
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_plat_fileSystem.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 #ifndef PAL_PALT_FILE_SYSTEM_H 00017 #define PAL_PALT_FILE_SYSTEM_H 00018 00019 #include "pal_fileSystem.h" 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 /*! \file pal_plat_fileSystem.h 00026 * \brief PAL file system - platform. 00027 * This file contains the file system APIs that need to be implemented in the platform layer. 00028 */ 00029 00030 /*! @defgroup PAL_PLAT_GROUP_FS 00031 * \note You need to add the prefix of the ESFS folder root stored in \c g_esfsRootFolder to all files and folders. 00032 * To change this, call \c pal_plat_fsSetEsfsRootFolder(). 00033 * 00034 */ 00035 00036 /** 00037 @defgroup PAL_PLAT_PUBLIC_FUNCTION PAL Platform Public Functions 00038 @ingroup PAL_PLAT_GROUP_FS 00039 */ 00040 00041 /** 00042 @addtogroup PAL_PLAT_PUBLIC_FUNCTION 00043 @{*/ 00044 00045 /*! \brief This function attempts to create a directory named \c pathName. 00046 * 00047 * @param[in] *pathName A pointer to the null-terminated string that specifies the directory name to create. 00048 * 00049 * \return PAL_SUCCESS upon a successful operation.\n 00050 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00051 * 00052 * \note To remove a directory use \c PAL_ERR_FS_rmdir. 00053 * 00054 *\b Example 00055 \code{.cpp} 00056 palStatus_t ret; 00057 ret = PAL_ERR_FS_mkdir("Dir1"); 00058 if(!ret) 00059 { 00060 //Error 00061 } 00062 \endcode 00063 */ 00064 palStatus_t pal_plat_fsMkdir(const char *pathName); 00065 00066 00067 00068 /*! \brief This function deletes a directory. 00069 * 00070 * @param[in] *pathName A pointer to the null-terminated string that specifies the name of the directory to be deleted. 00071 * 00072 * \return PAL_SUCCESS upon a successful operation.\n 00073 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00074 * 00075 * \note The directory to be deleted \b must \b be \b Empty and \b closed. 00076 * The folder path must end with "/". 00077 * If given "..", the function changes the root directory to one directory down and deletes the working directory. 00078 */ 00079 palStatus_t pal_plat_fsRmdir(const char *pathName); 00080 00081 00082 00083 /*!\brief This function opens the file whose name is specified in the parameter `pathName` and associates it with a stream 00084 * that can be identified in future operations by the `fd` pointer returned. 00085 * 00086 * @param[out] fd A file descriptor for the file entered in the `pathName`. 00087 * @param[in] *pathName A pointer to the null-terminated string that specifies the file name to open or create. 00088 * @param[in] mode A mode flag that specifies the type of access and open method for the file. 00089 * 00090 * 00091 * \return PAL_SUCCESS upon a successful operation.\n 00092 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00093 * 00094 * \note The folder path must end with "/". 00095 * \note If necessary, the platform layer \b allocates \b memory for the file descriptor. The structure 00096 * \c pal_plat_fclose() shall free that buffer. 00097 * \note The mode flags sent to this function are normalized to the \c pal_fsFileMode_t and each platform needs to replace them with the proper values. 00098 * 00099 */ 00100 palStatus_t pal_plat_fsFopen(const char *pathName, pal_fsFileMode_t mode, palFileDescriptor_t *fd); 00101 00102 00103 00104 /*! \brief This function closes an open file object. 00105 * 00106 * @param[in] fd A pointer to the open file object structure to be closed. 00107 * 00108 * \return PAL_SUCCESS upon a successful operation. \n 00109 * PAL_FILE_SYSTEM_ERROR - see the error code \c palError_t.a 00110 * 00111 * \note After successful execution of the function, the file object is no longer valid and it can be discarded. 00112 * \note In some platforms, this function needs to \b free the file descriptor memory. 00113 */ 00114 palStatus_t pal_plat_fsFclose(palFileDescriptor_t *fd); 00115 00116 00117 00118 /*! \brief This function reads an array of bytes from the stream and stores them in the block of memory 00119 * specified by the buffer. The position indicator of the stream is advanced by the total amount of bytes read. 00120 * 00121 * @param[in] fd A pointer to the open file object structure. 00122 * @param[in] buffer A buffer for storing the read data. 00123 * @param[in] numOfBytes The number of bytes to read. 00124 * @param[out] numberOfBytesRead The number of bytes read. 00125 * 00126 * \return PAL_SUCCESS upon a successful operation.\n 00127 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00128 * 00129 * \note After successful execution of the function, 00130 * `numberOfBytesRead` should be checked to detect end of the file. 00131 * If `numberOfBytesRead` is less than `numOfBytes`, 00132 * the read/write pointer has reached the end of the file during the read operation or an error has occurred. 00133 * 00134 */ 00135 palStatus_t pal_plat_fsFread(palFileDescriptor_t *fd, void * buffer, size_t numOfBytes, size_t *numberOfBytesRead); 00136 00137 00138 00139 /*! \brief This function starts to write data from the \c buffer to the file at the position pointed by the read/write pointer. 00140 * 00141 * @param[in] fd A pointer to the open file object structure. 00142 * @param[in] buffer A pointer to the data to be written. 00143 * @param[in] numOfBytes The number of bytes to write. 00144 * @param[out] numberOfBytesWritten The number of bytes written. 00145 * 00146 * \return PAL_SUCCESS upon a successful operation. \n 00147 * PAL_FILE_SYSTEM_ERROR - see the error code \c palError_t. 00148 * 00149 * \note The read/write pointer advances as number of bytes written. After successful execution of the function, 00150 * \note `numberOfBytesWritten` should be checked to detect if the disk is full. 00151 * If `numberOfBytesWritten` is less than `numOfBytes`, the volume got full during the write operation. 