
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
pal_configuration.h
00001 /******************************************************************************* 00002 * Copyright 2016, 2017 ARM Ltd. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 *******************************************************************************/ 00016 00017 00018 #ifndef _PAL_COFIGURATION_H 00019 #define _PAL_COFIGURATION_H 00020 #include "limits.h" 00021 00022 00023 #ifdef PAL_USER_DEFINED_CONFIGURATION 00024 #include PAL_USER_DEFINED_CONFIGURATION 00025 #else 00026 #include "sotp_fs.h" 00027 #endif 00028 00029 00030 /*! \brief If needed any board specific configuration please set this define 00031 */ 00032 #ifdef PAL_BOARD_SPECIFIC_CONFIG 00033 #include PAL_BOARD_SPECIFIC_CONFIG 00034 #endif 00035 00036 00037 /*! \brief let the user choose its platform configuration file. 00038 \note if the user does not specify a platform configuration file, 00039 \note PAL uses a default configuration set that can be found at \b Configs/pal_config folder 00040 */ 00041 00042 #ifdef PAL_PLATFORM_DEFINED_CONFIGURATION 00043 #include PAL_PLATFORM_DEFINED_CONFIGURATION 00044 #elif defined(__LINUX__) 00045 #include "Linux_default.h" 00046 #elif defined(__FREERTOS__) 00047 #include "FreeRTOS_default.h" 00048 #elif defined(__MBED__) 00049 #include "mbedOS_default.h" 00050 #else 00051 #error "Please specify the platform PAL_PLATFORM_DEFINED_CONFIGURATION" 00052 #endif 00053 00054 /*! \file pal_configuration.h 00055 * \brief PAL Configuration. 00056 * This file contains PAL configuration information including the following: 00057 * 1. The flags to enable or disable features. 00058 * 2. The configuration of the number of objects provided by PAL (such as the number of threads supported) or their sizes. 00059 * 3. The configuration of supported cipher suites. 00060 * 4. The configuration for flash memory usage. 00061 * 5. The configuration for the root of trust. 00062 */ 00063 00064 00065 /* 00066 * Network configuration 00067 */ 00068 //! PAL configuration options 00069 #ifndef PAL_NET_TCP_AND_TLS_SUPPORT 00070 #define PAL_NET_TCP_AND_TLS_SUPPORT true/* Add PAL support for TCP. */ 00071 #endif 00072 00073 #ifndef PAL_NET_ASYNCHRONOUS_SOCKET_API 00074 #define PAL_NET_ASYNCHRONOUS_SOCKET_API true/* Add PAL support for asynchronous sockets. */ 00075 #endif 00076 00077 #ifndef PAL_NET_DNS_SUPPORT 00078 #define PAL_NET_DNS_SUPPORT true/* Add PAL support for DNS lookup. */ 00079 #endif 00080 00081 #if (PAL_NET_DNS_SUPPORT == true) && !