
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_rtos.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 00018 #ifndef _PAL_PLAT_RTOS_H 00019 #define _PAL_PLAT_RTOS_H 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #include "pal.h" 00026 00027 00028 /*! \file pal_plat_rtos.h 00029 * \brief PAL RTOS - platform. 00030 * This file contains the real-time OS APIs that need to be implemented in the platform layer. 00031 */ 00032 00033 #define PAL_SHA256_DEVICE_KEY_SIZE_IN_BYTES 32 00034 #define PAL_DEVICE_KEY_SIZE_IN_BITS (128) 00035 #define PAL_DEVICE_KEY_SIZE_IN_BYTES (PAL_DEVICE_KEY_SIZE_IN_BITS / 8) 00036 00037 00038 /*! Initiate a system reboot. 00039 */ 00040 void pal_plat_osReboot (void); 00041 00042 /*! Application provided implementation to replace default pal_osReboot() functionality. 00043 */ 00044 void pal_plat_osApplicationReboot (void); 00045 00046 /*! Initialize all data structures (semaphores, mutexes, memory pools, message queues) at system initialization. 00047 * In case of a failure in any of the initializations, the function returns an error and stops the rest of the initializations. 00048 * @param[in] opaqueContext The context passed to the initialization (not required for generic CMSIS, pass NULL in this case). 00049 * \return PAL_SUCCESS(0) in case of success, PAL_ERR_CREATION_FAILED in case of failure. 00050 */ 00051 palStatus_t pal_plat_RTOSInitialize (void* opaqueContext); 00052 00053 /*! De-initialize thread objects. 00054 */ 00055 palStatus_t pal_plat_RTOSDestroy (void); 00056 00057 /*! Get the RTOS kernel system timer counter. 00058 * 00059 * \return The RTOS kernel system timer counter. 00060 * 00061 * \note The required tick counter is the OS (platform) kernel system tick counter. 00062 * \note If the platform supports 64-bit tick counter, please implement it. If the platform supports only 32 bit, note 00063 * that this counter wraps around very often (for example, once every 42 sec for 100Mhz). 00064 */ 00065 uint64_t pal_plat_osKernelSysTick (void); 00066 00067 /*! Convert the value from microseconds to kernel sys ticks. 00068 * This is the same as CMSIS macro `osKernelSysTickMicroSec`. 00069 */ 00070 uint64_t pal_plat_osKernelSysTickMicroSec (uint64_t microseconds); 00071 00072 /*! Get the system tick frequency. 00073 * \return The system tick frequency. 00074 * 00075 * \note The system tick frequency MUST be more than 1KHz (at least one tick per millisecond). 00076 */ 00077 uint64_t pal_plat_osKernelSysTickFrequency (void); 00078 00079 /*! Create and run the thread. 00080 * 00081 * @param[in] function A function pointer to the thread callback function. 00082 * @param[in] funcArgument An argument for the thread function. 00083 * @param[in] priority The priority of the thread. 00084 * @param[in] stackSize The stack size of the thread, can NOT be 0. 00085 * @param[out] threadID: The created thread ID handle. In case of error, this value is NULL. 00086 * 00087 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00088 * 00089 */ 00090 palStatus_t pal_plat_osThreadCreate (palThreadFuncPtr function, void* funcArgument, palThreadPriority_t priority, uint32_t stackSize, palThreadID_t* threadID); 00091 00092 /*! Terminate and free allocated data for the thread. 00093 * 00094 * @param[in] threadData A pointer to a palThreadData_t structure containing information about the thread. 00095 * 00096 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00097 * 00098 */ 00099 palStatus_t pal_plat_osThreadTerminate (palThreadID_t* threadID); 00100 00101 /*! Get the ID of the current thread. 00102 * \return The ID of the current thread. In case of error, returns PAL_MAX_UINT32. 00103 */ 00104 palThreadID_t pal_plat_osThreadGetId (void); 00105 00106 /*! Wait for a specified period of time in milliseconds. 00107 * 00108 * @param[in] milliseconds The number of milliseconds to wait before proceeding. 00109 * 00110 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00111 */ 00112 palStatus_t pal_plat_osDelay (uint32_t milliseconds); 00113 00114 /*! Create a timer. 00115 * 00116 * @param[in] function A function pointer to the timer callback function. 00117 * @param[in] funcArgument An argument for the timer callback function. 00118 * @param[in] timerType The timer type to be created, periodic or `oneShot`. 00119 * @param[out] timerID The ID of the created timer. Zero value indicates an error. 00120 * 00121 * \return PAL_SUCCESS when the timer was created successfully. A specific error in case of failure. \n 00122 * PAL_ERR_NO_MEMORY: No memory resource available to create a timer object. 00123 * 00124 * \note The timer callback function runs according to the platform resources of stack size and priority. 