
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pv_macros.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __PV_MACROS_H__ 00018 #define __PV_MACROS_H__ 00019 00020 #include <inttypes.h> 00021 #include <stdbool.h> 00022 #include <string.h> 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /* Ignore a pointer parameter */ 00029 #define PV_IGNORE_PARAM_PTR NULL 00030 00031 /* Ignore parameter value */ 00032 #define PV_IGNORE_PARAM_VAL 0 00033 00034 /* This parameter is temporarily muted or unused for good resons */ 00035 #define PV_UNUSED_PARAM(param) \ 00036 (void)(param) 00037 00038 /* Variable used only for DEBUG targets (like prints or macros 00039 * which are effective only in debug mode) */ 00040 #define PV_DEBUG_USE(var) \ 00041 PV_UNUSED_PARAM(var) 00042 00043 00044 /* Compile time assertion (we do not have static_assert support). */ 00045 #define PV_ASSERT_CONCAT_(a, b) a##b 00046 #define PV_ASSERT_CONCAT(a, b) PV_ASSERT_CONCAT_(a, b) 00047 #define PV_CASSERT(cond, message) \ 00048 enum { PV_ASSERT_CONCAT(assert_line_, __LINE__) = 1 / (int)(!!(cond)) } 00049 00050 /* Returns the amount of elements in an array. */ 00051 #define PV_ARRAY_LENGTH(array) (sizeof(array)/sizeof((array)[0])) 00052 00053 /*Returns number in range between max and min */ 00054 #define PV_NUMBER_LIMIT(number, max, min ) ((number % (max - min + 1)) + min) 00055 00056 00057 /** 00058 * Returns the size of a member in a struct. 00059 */ 00060 #define PV_SIZEOF_MEMBER(struct_type, member_name) sizeof(((struct_type *)0)->member_name) 00061 00062 /** 00063 * Checks alignment of val to uint32_t 00064 */ 00065 #ifndef SA_PV_PLAT_PC 00066 #define PV_IS_ALIGNED(val) \ 00067 ((val & ((sizeof(uint32_t) - 1))) == 0) 00068 #else 00069 #define PV_IS_ALIGNED(val) \ 00070 ((sizeof(uint32_t) & ((sizeof(uint32_t) - 1))) == 0) 00071 #endif 00072 00073 00074 /** Reads a uint32_t from a potentially unaligned uint8_t pointer. 00075 * As we cannot know if unaligned access is allowed, using this approach is the 00076 * only way to guarantee correct behavior. 00077 * 00078 * @param buf 00079 * 00080 * @returns 00081 * 32 bit number 00082 */ 00083 static inline uint32_t pv_read_uint32(const uint8_t *buf) 00084 { 00085 uint32_t number; 00086 memcpy(&number, buf, sizeof(number)); 00087 return number; 00088 } 00089 00090 00091 00092 /** Reads a uint64_t from a potentially unaligned uint8_t pointer. 00093 * As we cannot know if unaligned access is allowed, using this approach is the 00094 * only way to guarantee correct behavior. 00095 * 00096 * @param buf 00097 * 00098 * @returns 00099 * 64 bit number 00100 */ 00101 static inline uint64_t pv_read_uint64(const uint8_t *buf) 00102 { 00103 uint64_t number; 00104 memcpy(&number, buf, sizeof(number)); 00105 return number; 00106 } 00107 00108 00109 /** Writes a uint32_t to a potentially unaligned uint8_t pointer. 00110 * As we cannot know if unaligned access is allowed, using this approach is the 00111 * only way to guarantee correct behavior. 00112 * 00113 * @param buf 00114 * @param number 00115 * 00116 */ 00117 static inline void pv_write_uint32(uint8_t *buf, uint32_t number) 00118 { 00119 memcpy(buf, &number, sizeof(number)); 00120 } 00121 00122 00123 /** Calculates the length of a string. 00124 * 00125 * @param str [in] - A pointer to an input string. If NULL, 0 will be returned. 00126 * 00127 * @returns 00128 * the number of characters in a string without counting the null termination character. 00129 * There is no strnlen in libC. It's posix extension that also exists in mbed-os, but may not exist in other OS 00130 */ 00131 static inline uint32_t pv_str_n_len(const char* str, uint32_t max_size) 00132 { 00133 uint32_t i = 0; 00134 if (str == NULL) { 00135 return 0; 00136 } 00137 00138 while (i < max_size && str[i] != '\0') { 00139 i++; 00140 } 00141 00142 return i; 00143 } 00144 00145 00146 /** Compares strings (source with target) 00147 * 00148 * @param str1 [in] - First string to compare 00149 * @param str2 [in] - Second string to compare 00150 * @param a_max_size [in] - Max number of characters to compare 00151 * 00152 * @returns 00153 * true - if strings are identical. 00154 * false -if strings are not identical 00155 */ 00156 static inline bool pv_str_equals(const char* str1, const char* str2, uint32_t a_max_size) 00157 { 00158 uint32_t str_size = pv_str_n_len(str1, a_max_size); 00159 00160 if (str_size == a_max_size) { 00161 return false; 00162 } 00163 if (str_size != pv_str_n_len(str2, a_max_size)) { 00164 return false; 00165 } 00166 00167 if (strncmp(str1, str2, a_max_size) != 0) { 00168 return false; 00169 } 00170 00171 return true; 00172 } 00173 00174 #ifdef __cplusplus 00175 } 00176 #endif 00177 00178 #endif // __PV_MACROS_H__ 00179
Generated on Tue Jul 12 2022 19:12:15 by
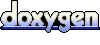