(defined(PAL_DNS_API_VERSION)) 00082 #define PAL_DNS_API_VERSION 1 00083 #endif 00084 00085 #if (PAL_DNS_API_VERSION == 2) 00086 #define PAL_DNS_API_V2 00087 #endif 00088 00089 #ifndef PAL_SUPPORT_IP_V4 00090 #define PAL_SUPPORT_IP_V4 true /* support IPV4 as default*/ 00091 #endif 00092 #ifndef PAL_SUPPORT_IP_V6 00093 #define PAL_SUPPORT_IP_V6 true /* support IPV6 as default*/ 00094 #endif 00095 00096 //values for PAL_NET_DNS_IP_SUPPORT 00097 #define PAL_NET_DNS_ANY 0 /* if PAL_NET_DNS_IP_SUPPORT is set to PAL_NET_DNS_ANY pal_getAddressInfo will return the first available IPV4 or IPV6 address*/ 00098 #define PAL_NET_DNS_IPV4_ONLY 2 /* if PAL_NET_DNS_IP_SUPPORT is set to PAL_NET_DNS_IPV4_ONLY pal_getAddressInfo will return the first available IPV4 address*/ 00099 #define PAL_NET_DNS_IPV6_ONLY 4 /* if PAL_NET_DNS_IP_SUPPORT is set to PAL_NET_DNS_IPV6_ONLY pal_getAddressInfo will return the first available IPV6 address*/ 00100 00101 00102 #ifndef PAL_NET_DNS_IP_SUPPORT 00103 #if PAL_SUPPORT_IP_V6 == true && PAL_SUPPORT_IP_V4 == true 00104 #define PAL_NET_DNS_IP_SUPPORT 0 /* sets the type of IP addresses returned by pal_getAddressInfo*/ 00105 #elif PAL_SUPPORT_IP_V6 == true 00106 #define PAL_NET_DNS_IP_SUPPORT 4 /* sets the type of IP addresses returned by pal_getAddressInfo*/ 00107 #else 00108 #define PAL_NET_DNS_IP_SUPPORT 2 /* sets the type of IP addresses returned by pal_getAddressInfo*/ 00109 #endif 00110 00111 #endif 00112 00113 //! The maximum number of interfaces that can be supported at a time. 00114 #ifndef PAL_MAX_SUPORTED_NET_INTERFACES 00115 #define PAL_MAX_SUPORTED_NET_INTERFACES 10 00116 #endif 00117 00118 //!< Stack size for thread created when calling pal_getAddressInfoAsync 00119 #ifndef PAL_NET_ASYNC_DNS_THREAD_STACK_SIZE 00120 #define PAL_NET_ASYNC_DNS_THREAD_STACK_SIZE (1024 * 2) 00121 #endif 00122 00123 00124 //! If you want PAL Not to perform a rollback/cleanup although main PAL init failed, please set this flag to `false` 00125 #ifndef PAL_CLEANUP_ON_INIT_FAILURE 00126 #define PAL_CLEANUP_ON_INIT_FAILURE true 00127 #endif 00128 00129 /* 00130 * RTOS configuration 00131 */ 00132 //! This flag determines if PAL moudles are thread safe. 1 - thread safety is enabled, 0 - thread safety is disabled 00133 #ifndef PAL_THREAD_SAFETY 00134 #define PAL_THREAD_SAFETY 1 00135 #endif 00136 00137 //! initial time until thread stack cleanup (mbedOs only). This is the amount of time we wait before checking that a thread has completed so we can free it's stack. 00138 #ifndef PAL_RTOS_THREAD_CLEANUP_TIMER_MILISEC 00139 #define PAL_RTOS_THREAD_CLEANUP_TIMER_MILISEC 200 00140 #endif 00141 00142 //! This define is used to determine the size of the initial random buffer (in bytes) held by PAL for random the algorithm. 00143 #ifndef PAL_INITIAL_RANDOM_SIZE 00144 #define PAL_INITIAL_RANDOM_SIZE 48 00145 #endif 00146 00147 #ifndef PAL_RTOS_WAIT_FOREVER 00148 #define PAL_RTOS_WAIT_FOREVER UINT_MAX 00149 #endif 00150 00151 /* 00152 * TLS configuration 00153 */ 00154 00155 //! The maximum number of supported cipher suites. 00156 #ifndef PAL_MAX_ALLOWED_CIPHER_SUITES 00157 #define PAL_MAX_ALLOWED_CIPHER_SUITES 1 00158 #endif 00159 00160 //! This value is in milliseconds. 1000 = 1 second. 