
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_internalFlash.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #ifndef PAL_PLAT_FLASH_H_ 00017 #define PAL_PLAT_FLASH_H_ 00018 00019 #include "pal_internalFlash.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 /*! \brief This function initialized the flash API module, 00026 * And should be called prior flash APIs calls 00027 * 00028 * \return PAL_SUCCESS upon successful operation. \n 00029 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00030 * 00031 * \note should be called only once unless \c pal_InternalFlashDeinit function is called 00032 * \note This function is Blocking till completion!! 00033 * 00034 */ 00035 palStatus_t pal_plat_internalFlashInit(void); 00036 00037 /*! \brief This function destroy the flash module 00038 * 00039 * \return PAL_SUCCESS upon successful operation. \n 00040 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00041 * 00042 * \note Should be called only after \c pal_InternalFlashinit() is called. 00043 * \note Flash APIs will not work after calling this function 00044 * \note This function is Blocking till completion!! 00045 * 00046 */ 00047 palStatus_t pal_plat_internalFlashDeInit(void); 00048 00049 /*! \brief This function writes to the internal flash 00050 * 00051 * @param[in] buffer - pointer to the buffer to be written 00052 * @param[in] size - the size of the buffer in bytes, must be aligned to minimum writing unit (page size). 00053 * @param[in] address - the address of the internal flash. 00054 * 00055 * \return PAL_SUCCESS upon successful operation. \n 00056 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00057 * 00058 * \note This function is Blocking till completion!! 00059 * \note This function is Thread Safe!! 00060 */ 00061 palStatus_t pal_plat_internalFlashWrite(const size_t size, const uint32_t address, const uint32_t * buffer); 00062 00063 /*! \brief This function copies the memory data into the user given buffer 00064 * 00065 * @param[in] size - the size of the buffer in bytes. 00066 * @param[in] address - the address of the internal flash. 00067 * @param[out] buffer - pointer to the buffer to write to 00068 * 00069 * \return PAL_SUCCESS upon successful operation. \n 00070 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00071 * \note This function is Blocking till completion!! 00072 * \note This function is Thread Safe!! 00073 * 00074 */ 00075 palStatus_t pal_plat_internalFlashRead(const size_t size, const uint32_t address, uint32_t * buffer); 00076 00077 /*! \brief This function Erase the sector 00078 * 00079 * @param[in] size - the size to be erased, must be align to sector. 00080 * @param[in] address - sector start address to be erased, must be align to sector. 00081 * 00082 * \return PAL_SUCCESS upon successful operation. \n 00083 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00084 * 00085 * \note ALL sectors can be erased!! No protection to bootloader, program or other... 00086 * \note This function is Blocking till completion!! 00087 * \note Only one sector can be erased in each function call 00088 * \note This function is Thread Safe!! 00089 */ 00090 palStatus_t pal_plat_internalFlashErase(uint32_t address, size_t size); 00091 00092 /*! \brief This function returns the minimum writing unit to the flash 00093 * 00094 * \return size_t the 2, 4, 8.... 00095 */ 00096 size_t pal_plat_internalFlashGetPageSize(void); 00097 00098 00099 /*! \brief This function returns the sector size 00100 * 00101 * @param[in] address - the starting address of the sector is question 00102 * 00103 * \return size of sector, 0 if error 00104 */ 00105 size_t pal_plat_internalFlashGetSectorSize(uint32_t address); 00106 00107 00108 00109 /////////////////////////////////////////////////////////////// 00110 ////-------------------SOTP functions------------------------// 00111 /////////////////////////////////////////////////////////////// 00112 /*! \brief This function return the SOTP section data 00113 * 00114 * @param[in] section - the section number (0 or 1) 00115 * @param[out] data - the information about the section 00116 * 00117 * \return PAL_SUCCESS upon successful operation. \n 00118 * PAL_ERR_INTERNAL_FLASH_ERROR - see error code \c palError_t. 00119 * 00120 */ 00121 palStatus_t pal_plat_internalFlashGetAreaInfo(uint8_t section, palSotpAreaData_t *data); 00122 00123 00124 #ifdef __cplusplus 00125 } 00126 #endif 00127 00128 #endif /* PAL_PLAT_FLASH_H_ */
Generated on Tue Jul 12 2022 19:12:14 by
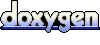