
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
nsdlaccesshelper.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "include/nsdlaccesshelper.h" 00017 #include "include/m2mnsdlinterface.h" 00018 #include "sn_nsdl_lib.h" 00019 #include "sn_grs.h" 00020 #include <stdlib.h> 00021 00022 // callback function for NSDL library to call into 00023 uint8_t __nsdl_c_callback(struct nsdl_s *nsdl_handle, 00024 sn_coap_hdr_s *received_coap_ptr, 00025 sn_nsdl_addr_s *address, 00026 sn_nsdl_capab_e nsdl_capab) 00027 { 00028 uint8_t status = 0; 00029 M2MNsdlInterface *interface = (M2MNsdlInterface*)sn_nsdl_get_context(nsdl_handle); 00030 if(interface) { 00031 status = interface->resource_callback(nsdl_handle, 00032 received_coap_ptr, 00033 address, nsdl_capab); 00034 } 00035 return status; 00036 } 00037 00038 void* __nsdl_c_memory_alloc(uint16_t size) 00039 { 00040 if(size) 00041 return malloc(size); 00042 else 00043 return 0; 00044 } 00045 00046 void __nsdl_c_memory_free(void *ptr) 00047 { 00048 if(ptr) 00049 free(ptr); 00050 } 00051 00052 uint8_t __nsdl_c_send_to_server(struct nsdl_s * nsdl_handle, 00053 sn_nsdl_capab_e protocol, 00054 uint8_t *data_ptr, 00055 uint16_t data_len, 00056 sn_nsdl_addr_s *address_ptr) 00057 { 00058 uint8_t status = 0; 00059 M2MNsdlInterface *interface = (M2MNsdlInterface*)sn_nsdl_get_context(nsdl_handle); 00060 #if defined(FEA_TRACE_SUPPORT) || MBED_CONF_MBED_TRACE_ENABLE || YOTTA_CFG_MBED_TRACE || (defined(YOTTA_CFG) && !defined(NDEBUG)) 00061 coap_version_e version = COAP_VERSION_UNKNOWN; 00062 sn_coap_hdr_s *header = sn_coap_parser(nsdl_handle->grs->coap, data_len, data_ptr, &version); 00063 sn_nsdl_print_coap_data(header, true); 00064 sn_coap_parser_release_allocated_coap_msg_mem(nsdl_handle->grs->coap, header); 00065 #endif 00066 if(interface) { 00067 status = interface->send_to_server_callback(nsdl_handle, 00068 protocol, data_ptr, 00069 data_len, address_ptr); 00070 } 00071 return status; 00072 } 00073 00074 uint8_t __nsdl_c_received_from_server(struct nsdl_s * nsdl_handle, 00075 sn_coap_hdr_s *coap_header, 00076 sn_nsdl_addr_s *address_ptr) 00077 { 00078 uint8_t status = 0; 00079 M2MNsdlInterface *interface = (M2MNsdlInterface*)sn_nsdl_get_context(nsdl_handle); 00080 if(interface) { 00081 status = interface->received_from_server_callback(nsdl_handle, 00082 coap_header, 00083 address_ptr); 00084 } 00085 return status; 00086 } 00087 00088 uint8_t __nsdl_c_auto_obs_token(struct nsdl_s *nsdl_handle, const char *path, uint8_t *token) 00089 { 00090 M2MNsdlInterface *interface = (M2MNsdlInterface*)sn_nsdl_get_context(nsdl_handle); 00091 if(interface) { 00092 return interface->find_auto_obs_token(path, token); 00093 } 00094 return 0; 00095 } 00096 00097 void* __socket_malloc( void * context, size_t size) 00098 { 00099 (void) context; 00100 return malloc(size); 00101 } 00102 00103 void __socket_free(void * context, void * ptr) 00104 { 00105 (void) context; 00106 free(ptr); 00107 } 00108
Generated on Tue Jul 12 2022 19:12:14 by
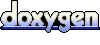