00161 #ifndef PAL_DTLS_PEER_MIN_TIMEOUT 00162 #define PAL_DTLS_PEER_MIN_TIMEOUT 1000 00163 #endif 00164 00165 //! The debug threshold for TLS API. 00166 #ifndef PAL_TLS_DEBUG_THRESHOLD 00167 #define PAL_TLS_DEBUG_THRESHOLD 5 00168 #endif 00169 00170 //! 32 or 48 (depends on the curve) bytes for the X,Y coordinates and 1 for the normalized/non-normalized 00171 #ifndef PAL_CERT_ID_SIZE 00172 #define PAL_CERT_ID_SIZE 33 00173 #endif 00174 00175 00176 #ifndef PAL_ENABLE_PSK 00177 #define PAL_ENABLE_PSK 0 00178 #endif 00179 00180 #ifndef PAL_ENABLE_X509 00181 #define PAL_ENABLE_X509 1 00182 #endif 00183 00184 //! Define the cipher suites for TLS (only one cipher suite per device available). 00185 #define PAL_TLS_PSK_WITH_AES_128_CBC_SHA256_SUITE 0x01 00186 #define PAL_TLS_PSK_WITH_AES_128_CCM_8_SUITE 0x02 00187 #define PAL_TLS_PSK_WITH_AES_256_CCM_8_SUITE 0x04 00188 #define PAL_TLS_ECDHE_ECDSA_WITH_AES_128_CCM_8_SUITE 0x08 00189 #define PAL_TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256_SUITE 0x10 00190 #define PAL_TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384_SUITE 0x20 00191 #define PAL_TLS_ECDHE_ECDSA_WITH_ARIA_128_GCM_SHA256_SUITE 0x40 00192 #define PAL_TLS_ECDHE_ECDSA_WITH_ARIA_128_CBC_SHA256_SUITE 0x80 00193 00194 //! Use the default cipher suite for TLS/DTLS operations 00195 #if (PAL_ENABLE_X509 == 1) 00196 #ifndef PAL_TLS_CIPHER_SUITE 00197 #define PAL_TLS_CIPHER_SUITE PAL_TLS_ECDHE_ECDSA_WITH_AES_128_CCM_8_SUITE 00198 #endif 00199 #elif (PAL_ENABLE_PSK == 1) 00200 #ifndef PAL_TLS_CIPHER_SUITE 00201 #define PAL_TLS_CIPHER_SUITE PAL_TLS_PSK_WITH_AES_128_CCM_8_SUITE 00202 #endif 00203 #endif 00204 00205 #ifndef PAL_CMAC_SUPPORT 00206 #define PAL_CMAC_SUPPORT true 00207 #endif 00208 00209 //! Enable the CMAC functionality (This flag was targeted to let the bootloader to be compiled without CMAC) 00210 #ifndef PAL_CMAC_SUPPORT 00211 #define PAL_CMAC_SUPPORT 1 00212 #endif //PAL_CMAC_SUPPORT 00213 00214 /* 00215 * UPDATE configuration 00216 */ 00217 00218 #define PAL_UPDATE_USE_FLASH 1 00219 #define PAL_UPDATE_USE_FS 2 00220 00221 #ifndef PAL_UPDATE_IMAGE_LOCATION 00222 #define PAL_UPDATE_IMAGE_LOCATION PAL_UPDATE_USE_FS //!< Choose the storage correct Storage option, File System or Flash 00223 #endif 00224 00225 //! Certificate date validation in Unix time format. 00226 #ifndef PAL_CRYPTO_CERT_DATE_LENGTH 00227 #define PAL_CRYPTO_CERT_DATE_LENGTH sizeof(uint64_t) 00228 #endif 00229 00230 /* 00231 * FS configuration 00232 */ 00233 00234 /* !\brief file system configurations 00235 * PAL_NUMBER_OF_PARTITIONS 00236 * 0 - Default behavior for the platform (Described by either 1 or 2 below). 00237 * 1 - There is a single partition in which the ARM client applications create and remove files (but do not format it). 