LENGTH 00032 */ 00033 00034 #define STORAGE_FILENAME_MAX_SIZE 1024 00035 00036 #if ((STORAGE_FILENAME_MAX_SIZE - KCM_FILE_PREFIX_MAX_SIZE) != KCM_MAX_FILENAME_SIZE) 00037 #error "ESFS_MAX_NAME_LENGTH must be equal to KCM_FILE_PREFIX_MAX_SIZE + KCM_MAX_FILENAME_SIZE. Perhaps there was a change in one of the defines." 00038 #endif 00039 00040 00041 /* === Initialization and Finalization === */ 00042 00043 /** Initializes storage so that it can be used. 00044 * Must be called once after boot. 00045 * Existing data in storage would not compromised. 00046 * 00047 * @returns 00048 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00049 */ 00050 kcm_status_e storage_init(void); 00051 00052 00053 /** Finalize storage. 00054 * Must be called once to close all storage resources. 00055 * 00056 * @returns 00057 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00058 */ 00059 kcm_status_e storage_finalize(void); 00060 00061 /** Resets storage to an empty state. 00062 * 00063 * @returns 00064 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00065 */ 00066 kcm_status_e storage_reset(void); 00067 00068 00069 /** Resets storage to a factory state. 00070 * 00071 * @returns 00072 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00073 */ 00074 kcm_status_e storage_factory_reset(void); 00075 00076 00077 /* === File Operations === */ 00078 00079 /** Create a new file 00080 * 00081 * @param KCM operation context. 00082 * @param file_name A binary blob that uniquely identifies the file 00083 * @param file_name_length The binary blob length in bytes. 00084 * @param meta_data_list A pointer to structure with single meta data for each type 00085 * @param is_factory A factory flag. 00086 * @param is_encrypted Encryption flag 00087 * 00088 * @returns 00089 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00090 */ 00091 kcm_status_e storage_file_create(kcm_ctx_s *ctx, const uint8_t *file_name, size_t file_name_length, const kcm_meta_data_list_s *kcm_meta_data_list, bool is_factory, bool is_encrypted); 00092 00093 /** Open existing file 00094 * 00095 * @param KCM operation context. 00096 * @param file_name A binary blob that uniquely identifies the file 00097 * @param file_name_length The binary blob length in bytes. 00098 * 00099 * @returns 00100 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00101 */ 00102 kcm_status_e storage_file_open(kcm_ctx_s *ctx, const uint8_t *file_name, size_t file_name_length); 00103 00104 /** Close file in storage 00105 * 00106 * @param ctx KCM operation context. 00107 * 00108 * @returns 00109 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00110 */ 00111 kcm_status_e storage_file_close(kcm_ctx_s *ctx); 00112 00113 /** Write data to previously opened file in storage 00114 * 00115 * @param ctx KCM operation context. 00116 * @param data A pointer to memory with the data to write into the newly created file. Can be NULL if data_length is 0. 00117 * @param data_length The data length in bytes. Can be 0 if we wish to write an empty file. 00118 * 00119 * @returns 00120 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00121 */ 00122 kcm_status_e storage_file_write_with_ctx(kcm_ctx_s *ctx, const uint8_t *data, size_t data_length); 00123 00124 00125 /** Writes a new file to storage 00126 * 00127 * @param ctx KCM operation context. 00128 * @param file_name A binary blob that uniquely identifies the file 00129 * @param file_name_length The binary blob length in bytes. 00130 * @param data A pointer to memory with the data to write into the newly created file. Can be NULL if data_length is 0. 00131 * @param data_length The data length in bytes. Can be 0 if we wish to write an empty file. 00132 * @param meta_data_list A pointer to structure with single meta data for each type 00133 * @param is_factory True if KCM item is factory item, or false otherwise 00134 * @param is_encrypted True if KCM item should be encrypted, or false otherwise 00135 * 00136 * @returns 00137 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00138 */ 00139 kcm_status_e storage_file_write(kcm_ctx_s *ctx, const uint8_t *file_name, size_t file_name_length, const uint8_t *data, size_t data_length, const kcm_meta_data_list_s *kcm_meta_data_list, bool is_factory, bool is_encrypted); 00140 00141 00142 /** Returns the size of the data in a file 00143 * 00144 * @param ctx KCM operation context. 