
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_internalFlash.c
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 #include "pal.h" 00018 #include "pal_plat_internalFlash.h" 00019 #include "fsl_flash.h" 00020 00021 ////////////////////////////PRIVATE/////////////////////////////////// 00022 PAL_PRIVATE flash_config_t g_flashDescriptor = {0}; 00023 ////////////////////////////END PRIVATE//////////////////////////////// 00024 00025 palStatus_t pal_plat_internalFlashInit(void) 00026 { 00027 status_t status; 00028 palStatus_t ret = PAL_SUCCESS; 00029 status = FLASH_Init(&g_flashDescriptor); 00030 if(kStatus_FLASH_Success != status) 00031 { 00032 ret = PAL_ERR_INTERNAL_FLASH_INIT_ERROR; 00033 } 00034 return ret; 00035 } 00036 00037 00038 palStatus_t pal_plat_internalFlashDeInit(void) 00039 { 00040 memset(&g_flashDescriptor, 0, sizeof(g_flashDescriptor)); 00041 return PAL_SUCCESS; 00042 } 00043 00044 00045 palStatus_t pal_plat_internalFlashWrite(const size_t size, const uint32_t address, const uint32_t * buffer) 00046 { 00047 palStatus_t ret = PAL_SUCCESS; 00048 status_t status = kStatus_Success; 00049 00050 /* We need to prevent flash accesses during program operation */ 00051 __disable_irq(); 00052 status = FLASH_Program(&g_flashDescriptor, address, (uint32_t *)buffer, size); 00053 if (kStatus_Success == status) 00054 { 00055 // Must use kFlashMargin_User, or kFlashMargin_Factory for verify program 00056 status = FLASH_VerifyProgram(&g_flashDescriptor, address, size, (uint32_t *)buffer, kFLASH_marginValueUser, NULL, NULL); 00057 if(kStatus_Success != status) 00058 { 00059 ret = PAL_ERR_INTERNAL_FLASH_WRITE_ERROR; 00060 } 00061 } 00062 __enable_irq(); 00063 00064 return ret; 00065 } 00066 00067 palStatus_t pal_plat_internalFlashRead(const size_t size, const uint32_t address, uint32_t * buffer) 00068 { 00069 memcpy(buffer, (const void *)address, size); 00070 return PAL_SUCCESS; 00071 } 00072 00073 00074 palStatus_t pal_plat_internalFlashErase(uint32_t address, size_t size) 00075 { 00076 palStatus_t ret = PAL_SUCCESS; 00077 int16_t status = kStatus_Success; 00078 00079 __disable_irq(); 00080 status = FLASH_Erase(&g_flashDescriptor, address, pal_plat_internalFlashGetSectorSize(address), kFLASH_apiEraseKey); 00081 if (kStatus_Success == status) 00082 { 00083 status = FLASH_VerifyErase(&g_flashDescriptor, address, pal_plat_internalFlashGetSectorSize(address), kFLASH_marginValueNormal); 00084 } 00085 00086 if (kStatus_Success != status) 00087 { 00088 ret = PAL_ERR_INTERNAL_FLASH_ERASE_ERROR; 00089 } 00090 __enable_irq(); 00091 return ret; 00092 } 00093 00094 00095 size_t pal_plat_internalFlashGetPageSize(void) 00096 { 00097 return FSL_FEATURE_FLASH_PFLASH_BLOCK_WRITE_UNIT_SIZE; 00098 } 00099 00100 00101 size_t pal_plat_internalFlashGetSectorSize(uint32_t address) 00102 { 00103 size_t devicesize = 0; 00104 FLASH_GetProperty(&g_flashDescriptor, kFLASH_propertyPflashSectorSize, (uint32_t*)&devicesize); 00105 return devicesize; 00106 } 00107
Generated on Tue Jul 12 2022 19:12:14 by
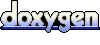