00238 * 2 - There are two partitions in which ARM client applications may format or create and remove files, 00239 * depending on PAL_PRIMARY_PARTITION_PRIVATE and PAL_SECONDARY_PARTITION_PRIVATE 00240 */ 00241 #ifndef PAL_NUMBER_OF_PARTITIONS 00242 #define PAL_NUMBER_OF_PARTITIONS 1 // Default partitions 00243 #endif 00244 00245 #if (PAL_NUMBER_OF_PARTITIONS > 2) 00246 #error "PAL_NUMBER_OF_PARTITIONS cannot be more then 2" 00247 #endif 00248 00249 // PAL_PRIMARY_PARTITION_PRIVATE 00250 // 1 if the primary partition is exclusively dedicated to the ARM client applications. 00251 // 0 if the primary partition is used for storing other files as well. 00252 #ifndef PAL_PRIMARY_PARTITION_PRIVATE 00253 #define PAL_PRIMARY_PARTITION_PRIVATE 0 00254 #endif 00255 00256 //! PAL_SECONDARY_PARTITION_PRIVATE 00257 //! 1 if the secondary partition is exclusively dedicated to the ARM client applications. 00258 //! 0 if the secondary partition is used for storing other files as well. 00259 #ifndef PAL_SECONDARY_PARTITION_PRIVATE 00260 #define PAL_SECONDARY_PARTITION_PRIVATE 0 00261 #endif 00262 00263 //! This define is the location of the primary mount point for the file system 00264 #ifndef PAL_FS_MOUNT_POINT_PRIMARY 00265 #define PAL_FS_MOUNT_POINT_PRIMARY "" 00266 #endif 00267 00268 //! This define is the location of the secondary mount point for the file system 00269 #ifndef PAL_FS_MOUNT_POINT_SECONDARY 00270 #define PAL_FS_MOUNT_POINT_SECONDARY "" 00271 #endif 00272 00273 // Update 00274 00275 #ifndef PAL_UPDATE_FIRMWARE_MOUNT_POINT 00276 #define PAL_UPDATE_FIRMWARE_MOUNT_POINT PAL_FS_MOUNT_POINT_PRIMARY 00277 #endif 00278 //! The location of the firmware update folder 00279 #ifndef PAL_UPDATE_FIRMWARE_DIR 00280 #define PAL_UPDATE_FIRMWARE_DIR PAL_UPDATE_FIRMWARE_MOUNT_POINT "/firmware" 00281 #endif 00282 00283 /*\brief If flash existed set to 1 else 0, the flash is used for none volatile backup*/ 00284 #ifndef PAL_USE_INTERNAL_FLASH 00285 #define PAL_USE_INTERNAL_FLASH 0 00286 #endif 00287 00288 #ifndef PAL_INT_FLASH_NUM_SECTIONS 00289 #define PAL_INT_FLASH_NUM_SECTIONS 0 00290 #endif 00291 00292 #ifndef PAL_USE_HW_ROT 00293 #define PAL_USE_HW_ROT 1 00294 #endif 00295 00296 #ifndef PAL_USE_HW_RTC 00297 #define PAL_USE_HW_RTC 1 00298 #endif 00299 00300 #ifndef PAL_USE_HW_TRNG 00301 #define PAL_USE_HW_TRNG 1 00302 #endif 00303 00304 //! The number of valid priorities limits the number of concurrent running threads. 00305 #ifndef PAL_MAX_NUMBER_OF_THREADS 00306 #if PAL_USE_HW_TRNG 00307 #define PAL_MAX_NUMBER_OF_THREADS 9 00308 #else 00309 #define PAL_MAX_NUMBER_OF_THREADS 8 00310 #endif 00311 #endif 00312 00313 #if PAL_USE_HW_TRNG 00314 //! Delay for TRNG noise collecting thread used between calls to TRNG 00315 #ifndef PAL_NOISE_TRNG_THREAD_DELAY_MILLI_SEC 00316 #define PAL_NOISE_TRNG_THREAD_DELAY_MILLI_SEC (1000 * 60) // one minute 00317 #endif 00318 //! Stack size for TRNG noise collecting thread 00319 #ifndef PAL_NOISE_TRNG_THREAD_STACK_SIZE 00320 #define PAL_NOISE_TRNG_THREAD_STACK_SIZE 1536 // 1.5K 00321 #endif 00322 #endif 00323 00324 #ifndef PAL_USE_SECURE_TIME 00325 #define PAL_USE_SECURE_TIME 1 00326 #endif 00327 00328 #ifndef