00125 * \note The create function MUST not wait for platform resources and it should return PAL_ERR_RTOS_RESOURCE, unless the platform API is blocking. 00126 */ 00127 palStatus_t pal_plat_osTimerCreate (palTimerFuncPtr function, void* funcArgument, palTimerType_t timerType, palTimerID_t* timerID); 00128 00129 /*! Start or restart a timer. 00130 * 00131 * @param[in] timerID The handle for the timer to start. 00132 * @param[in] millisec: The time in milliseconds to set the timer to, MUST be larger than 0. 00133 * In case the value is 0, the error PAL_ERR_RTOS_VALUE will be returned. 00134 * 00135 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00136 */ 00137 palStatus_t pal_plat_osTimerStart (palTimerID_t timerID, uint32_t millisec); 00138 00139 /*! Stop a timer. 00140 * 00141 * @param[in] timerID The handle for the timer to stop. 00142 * 00143 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00144 */ 00145 palStatus_t pal_plat_osTimerStop (palTimerID_t timerID); 00146 00147 /*! Delete the timer object. 00148 * 00149 * @param[inout] timerID The handle for the timer to delete. In success, `*timerID` = NULL. 00150 * 00151 * \return PAL_SUCCESS when the timer was deleted successfully. PAL_ERR_RTOS_PARAMETER when the `timerID` is incorrect. 00152 * \note In case of a running timer, `pal_platosTimerDelete()` MUST stop the timer before deletion. 00153 */ 00154 palStatus_t pal_plat_osTimerDelete (palTimerID_t* timerID); 00155 00156 /*! Create and initialize a mutex object. 00157 * 00158 * @param[out] mutexID The created mutex ID handle, zero value indicates an error. 00159 * 00160 * \return PAL_SUCCESS when the mutex was created successfully, a specific error in case of failure. \n 00161 * PAL_ERR_NO_MEMORY when there is no memory resource available to create a mutex object. 00162 * \note The create function MUST NOT wait for the platform resources and it should return PAL_ERR_RTOS_RESOURCE, unless the platform API is blocking. 00163 * By default, the mutex is created with a recursive flag set. 00164 */ 00165 palStatus_t pal_plat_osMutexCreate (palMutexID_t* mutexID); 00166 00167 /*! Wait until a mutex becomes available. 00168 * 00169 * @param[in] mutexID The handle for the mutex. 00170 * @param[in] millisec The timeout for the waiting operation if the timeout expires before the semaphore is released and an error is returned from the function. 00171 * 00172 * \return PAL_SUCCESS(0) in case of success. One of the following error codes in case of failure: \n 00173 * - PAL_ERR_RTOS_RESOURCE - Mutex not available but no timeout set. \n 00174 * - PAL_ERR_RTOS_TIMEOUT - Mutex was not available until timeout expired. \n 00175 * - PAL_ERR_RTOS_PARAMETER - The mutex ID is invalid. \n 00176 * - PAL_ERR_RTOS_ISR - Cannot be called from interrupt service routines. 00177 */ 00178 palStatus_t pal_plat_osMutexWait (palMutexID_t mutexID, uint32_t millisec); 00179 00180 /*! Release a mutex that was obtained by `osMutexWait`. 00181 * 00182 * @param[in] mutexID The handle for the mutex. 00183 * 00184 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00185 */ 00186 palStatus_t pal_plat_osMutexRelease (palMutexID_t mutexID); 00187 00188 /*! Delete a mutex object. 00189 * 00190 * @param[inout] mutexID The ID of the mutex to delete. In success, `*mutexID` = NULL. 00191 * 00192 * \return PAL_SUCCESS when the mutex was deleted successfully, one of the following error codes in case of failure: \n 00193 * - PAL_ERR_RTOS_RESOURCE - Mutex already released. \n 00194 * - PAL_ERR_RTOS_PARAMETER - Mutex ID is invalid. \n 00195 * - PAL_ERR_RTOS_ISR - Cannot be called from interrupt service routines. 00196 * \note After this call, `mutex_id` is no longer valid and cannot be used. 00197 */ 00198 palStatus_t pal_plat_osMutexDelete (palMutexID_t* mutexID); 00199 00200 /*! Create and initialize a semaphore object. 00201 * 00202 * @param[in] count The number of available resources. 00203 * @param[out] semaphoreID The ID of the created semaphore, zero value indicates an error. 00204 * 00205 * \return PAL_SUCCESS when the semaphore was created successfully, a specific error in case of failure. \n 00206 * PAL_ERR_NO_MEMORY: No memory resource available to create a semaphore object. 00207 * \note The create function MUST not wait for the platform resources and it should return PAL_ERR_RTOS_RESOURCE, unless the platform API is blocking. 00208 */ 00209 palStatus_t pal_plat_osSemaphoreCreate (uint32_t count, palSemaphoreID_t* semaphoreID); 00210 00211 /*! Wait until a semaphore token becomes available. 00212 * 00213 * @param[in] semaphoreID The handle for the semaphore. 00214 * @param[in] millisec The timeout for the waiting operation if the timeout expires before the semaphore is released and an error is returned from the function. 