
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_startup.cpp
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #include "PlatIncludes.h" 00017 #include "pal_BSP.h" 00018 #include "mbed.h" 00019 00020 #ifndef TEST_K64F_BAUD_RATE 00021 #define TEST_K64F_BAUD_RATE 115200 00022 #endif 00023 00024 #ifndef TEST_MAIN_THREAD_STACK_SIZE 00025 #define TEST_MAIN_THREAD_STACK_SIZE (1024*7) 00026 #endif 00027 00028 extern int initSDcardAndFileSystem(bool reformat); 00029 00030 Serial pc(USBTX, USBRX); 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 00037 bool runProgram(testMain_t func, pal_args_t * args) 00038 { 00039 Thread thread(osPriorityNormal, TEST_MAIN_THREAD_STACK_SIZE); 00040 thread.start(callback(func, args)); 00041 wait(1); // to be on the safe side - sleep for 1sec 00042 bool result = (thread.join() == osOK); 00043 return result; 00044 } 00045 00046 bspStatus_t initPlatformReformat(void** outputContext) 00047 { 00048 bspStatus_t bspStatus = BSP_SUCCESS; 00049 int err = 0; 00050 00051 pc.baud(TEST_K64F_BAUD_RATE); 00052 00053 err = initSDcardAndFileSystem(true); 00054 if (err < 0) { 00055 bspStatus = BSP_GENERIC_FAILURE; 00056 printf("BSP ERROR: failed to init SD card and filesystem \r\n"); 00057 } 00058 00059 if (BSP_SUCCESS == bspStatus) 00060 { 00061 if (NULL != outputContext) 00062 { 00063 *outputContext = palTestGetNetWorkInterfaceContext(); 00064 } 00065 } 00066 00067 return bspStatus; 00068 } 00069 00070 bspStatus_t initPlatform(void** outputContext) 00071 { 00072 bspStatus_t bspStatus = BSP_SUCCESS; 00073 int err = 0; 00074 00075 pc.baud(TEST_K64F_BAUD_RATE); 00076 00077 err = initSDcardAndFileSystem(false); 00078 if (err < 0) { 00079 bspStatus = BSP_GENERIC_FAILURE; 00080 printf("BSP ERROR: failed to init SD card and filesystem \r\n"); 00081 } 00082 00083 if (BSP_SUCCESS == bspStatus) 00084 { 00085 if (NULL != outputContext) 00086 { 00087 *outputContext = palTestGetNetWorkInterfaceContext(); 00088 } 00089 } 00090 00091 return bspStatus; 00092 } 00093 00094 #ifdef __cplusplus 00095 } 00096 #endif
Generated on Tue Jul 12 2022 19:12:15 by
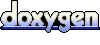