00145 * @param file_name A binary blob that uniquely identifies the file 00146 * @param file_name_length The binary blob length in bytes 00147 * @param file_size_out A pointer to hold the size of the data in the file 00148 * 00149 * @returns 00150 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00151 */ 00152 kcm_status_e storage_file_size_get(kcm_ctx_s *ctx, const uint8_t *file_name, size_t file_name_length, size_t *file_size_out); 00153 00154 /** Reads data from a file. 00155 * 00156 * @param ctx KCM operation context. 00157 * @param file_name A binary blob that uniquely identifies the file 00158 * @param file_name_length The binary blob length in bytes 00159 * @param buffer_out A pointer to memory buffer where the data will be read from the file. Can be NULL if buffer_size is 0. 00160 * @param buffer_size The number of bytes to be read. Buffer must be big enough to contain this size. Can be 0 if we wish to read an empty file. 00161 * @param buffer_actual_size_out The effective bytes size read from the file. 00162 * 00163 * @returns 00164 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00165 */ 00166 kcm_status_e storage_file_read(kcm_ctx_s *ctx, const uint8_t *file_name, size_t file_name_length, uint8_t *buffer_out, size_t buffer_size, size_t *buffer_actual_size_out); 00167 00168 /** Returns the size of the data in a file. The file should be opened by storage_file_open() 00169 * 00170 * @param ctx KCM operation context. 00171 * @param file_size_out A pointer to hold the size of the data in the file 00172 * 00173 * @returns 00174 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00175 */ 00176 kcm_status_e storage_file_size_get_with_ctx(kcm_ctx_s *ctx, size_t *file_size_out); 00177 00178 /** Reads data from a file. The file should be opened by storage_file_open(). 00179 * 00180 * @param ctx KCM operation context. 00181 * @param buffer_out A pointer to memory buffer where the data will be read from the file. Can be NULL if buffer_size is 0. 00182 * @param buffer_size The number of bytes to be read. Buffer must be big enough to contain this size. Can be 0 if we wish to read an empty file. 00183 * @param buffer_actual_size_out The effective bytes size read from the file. 00184 * 00185 * @returns 00186 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00187 */ 00188 kcm_status_e storage_file_read_with_ctx(kcm_ctx_s *ctx, uint8_t *buffer_out, size_t buffer_size, size_t *buffer_actual_size_out); 00189 /** Deletes the file from storage 00190 * 00191 * @param ctx KCM operation context. 00192 * @param file_name A binary blob that uniquely identifies the file 00193 * @param file_name_length The binary blob length in bytes 00194 * 00195 * @returns 00196 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00197 */ 00198 kcm_status_e storage_file_delete(kcm_ctx_s *ctx, const uint8_t *file_name, size_t file_name_length); 00199 00200 /** Get the size of the stored meta data type. The file should be opened by storage_file_open(). 00201 * 00202 * @param ctx KCM operation context. 00203 * @param type the meta data type to get size for. 00204 * @param metadata_size_out A pointer to hold the size of the meta data 00205 * 00206 * @returns 00207 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00208 */ 00209 kcm_status_e storage_file_get_meta_data_size(kcm_ctx_s *ctx, kcm_meta_data_type_e type, size_t *meta_data_size_out); 00210 00211 /** Reads meta data into a kcm_meta_data 00212 * 00213 * @param ctx KCM operation context. 00214 * @param type the meta data type to get size for. 00215 * @param buffer_out A pointer to memory buffer where the meta data will be read to. 00216 * @param buffer_size The number of bytes to be read. Buffer must be big enough to contain this size. 00217 * @param buffer_actual_size_out The effective bytes size read from the file. 00218 * 00219 * @returns 00220 * KCM_STATUS_SUCCESS in case of success otherwise one of kcm_status_e errors 00221 */ 00222 kcm_status_e storage_file_read_meta_data_by_type(kcm_ctx_s *ctx, kcm_meta_data_type_e type, uint8_t *buffer_out, size_t buffer_size, size_t *buffer_actual_size_out); 00223 00224 #ifdef __cplusplus 00225 } 00226 #endif 00227 00228 #endif //__STORAGE_H__
Generated on Tue Jul 12 2022 19:12:16 by
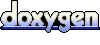