00215 * @param[out] countersAvailable The number of semaphores available. If semaphores are not available (timeout/error) zero is returned. 00216 * \return PAL_SUCCESS(0) in case of success. One of the following error codes in case of failure: \n 00217 * - PAL_ERR_RTOS_TIMEOUT - Semaphore was not available until timeout expired. \n 00218 * - PAL_ERR_RTOS_PARAMETER - Semaphore ID is invalid. 00219 */ 00220 palStatus_t pal_plat_osSemaphoreWait (palSemaphoreID_t semaphoreID, uint32_t millisec, int32_t* countersAvailable); 00221 00222 /*! Release a semaphore token. 00223 * 00224 * @param[in] semaphoreID The handle for the semaphore. 00225 * 00226 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00227 */ 00228 palStatus_t pal_plat_osSemaphoreRelease (palSemaphoreID_t semaphoreID); 00229 00230 /*! Delete a semaphore object. 00231 * 00232 * @param[inout] semaphoreID: The ID of the semaphore to delete. In success, `*semaphoreID` = NULL. 00233 * 00234 * \return PAL_SUCCESS when the semaphore was deleted successfully. One of the following error codes in case of failure: \n 00235 * PAL_ERR_RTOS_RESOURCE - Semaphore already released. \n 00236 * PAL_ERR_RTOS_PARAMETER - Semaphore ID is invalid. 00237 * \note After this call, the `semaphore_id` is no longer valid and cannot be used. 00238 */ 00239 palStatus_t pal_plat_osSemaphoreDelete (palSemaphoreID_t* semaphoreID); 00240 00241 00242 /*! Perform an atomic increment for a signed32 bit value. 00243 * 00244 * @param[in,out] valuePtr The address of the value to increment. 00245 * @param[in] increment The number by which to increment. 00246 * 00247 * \returns The value of the `valuePtr` after the increment operation. 00248 */ 00249 int32_t pal_plat_osAtomicIncrement (int32_t* valuePtr, int32_t increment); 00250 00251 /*! Perform allocation from the heap according to the OS specification. 00252 * 00253 * @param[in] len The length of the buffer to be allocated. 00254 * 00255 * \returns `void *`. The pointer of the malloc received from the OS if NULL error occurred 00256 */ 00257 void *pal_plat_malloc (size_t len); 00258 00259 /*! Free memory back to the OS heap. 00260 * 00261 * @param[in] *buffer A pointer to the buffer that should be free. 00262 * 00263 * \returns `void` 00264 */ 00265 void pal_plat_free (void * buffer); 00266 00267 /*! Generate a random number into the given buffer with the given size in bytes. 00268 * 00269 * @param[out] randomBuf A buffer to hold the generated number. 00270 * @param[in] bufSizeBytes The size of the buffer and the size of the required random number to generate. 00271 * @param[out] actualRandomSizeBytes The actual size of the written random data to the output buffer. 00272 \return PAL_SUCCESS on success. A negative value indicating a specific error code in case of failure. 00273 \note In case the platform was able to provide random data with non-zero size and less than `bufSizeBytes`the function must return `PAL_ERR_RTOS_TRNG_PARTIAL_DATA` 00274 */ 00275 palStatus_t pal_plat_osRandomBuffer (uint8_t *randomBuf, size_t bufSizeBytes, size_t* actualRandomSizeBytes); 00276 00277 00278 /*! Retrieve platform Root of Trust certificate 00279 * 00280 * @param[in,out] *keyBuf A pointer to the buffer that holds the RoT. 00281 * @param[in] keyLenBytes The size of the buffer to hold the 128 bit key, must be at least 16 bytes. 00282 * The buffer needs to be able to hold 16 bytes of data. 00283 * 00284 * \return PAL_SUCCESS(0) in case of success. A negative value indicating a specific error code in case of failure. 00285 */ 00286 00287 palStatus_t pal_plat_osGetRoTFromHW (uint8_t *keyBuf, size_t keyLenBytes); 00288 00289 /*! \brief This function calls the platform layer and sets the new RTC to the H/W 00290 * 00291 * @param[in] uint64_t rtcSetTime the new RTC time 00292 * 00293 * \return PAL_SUCCESS when the RTC return correctly 00294 * 00295 */ 00296 palStatus_t pal_plat_osSetRtcTime(uint64_t rtcSetTime); 00297 00298 /*! \brief This function gets the RTC from the platform 00299 * 00300 * @param[out] uint64_t * rtcGetTime - Holds the RTC value 00301 * 00302 * \return PAL_SUCCESS when the RTC return correctly 00303 * 00304 */ 00305 palStatus_t pal_plat_osGetRtcTime(uint64_t *rtcGetTime); 00306 00307 00308 /*! \brief This function DeInitialize the RTC module 00309 * 00310 * \return PAL_SUCCESS when the success or error upon failing 00311 * 00312 */ 00313 palStatus_t pal_plat_rtcDeInit(void); 00314 00315 00316 /*! \brief This function initialize the RTC module 00317 * 00318 * \return PAL_SUCCESS when the success or error upon failing 00319 * 00320 */ 00321 palStatus_t pal_plat_rtcInit(void); 00322 00323 #ifdef __cplusplus 00324 } 00325 #endif 00326 #endif //_PAL_COMMON_H
Generated on Tue Jul 12 2022 19:12:14 by
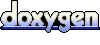