
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pv_endian.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __PV_ENDIAN_H__ 00018 #define __PV_ENDIAN_H__ 00019 00020 #include <inttypes.h> 00021 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 /** @file pv_endian.h 00028 * 00029 * Utility functions that treats endianness. 00030 */ 00031 00032 00033 /** Converts a little endian 32 bit integer to the host endianness, in a platform independent manner. 00034 * 00035 * @param le32 [in] 32 bit integer in little endian format. 00036 * 00037 * @returns 00038 * 32 bit integer in host endianness format. 00039 */ 00040 static inline uint32_t pv_le32_to_h(uint32_t le32) 00041 { 00042 const uint8_t* le32ptr = (uint8_t*)&le32; 00043 return (le32ptr[0] << 0) + 00044 (le32ptr[1] << 8) + 00045 (le32ptr[2] << 16) + 00046 (le32ptr[3] << 24); 00047 } 00048 00049 /** Converts a big endian 32 bit integer to the host endianness, in a platform independent manner. 00050 * 00051 * @param be32 [in] 32 bit integer in big endian format. 00052 * 00053 * @returns 00054 * 32 bit integer in host endianness format. 00055 */ 00056 static inline uint32_t pv_be32_to_h(uint32_t be32) 00057 { 00058 const uint8_t* be32ptr = (uint8_t*)&be32; 00059 return (be32ptr[0] << 24) + 00060 (be32ptr[1] << 16) + 00061 (be32ptr[2] << 8) + 00062 (be32ptr[3] << 0); 00063 } 00064 00065 00066 /** Converts a host endianness 32 bit integer to little endian, in a platform independent manner. 00067 * 00068 * @param host32 [in] 32 bit integer in host endianness format 00069 * 00070 * @returns 00071 * 32 bit integer in little endian format. 00072 */ 00073 static inline uint32_t pv_h_to_le32(uint32_t host32) 00074 { 00075 uint32_t le32; 00076 uint8_t *le32_ptr = (uint8_t*)&le32; 00077 00078 le32_ptr[0] = (host32 >> 0) & 0xff; 00079 le32_ptr[1] = (host32 >> 8) & 0xff; 00080 le32_ptr[2] = (host32 >> 16) & 0xff; 00081 le32_ptr[3] = (uint8_t)(host32 >> 24) & 0xff; 00082 00083 /*@-usedef@*/ 00084 // le32 is being initialized through a pointer. 00085 return le32; 00086 /*@+usedef@*/ 00087 } 00088 00089 00090 /** Converts a host endianness 32 bit integer to big endian, in a platform independent manner. 00091 * 00092 * @param host32 [in] 32 bit integer in host endianness format 00093 * 00094 * @returns 00095 * 32 bit integer in big endian format. 00096 */ 00097 static inline uint32_t pv_h_to_be32(uint32_t host32) 00098 { 00099 uint32_t be32; 00100 uint8_t *be32_ptr = (uint8_t*)&be32; 00101 00102 be32_ptr[0] = (uint8_t)(host32 >> 24) & 0xff; 00103 be32_ptr[1] = (host32 >> 16) & 0xff; 00104 be32_ptr[2] = (host32 >> 8) & 0xff; 00105 be32_ptr[3] = (host32 >> 0) & 0xff; 00106 00107 /*@-usedef@*/ 00108 // be32 is being initialized through a pointer. 00109 return be32; 00110 /*@+usedef@*/ 00111 } 00112 00113 #ifdef __cplusplus 00114 } 00115 #endif 00116 00117 #endif // __PV_ENDIAN_H__ 00118
Generated on Tue Jul 12 2022 19:12:15 by
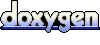