PAL_DEVICE_KEY_DERIVATION_BACKWARD_COMPATIBILITY_CALC 00329 #define PAL_DEVICE_KEY_DERIVATION_BACKWARD_COMPATIBILITY_CALC 0 00330 #endif 00331 00332 /*\brief Starting Address for section 1 Minimum requirement size is 1KB and section must be consecutive sectors*/ 00333 #ifndef PAL_INTERNAL_FLASH_SECTION_1_ADDRESS 00334 #define PAL_INTERNAL_FLASH_SECTION_1_ADDRESS 0 00335 #endif 00336 /*\brief Starting Address for section 2 Minimum requirement size is 1KB and section must be consecutive sectors*/ 00337 #ifndef PAL_INTERNAL_FLASH_SECTION_2_ADDRESS 00338 #define PAL_INTERNAL_FLASH_SECTION_2_ADDRESS 0 00339 #endif 00340 /*\brief Size for section 1*/ 00341 #ifndef PAL_INTERNAL_FLASH_SECTION_1_SIZE 00342 #define PAL_INTERNAL_FLASH_SECTION_1_SIZE 0 00343 #endif 00344 /*\brief Size for section 2*/ 00345 #ifndef PAL_INTERNAL_FLASH_SECTION_2_SIZE 00346 #define PAL_INTERNAL_FLASH_SECTION_2_SIZE 0 00347 #endif 00348 00349 #ifndef PAL_SIMULATOR_TEST_ENABLE 00350 #define PAL_SIMULATOR_TEST_ENABLE 0 00351 #endif 00352 00353 00354 00355 #if (PAL_SIMULATOR_TEST_ENABLE == 1) 00356 00357 #undef PAL_SIMULATE_RTOS_REBOOT 00358 #define PAL_SIMULATE_RTOS_REBOOT 1 00359 00360 #undef PAL_SIMULATOR_FLASH_OVER_FILE_SYSTEM 00361 #define PAL_SIMULATOR_FLASH_OVER_FILE_SYSTEM 1 00362 00363 /*\brief overwrite format command with remove all file and directory only for Linux*/ 00364 #undef PAL_SIMULATOR_FS_RM_INSTEAD_OF_FORMAT 00365 #define PAL_SIMULATOR_FS_RM_INSTEAD_OF_FORMAT 1 00366 #endif //PAL_SIMULATOR_TEST_ENABLE 00367 00368 #ifndef PAL_SIMULATOR_FLASH_OVER_FILE_SYSTEM 00369 #define PAL_SIMULATOR_FLASH_OVER_FILE_SYSTEM 0 00370 #endif 00371 00372 00373 00374 #if PAL_SIMULATOR_FLASH_OVER_FILE_SYSTEM 00375 00376 00377 #undef PAL_USE_INTERNAL_FLASH 00378 #define PAL_USE_INTERNAL_FLASH 1 00379 00380 #undef PAL_INT_FLASH_NUM_SECTIONS 00381 #define PAL_INT_FLASH_NUM_SECTIONS 2 00382 00383 #ifndef PAL_SIMULATOR_SOTP_AREA_SIZE 00384 #define PAL_SIMULATOR_SOTP_AREA_SIZE 4096 /*\brief must be power of two the can be divded to page size without reminder and must be a multiple of sector size*/ 00385 #endif 00386 00387 #ifndef SIMULATE_FLASH_SECTOR_SIZE 00388 #define SIMULATE_FLASH_SECTOR_SIZE 4096 /*\brief Flash Sector size*/ 00389 #endif 00390 00391 #ifndef SIMULATE_FLASH_DIR 00392 #define SIMULATE_FLASH_DIR "" /*\brief Directory that holds the flash simulator file*/ 00393 #endif 00394 00395 #ifndef SIMULATE_FLASH_FILE_NAME 00396 #define SIMULATE_FLASH_FILE_NAME SIMULATE_FLASH_DIR"/flashSim" /*\brief File name and path to the flash simulator file*/ 00397 #endif 00398 00399 #ifndef SIMULATE_FLASH_PAGE_SIZE 00400 #define SIMULATE_FLASH_PAGE_SIZE 8 /*\brief Minumum writing uint to flash (2, 4, 8, 16)*/ 00401 #endif 00402 00403 #if PAL_SIMULATOR_SOTP_AREA_SIZE < 4096 00404 #error Minimum Size of 4K 00405 #endif 00406 00407 /*\brief Note - In simulator mode all flash areas are overriden with the simulation sizes and address*/ 