00152 * 00153 */ 00154 palStatus_t pal_plat_fsFwrite(palFileDescriptor_t *fd, const void *buffer, size_t numOfBytes, size_t *numberOfBytesWritten); 00155 00156 00157 /*! \brief This function moves the file read/write pointer without any read/write operation to the file. 00158 * 00159 * @param[in] fd A pointer to the open file object structure. 00160 * @param[in] offset The byte offset from top of the file to set the read/write pointer. 00161 * @param[out] whence The offset is relative to this. 00162 * 00163 * \return PAL_SUCCESS upon a successful operation.\n 00164 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00165 * 00166 * \note - The `whence` options are: 00167 * -# \c PAL_ERR_FS_SEEKSET - relative to the start of the file. 00168 * -# \c PAL_ERR_FS_SEEKCUR - relative to the current position indicator. 00169 * -# \c PAL_ERR_FS_SEEKEND - relative to the end-of-file. 00170 * 00171 * \note In some systems, there is no \c whence argument. 00172 * If you need to implement the `whence` argument:\n 00173 * \b PAL_ERR_FS_SEEKEND - The function first finds the length of the file, then subtracts the file length from the position to find the relative path from the beginning.\n 00174 * \b PAL_ERR_FS_SEEKCUR - The function finds the current stream position and calculates the relative path from the file start.\n 00175 * 00176 * In both options, \c fseek() needs to verify that the offset is smaller than the file end or start. 00177 * 00178 */ 00179 palStatus_t pal_plat_fsFseek(palFileDescriptor_t *fd, int32_t offset, pal_fsOffset_t whence); 00180 00181 00182 00183 /*! \brief This function gets the current read/write pointer of a file. 00184 * 00185 * @param[in] fd A pointer to the open file object structure. 00186 * 00187 * \return PAL_SUCCESS upon a successful operation.\n 00188 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00189 * 00190 */ 00191 palStatus_t pal_plat_fsFtell(palFileDescriptor_t *fd, int32_t * pos); 00192 00193 00194 00195 /*! \brief This function deletes a \b single file from the file system. 00196 * 00197 * @param[in] pathName A pointer to a null-terminated string that specifies the \b file to be removed. 00198 * @param[in] buildRelativeDirectory Needed to add a working directory to give \c pathName. 00199 * 00200 * \return PAL_SUCCESS upon a successful operation.\n 00201 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00202 * 00203 * \note The file \b must \b not \b be \b opened 00204 * 00205 */ 00206 palStatus_t pal_plat_fsUnlink(const char *pathName); 00207 00208 00209 00210 /*! \brief This function deletes \b all files in a folder from the file system (FLAT remove only). 00211 * 00212 * @param[in] pathName A pointer to a null-terminated string that specifies the \b folder. 00213 * 00214 * \return PAL_SUCCESS upon a successful operation.\n 00215 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00216 * 00217 * \note The folder \b must \b not \b be \b open and the folder path must end with "/". 00218 * \note The process deletes one file at a time by calling \c pal_plat_fsUnlink until all files are removed. 00219 * \note The function does not remove the directory found in the path. 00220 */ 00221 palStatus_t pal_plat_fsRmFiles(const char *pathName); 00222 00223 00224 00225 /*! \brief This function copies \b all files from a source folder to a destination folder (FLAT copy only). 00226 * 00227 * @param[in] pathNameSrc A pointer to a null-terminated string that specifies the source folder. 00228 * @param[in] pathNameDest A pointer to a null-terminated string that specifies the destination folder (MUST exist). 00229 * 00230 * \return PAL_SUCCESS upon a successful operation.\n 00231 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00232 * 00233 * \note Both folders \b must \b not \b be \b open. If a folder does not exist the function fails. 00234 * \note The process copies one file at a time until all files are copied. 00235 * \note The function does not copy a directory found in the path. 00236 */ 00237 palStatus_t pal_plat_fsCpFolder(const char *pathNameSrc, char *pathNameDest); 00238 00239 00240 /*! \brief This function gets the default value for root directory (primary) 00241 * 00242 * @param[in] dataID - id of the data to ge the root folder for. 00243 * 00244 * \return pointer to the default path. 00245 * 00246 */ 00247 const char* pal_plat_fsGetDefaultRootFolder(pal_fsStorageID_t dataID); 00248 00249 00250 00251 /*! \brief This function finds the length of the string received. 00252 * 00253 * 00254 * @param[in] stringToChk A pointer to the string received with a null terminator. 00255 * 00256 * \return The size of the string. 00257 * 00258 */ 00259 size_t pal_plat_fsSizeCheck(const char *stringToChk); 00260 00261 00262 00263 /*! \brief This function sets up the mount point. 00264 * 00265 * 00266 * @param void 00267 * 00268 * \return PAL_SUCCESS upon a successful operation.\n 00269 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00270 * 00271 */ 00272 palStatus_t pal_plat_fsMountSystem(void); 00273 /** 00274 @} */ 00275 00276 00277 /*! \brief This function formats the SD partition indicated by `partitionID` (mapping the ID to an actual partition is done in the porting layer). 00278 * 00279 * 00280 * @param[in] partitionID The ID of the partition to be formatted. 00281 * 00282 * \return PAL_SUCCESS upon a successful operation.\n 00283 * PAL_FILE_SYSTEM_ERROR - see the error code description \c palError_t. 00284 * 00285 */ 00286 palStatus_t pal_plat_fsFormat(pal_fsStorageID_t dataID); 00287 00288 00289 #ifdef __cplusplus 00290 } 00291 #endif 00292 #endif
Generated on Tue Jul 12 2022 19:12:14 by
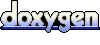