
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
sotp.h
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __SOTP_H 00018 #define __SOTP_H 00019 00020 #include <stdint.h> 00021 #include "pal.h" 00022 00023 #define SYS_CONF_SOTP_DISABLED 0 00024 #define SYS_CONF_SOTP_ENABLED 1 00025 #define SYS_CONF_SOTP_LIMITED 2 00026 00027 #if PAL_USE_INTERNAL_FLASH 00028 #ifndef SYS_CONF_SOTP 00029 #define SYS_CONF_SOTP SYS_CONF_SOTP_ENABLED 00030 #endif 00031 #else 00032 #ifndef SYS_CONF_SOTP 00033 #define SYS_CONF_SOTP SYS_CONF_SOTP_LIMITED 00034 #endif 00035 #endif 00036 00037 #ifdef SOTP_TESTING 00038 #undef SOTP_PROBE_ONLY 00039 #endif 00040 00041 typedef enum { 00042 SOTP_SUCCESS = 0, 00043 SOTP_READ_ERROR = 1, 00044 SOTP_WRITE_ERROR = 2, 00045 SOTP_NOT_FOUND = 3, 00046 SOTP_DATA_CORRUPT = 4, 00047 SOTP_BAD_VALUE = 5, 00048 SOTP_BUFF_TOO_SMALL = 6, 00049 SOTP_FLASH_AREA_TOO_SMALL = 7, 00050 SOTP_OS_ERROR = 8, 00051 SOTP_BUFF_NOT_ALIGNED = 9, 00052 SOTP_ALREADY_EXISTS = 10, 00053 SOTP_ERROR_MAXVAL = 0xFFFF 00054 } sotp_result_e; 00055 00056 #define SOTP_NUM_AREAS PAL_INT_FLASH_NUM_SECTIONS 00057 00058 // Each type can be either OTP (One Time programmed) or RBP (RollBack protected) 00059 typedef enum { 00060 SOTP_TYPE_FACTORY_DONE = 0, 00061 SOTP_TYPE_RANDOM_SEED, 00062 SOTP_TYPE_SAVED_TIME, 00063 SOTP_TYPE_LAST_TIME_BACK, 00064 SOTP_TYPE_ROT, 00065 SOTP_TYPE_TRUSTED_TIME_SRV_ID, 00066 SOTP_TYPE_EXECUTION_MODE, 00067 SOTP_TYPE_OEM_TRANSFER_MODE_ENABLED, 00068 SOTP_TYPE_MIN_FW_VERSION, 00069 00070 SOTP_LAST_TYPE = 15, // Keep as long as we have less than this 00071 SOTP_MAX_TYPES 00072 } sotp_type_e; 00073 00074 #define SOTP_BLANK_FLASH_VAL PAL_INT_FLASH_BLANK_VAL 00075 00076 00077 #ifdef __cplusplus 00078 extern "C" { 00079 #endif 00080 00081 // Check whether a type is an OTP type. 00082 /** 00083 * @brief Check whether a type is an OTP type (can be written once) or RBP (more than once). 00084 * 00085 * @param [in] type 00086 * Given type. 00087 * 00088 * @returns false Type is RBP (can be written more than once). 00089 * true Type is OTP (can be written only once). 00090 */ 00091 bool sotp_is_otp_type(uint32_t type); 00092 00093 /** 00094 * @brief Returns one item of data programmed on Flash, given type. 00095 * 00096 * @param [in] type 00097 * Type of stored item (must be between 0-15). 00098 * 00099 * @param [in] buf_len_bytes 00100 * Length of input buffer in bytes. 00101 * 00102 * @param [in] buf 00103 * Buffer to store data on (must be aligned to a 32 bit boundary). 00104 * 00105 * @param [out] actual_len_bytes. 00106 * Actual length of returned data 00107 * 00108 * @returns SOTP_SUCCESS Value was found on Flash. 00109 * SOTP_NOT_FOUND Value was not found on Flash. 00110 * SOTP_READ_ERROR Physical error reading data. 00111 * SOTP_DATA_CORRUPT Data on Flash is corrupt. 00112 * SOTP_BAD_VALUE Bad value in any of the parameters. 00113 * SOTP_BUFF_TOO_SMALL Not enough memory in user buffer. 00114 * SOTP_BUFF_NOT_ALIGNED Buffer not aligned to 32 bits. 00115 */ 00116 sotp_result_e sotp_get(uint32_t type, uint16_t buf_len_bytes, uint32_t *buf, uint16_t *actual_len_bytes); 00117 00118 /** 00119 * @brief Returns one item of data programmed on Flash, given type. 00120 * 00121 * @param [in] type 00122 * Type of stored item. 00123 * 00124 * @param [out] actual_len_bytes. 00125 * Actual length of item 00126 * 00127 * @returns SOTP_SUCCESS Value was found on Flash. 00128 * SOTP_NOT_FOUND Value was not found on Flash. 00129 * SOTP_READ_ERROR Physical error reading data. 00130 * SOTP_DATA_CORRUPT Data on Flash is corrupt. 00131 * SOTP_BAD_VALUE Bad value in any of the parameters. 00132 */ 00133 sotp_result_e sotp_get_item_size(uint32_t type, uint16_t *actual_len_bytes); 00134 00135 00136 /** 00137 * @brief Programs one item of data on Flash, given type. 00138 * 00139 * @param [in] type 00140 * Type of stored item (must be between 0-15). 00141 * 00142 * @param [in] buf_len_bytes 00143 * Item length in bytes. 00144 * 00145 * @param [in] buf 00146 * Buffer containing data (must be aligned to a 32 bit boundary). 00147 * 00148 * @returns SOTP_SUCCESS Value was successfully written on Flash. 00149 * SOTP_WRITE_ERROR Physical error writing data. 00150 * SOTP_BAD_VALUE Bad value in any of the parameters. 00151 * SOTP_FLASH_AREA_TOO_SMALL 00152 * Not enough space in Flash area. 00153 * SOTP_BUFF_NOT_ALIGNED Buffer not aligned to 32 bits. 00154 * SOTP_ALREADY_EXISTS Item (OTP type) already exists. 