
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_update.cpp
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 #include <mbed.h> 00018 #include <pal.h> 00019 #include <pal_plat_update.h> 00020 00021 00022 #ifndef PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 00023 #ifdef MBED_CONF_MBED_CLIENT_PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 00024 #define PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET MBED_CONF_MBED_CLIENT_PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 00025 #else // MBED_CONF_MBED_CLIENT_PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 00026 #define PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 0 00027 #endif // MBED_CONF_MBED_CLIENT_PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 00028 #endif // PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET 00029 00030 palStatus_t pal_plat_imageInitAPI (palImageSignalEvent_t CBfunction) 00031 { 00032 return PAL_ERR_NOT_IMPLEMENTED ; 00033 } 00034 00035 palStatus_t pal_plat_imageDeInit (void) 00036 { 00037 return PAL_ERR_NOT_IMPLEMENTED ; 00038 } 00039 00040 palStatus_t pal_plat_imageGetMaxNumberOfImages (uint8_t *imageNumber) 00041 { 00042 return PAL_ERR_NOT_IMPLEMENTED ; 00043 } 00044 00045 palStatus_t pal_plat_imageSetVersion (palImageId_t imageId, const palConstBuffer_t* version) 00046 { 00047 return PAL_ERR_NOT_IMPLEMENTED ; 00048 } 00049 00050 palStatus_t pal_plat_imageGetDirectMemAccess (palImageId_t imageId, void** imagePtr, size_t *imageSizeInBytes) 00051 { 00052 return PAL_ERR_NOT_IMPLEMENTED ; 00053 } 00054 00055 palStatus_t pal_plat_imageActivate (palImageId_t imageId) 00056 { 00057 return PAL_ERR_NOT_IMPLEMENTED ; 00058 } 00059 00060 palStatus_t pal_plat_imageGetActiveHash (palBuffer_t *hash) 00061 { 00062 palStatus_t ret = PAL_ERR_UPDATE_ERROR ; 00063 uint32_t read_offset = PAL_UPDATE_ACTIVE_METADATA_HEADER_OFFSET + 00064 offsetof(FirmwareHeader_t, firmwareSHA256); 00065 00066 FlashIAP flash; 00067 int rc = -1; 00068 00069 rc = flash.init(); 00070 if (rc != 0) 00071 { 00072 DEBUG_PRINT("flash init failed\r\n"); 00073 goto exit; 00074 } 00075 00076 00077 rc = flash.read(hash->buffer, read_offset, SIZEOF_SHA256); 00078 if (rc != 0) 00079 { 00080 DEBUG_PRINT("flash read failed\r\n"); 00081 goto exit; 00082 } 00083 00084 hash->bufferLength = SIZEOF_SHA256; 00085 00086 rc = flash.deinit(); 00087 if (rc != 0) 00088 { 00089 DEBUG_PRINT("flash deinit failed\r\n"); 00090 goto exit; 00091 } 00092 00093 ret = PAL_SUCCESS; 00094 00095 exit: 00096 return ret; 00097 } 00098 00099 palStatus_t pal_plat_imageGetActiveVersion (palBuffer_t* version) 00100 { 00101 return PAL_ERR_NOT_IMPLEMENTED ; 00102 } 00103 00104 palStatus_t pal_plat_imageWriteHashToMemory (const palConstBuffer_t* const hashValue) 00105 { 00106 return PAL_ERR_NOT_IMPLEMENTED ; 00107 } 00108 00109 palStatus_t pal_plat_imageSetHeader (palImageId_t imageId, palImageHeaderDeails_t *details) 00110 { 00111 return PAL_ERR_NOT_IMPLEMENTED ; 00112 } 00113 00114 palStatus_t pal_plat_imageReserveSpace (palImageId_t imageId, size_t imageSize) 00115 { 00116 return PAL_ERR_NOT_IMPLEMENTED ; 00117 } 00118 00119 palStatus_t pal_plat_imageWrite (palImageId_t imageId, size_t offset, palConstBuffer_t *chunk) 00120 { 00121 return PAL_ERR_NOT_IMPLEMENTED ; 00122 } 00123 00124 palStatus_t pal_plat_imageReadToBuffer (palImageId_t imageId, size_t offset, palBuffer_t *chunk) 00125 { 00126 return PAL_ERR_NOT_IMPLEMENTED ; 00127 } 00128 00129 palStatus_t pal_plat_imageFlush (palImageId_t package_id) 00130 { 00131 return PAL_ERR_NOT_IMPLEMENTED ; 00132 }
Generated on Tue Jul 12 2022 19:12:14 by
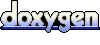