
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
randLIB.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /** 00018 * \file randLIB.h 00019 * \brief Pseudo Random Library API: 00020 * 00021 * 00022 * \section net-boot Network Bootstrap Control API: 00023 * - randLIB_seed_random(), Set seed for pseudo random 00024 * - randLIB_get_8bit(), Generate 8-bit random number 00025 * - randLIB_get_16bit(),Generate 16-bit random number 00026 * - randLIB_get_32bit(),Generate 32-bit random number 00027 * - randLIB_get_n_bytes_random(), Generate n-bytes random numbers 00028 * 00029 */ 00030 00031 #ifndef RANDLIB_H_ 00032 #define RANDLIB_H_ 00033 00034 #include <stdint.h> 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 * This library is made for getting random numbers for Timing needs in protocols. 00042 * 00043 * **not safe to use for security or cryptographic operations.** 00044 * 00045 */ 00046 00047 00048 /** 00049 * \brief Init seed for Pseudo Random. 00050 * 00051 * Makes call(s) to the platform's arm_random_seed_get() to seed the 00052 * pseudo-random generator. 00053 * 00054 * \return None 00055 * 00056 */ 00057 extern void randLIB_seed_random(void); 00058 00059 /** 00060 * \brief Update seed for pseudo-random generator 00061 * 00062 * Adds seed information to existing generator, to perturb the 00063 * sequence. 00064 * \param seed 64 bits of data to add to the seed. 00065 */ 00066 extern void randLIB_add_seed(uint64_t seed); 00067 00068 /** 00069 * \brief Generate 8-bit random number. 00070 * 00071 * \param None 00072 * \return 8-bit random number 00073 * 00074 */ 00075 extern uint8_t randLIB_get_8bit(void); 00076 00077 /** 00078 * \brief Generate 16-bit random number. 00079 * 00080 * \param None 00081 * \return 16-bit random number 00082 * 00083 */ 00084 extern uint16_t randLIB_get_16bit(void); 00085 00086 /** 00087 * \brief Generate 32-bit random number. 00088 * 00089 * \param None 00090 * \return 32-bit random number 00091 * 00092 */ 00093 extern uint32_t randLIB_get_32bit(void); 00094 00095 /** 00096 * \brief Generate 64-bit random number. 00097 * 00098 * \param None 00099 * \return 64-bit random number 00100 * 00101 */ 00102 extern uint64_t randLIB_get_64bit(void); 00103 00104 /** 00105 * \brief Generate n-bytes random numbers. 00106 * 00107 * \param data_ptr pointer where random will be stored 00108 * \param count how many bytes need random 00109 * 00110 * \return data_ptr 00111 */ 00112 extern void *randLIB_get_n_bytes_random(void *data_ptr, uint8_t count); 00113 00114 /** 00115 * \brief Generate a random number within a range. 00116 * 00117 * The result is linearly distributed in the range [min..max], inclusive. 00118 * 00119 * \param min minimum value that can be generated 00120 * \param max maximum value that can be generated 00121 */ 00122 uint16_t randLIB_get_random_in_range(uint16_t min, uint16_t max); 00123 00124 /** 00125 * \brief Randomise a base 32-bit number by a jitter factor 00126 * 00127 * The result is linearly distributed in the jitter range, which is expressed 00128 * as fixed-point unsigned 1.15 values. For example, to produce a number in the 00129 * range [0.75 * base, 1.25 * base], set min_factor to 0x6000 and max_factor to 00130 * 0xA000. 00131 * 00132 * Result is clamped to 0xFFFFFFFF if it overflows. 00133 * 00134 * \param base The base 32-bit value 00135 * \param min_factor The minimum value for the random factor 00136 * \param max_factor The maximum value for the random factor 00137 */ 00138 uint32_t randLIB_randomise_base(uint32_t base, uint16_t min_factor, uint16_t max_factor); 00139 00140 #ifdef RANDLIB_PRNG 00141 /* \internal Reset the PRNG state to zero (invalid) */ 00142 void randLIB_reset(void); 00143 #endif 00144 00145 00146 #ifdef __cplusplus 00147 } 00148 #endif 00149 #endif /* RANDLIB_H_ */
Generated on Tue Jul 12 2022 19:12:15 by
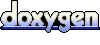