00155 * 00156 */ 00157 sotp_result_e sotp_set(uint32_t type, uint16_t buf_len_bytes, const uint32_t *buf); 00158 00159 #ifdef SOTP_TESTING 00160 /** 00161 * @brief Delete an item from flash. 00162 * 00163 * @param [in] type 00164 * Type of stored item (must be between 0-15). 00165 * 00166 * @param [in] buf_len_bytes 00167 * Item length in bytes. 00168 * 00169 * @param [in] buf 00170 * Buffer containing data (must be aligned to a 32 bit boundary). 00171 * 00172 * @returns SOTP_SUCCESS Value was successfully written on Flash. 00173 * SOTP_WRITE_ERROR Physical error writing data. 00174 * SOTP_BAD_VALUE Bad value in any of the parameters. 00175 * SOTP_FLASH_AREA_TOO_SMALL 00176 * Not enough space in Flash area. 00177 * SOTP_BUFF_NOT_ALIGNED Buffer not aligned to 32 bits. 00178 * 00179 */ 00180 sotp_result_e sotp_delete(uint32_t type); 00181 00182 /** 00183 * @brief Programs one item of data on Flash, given type. No OTP existing check. 00184 * 00185 * @param [in] type 00186 * Type of stored item (must be between 0-15). 00187 * 00188 * @param [in] buf_len_bytes 00189 * Item length in bytes. 00190 * 00191 * @param [in] buf 00192 * Buffer containing data (must be aligned to a 32 bit boundary). 00193 * 00194 * @returns SOTP_SUCCESS Value was successfully written on Flash. 00195 * SOTP_WRITE_ERROR Physical error writing data. 00196 * SOTP_BAD_VALUE Bad value in any of the parameters. 00197 * SOTP_FLASH_AREA_TOO_SMALL 00198 * Not enough space in Flash area. 00199 * SOTP_BUFF_NOT_ALIGNED Buffer not aligned to 32 bits. 00200 * 00201 */ 00202 sotp_result_e sotp_set_for_testing(uint32_t type, uint16_t buf_len_bytes, const uint32_t *buf); 00203 #endif 00204 00205 /** 00206 * @brief Initializes SOTP component. 00207 * 00208 * @returns SOTP_SUCCESS Initialization completed successfully. 00209 * SOTP_READ_ERROR Physical error reading data. 00210 * SOTP_WRITE_ERROR Physical error writing data (on recovery). 00211 * SOTP_FLASH_AREA_TOO_SMALL 00212 * Not enough space in Flash area. 00213 */ 00214 sotp_result_e sotp_init(void); 00215 00216 /** 00217 * @brief Deinitializes SOTP component. 00218 * Warning: This function is not thread safe and should not be called 00219 * concurrently with other SOTP functions. 00220 * 00221 * @returns SOTP_SUCCESS Deinitialization completed successfully. 00222 */ 00223 sotp_result_e sotp_deinit(void); 00224 00225 /** 00226 * @brief Reset Flash SOTP areas. 00227 * Warning: This function is not thread safe and should not be called 00228 * concurrently with other SOTP functions. 00229 * 00230 * @returns SOTP_SUCCESS Reset completed successfully. 00231 * SOTP_READ_ERROR Physical error reading data. 00232 * SOTP_WRITE_ERROR Physical error writing data. 00233 */ 00234 sotp_result_e sotp_reset(void); 00235 00236 #ifdef SOTP_TESTING 00237 00238 /** 00239 * @brief Initiate a forced garbage collection. 00240 * 00241 * @returns SOTP_SUCCESS GC completed successfully. 00242 * SOTP_READ_ERROR Physical error reading data. 00243 * SOTP_WRITE_ERROR Physical error writing data. 00244 * SOTP_FLASH_AREA_TOO_SMALL 00245 * Not enough space in Flash area. 00246 */ 00247 sotp_result_e sotp_force_garbage_collection(void); 00248 #endif 00249 00250 /** 00251 * @brief Returns one item of data programmed on Flash, given type. 00252 * This is the "initless" version of the function, traversing the flash if triggered. 00253 * 00254 * @param [in] type 00255 * Type of stored item (must be between 0-15). 00256 * 00257 * @param [in] buf_len_bytes 00258 * Length of input buffer in bytes. 00259 * 00260 * @param [in] buf 00261 * Buffer to store data on (must be aligned to a 32 bit boundary). 00262 * 00263 * @param [out] actual_len_bytes. 00264 * Actual length of returned data 00265 * 00266 * @returns SOTP_SUCCESS Value was found on Flash. 00267 * SOTP_NOT_FOUND Value was not found on Flash. 00268 * SOTP_READ_ERROR Physical error reading data. 00269 * SOTP_DATA_CORRUPT Data on Flash is corrupt. 00270 * SOTP_BAD_VALUE Bad value in any of the parameters. 00271 * SOTP_BUFF_TOO_SMALL Not enough memory in user buffer. 00272 * SOTP_BUFF_NOT_ALIGNED Buffer not aligned to 32 bits. 00273 */ 00274 sotp_result_e sotp_probe(uint32_t type, uint16_t buf_len_bytes, uint32_t *buf, uint16_t *actual_len_bytes); 00275 00276 00277 #ifdef __cplusplus 00278 } 00279 #endif 00280 00281 #endif 00282 00283
Generated on Tue Jul 12 2022 19:12:15 by
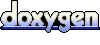