00408 00409 #undef PAL_INTERNAL_FLASH_SECTION_1_SIZE 00410 /*\brief Size for section 1*/ 00411 #define PAL_INTERNAL_FLASH_SECTION_1_SIZE PAL_SIMULATOR_SOTP_AREA_SIZE 00412 00413 #undef PAL_INTERNAL_FLASH_SECTION_2_SIZE 00414 /*\brief Size for section 2*/ 00415 #define PAL_INTERNAL_FLASH_SECTION_2_SIZE PAL_SIMULATOR_SOTP_AREA_SIZE 00416 00417 #undef PAL_INTERNAL_FLASH_SECTION_1_ADDRESS 00418 /*\brief Starting Address for section 1 Minimum requirement size is 1KB and section must be consecutive sectors*/ 00419 #define PAL_INTERNAL_FLASH_SECTION_1_ADDRESS 0 00420 00421 #undef PAL_INTERNAL_FLASH_SECTION_2_ADDRESS 00422 /*\brief Starting Address for section 2 Minimum requirement size is 1KB and section must be consecutive sectors*/ 00423 #define PAL_INTERNAL_FLASH_SECTION_2_ADDRESS PAL_INTERNAL_FLASH_SECTION_1_SIZE 00424 00425 #endif //PAL_SIMULATOR_FLASH_OVER_FILE_SYSTEM 00426 00427 00428 #define VALUE_TO_STRING(x) #x 00429 #define VALUE(x) VALUE_TO_STRING(x) 00430 #define VAR_NAME_VALUE(var) #var " = " VALUE(var) 00431 00432 #if ((!PAL_USE_INTERNAL_FLASH && (!PAL_USE_HW_ROT || !PAL_USE_HW_RTC || !PAL_USE_HW_TRNG)) \ 00433 || ((PAL_INT_FLASH_NUM_SECTIONS == 1) && PAL_USE_INTERNAL_FLASH && (!PAL_USE_HW_RTC || !PAL_USE_HW_TRNG))) 00434 #pragma message(VAR_NAME_VALUE(PAL_USE_INTERNAL_FLASH)) 00435 #pragma message(VAR_NAME_VALUE(PAL_USE_HW_ROT)) 00436 #pragma message(VAR_NAME_VALUE(PAL_USE_HW_RTC)) 00437 #pragma message(VAR_NAME_VALUE(PAL_USE_HW_TRNG)) 00438 #pragma message(VAR_NAME_VALUE(PAL_INT_FLASH_NUM_SECTIONS)) 00439 #error Minimum configuration setting does not meet the requirements 00440 #endif 00441 00442 #if (((PAL_ENABLE_PSK == 1) && (PAL_ENABLE_X509 == 1)) && !(defined(__LINUX__))) 00443 #error "Please select only one option PSK/X509" 00444 #endif 00445 00446 #if ((PAL_ENABLE_PSK == 0) && (PAL_ENABLE_X509 == 0)) 00447 #error "Please select one option PSK/X509" 00448 #endif 00449 00450 00451 00452 #if ((PAL_ENABLE_PSK == 1) && (PAL_USE_SECURE_TIME == 1)) 00453 #error "PSK feature cannot be configured along with secure time" 00454 #endif 00455 00456 00457 00458 //! Delay (in milliseconds) between calls to TRNG random buffer in case only partial data (PAL_ERR_RTOS_TRNG_PARTIAL_DATA) was generated for the function call 00459 #ifndef PAL_TRNG_COLLECT_DELAY_MILLI_SEC 00460 #define PAL_TRNG_COLLECT_DELAY_MILLI_SEC 1000 00461 #endif // !PAL_TRNG_COLLECT_DELAY_MILLI_SEC 00462 00463 //! define the number of images 00464 #ifndef IMAGE_COUNT_MAX 00465 #define IMAGE_COUNT_MAX 1 00466 #endif 00467 00468 #define PAL_NOISE_SIZE_BYTES 48 // max number of bytes for noise 00469 #define PAL_NOISE_SIZE_BITS (PAL_NOISE_SIZE_BYTES * CHAR_BIT) // max number of bits for noise 00470 #define PAL_NOISE_BUFFER_LEN (PAL_NOISE_SIZE_BYTES / sizeof(int32_t)) // length of the noise buffer 00471 00472 #endif //_PAL_COFIGURATION_H
Generated on Tue Jul 12 2022 19:12:14 by
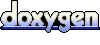