
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
ns_timeout.c
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "eventOS_event.h" 00017 #include "eventOS_event_timer.h" 00018 #include "nsdynmemLIB.h" 00019 #include "ns_list.h" 00020 #include "timer_sys.h" 00021 00022 #define STARTUP_EVENT 0 00023 #define TIMER_EVENT 1 00024 00025 // Timeout structure, already typedefed to timeout_t 00026 struct timeout_entry_t { 00027 void (*callback)(void *); 00028 void *arg; 00029 arm_event_storage_t *event; 00030 }; 00031 00032 static int8_t timeout_tasklet_id = -1; 00033 00034 static void timeout_tasklet(arm_event_s *event) 00035 { 00036 if (TIMER_EVENT != event->event_type) { 00037 return; 00038 } 00039 00040 timeout_t *t = event->data_ptr; 00041 arm_event_storage_t *storage = t->event; 00042 sys_timer_struct_s *timer = NS_CONTAINER_OF(storage, sys_timer_struct_s, event); 00043 00044 t->callback(t->arg); 00045 00046 00047 // Check if this was periodic timer 00048 if (timer->period == 0) { 00049 ns_dyn_mem_free(event->data_ptr); 00050 } 00051 } 00052 00053 static timeout_t *eventOS_timeout_at_(void (*callback)(void *), void *arg, uint32_t at, uint32_t period) 00054 { 00055 arm_event_storage_t *storage; 00056 00057 timeout_t *timeout = ns_dyn_mem_alloc(sizeof(timeout_t)); 00058 if (!timeout) { 00059 return NULL; 00060 } 00061 timeout->callback = callback; 00062 timeout->arg = arg; 00063 00064 // Start timeout taskled if it is not running 00065 if (-1 == timeout_tasklet_id) { 00066 timeout_tasklet_id = eventOS_event_handler_create(timeout_tasklet, STARTUP_EVENT); 00067 if (timeout_tasklet_id < 0) { 00068 timeout_tasklet_id = -1; 00069 goto FAIL; 00070 } 00071 } 00072 00073 arm_event_t event = { 00074 .receiver = timeout_tasklet_id, 00075 .sender = timeout_tasklet_id, 00076 .event_type = TIMER_EVENT, 00077 .event_id = TIMER_EVENT, 00078 .data_ptr = timeout 00079 }; 00080 00081 if (period) 00082 storage = eventOS_event_timer_request_every(&event, period); 00083 else 00084 storage = eventOS_event_timer_request_at(&event, at); 00085 00086 timeout->event = storage; 00087 if (storage) 00088 return timeout; 00089 FAIL: 00090 ns_dyn_mem_free(timeout); 00091 return NULL; 00092 } 00093 00094 timeout_t *eventOS_timeout_ms(void (*callback)(void *), uint32_t ms, void *arg) 00095 { 00096 return eventOS_timeout_at_(callback, arg, eventOS_event_timer_ms_to_ticks(ms)+eventOS_event_timer_ticks(), 0); 00097 } 00098 00099 timeout_t *eventOS_timeout_every_ms(void (*callback)(void *), uint32_t every, void *arg) 00100 { 00101 return eventOS_timeout_at_(callback, arg, 0, eventOS_event_timer_ms_to_ticks(every)); 00102 } 00103 00104 void eventOS_timeout_cancel(timeout_t *t) 00105 { 00106 if (!t) 00107 return; 00108 00109 eventOS_cancel(t->event); 00110 00111 // Defer the freeing until returning from the callback 00112 if (t->event->state != ARM_LIB_EVENT_RUNNING) { 00113 ns_dyn_mem_free(t); 00114 } 00115 }
Generated on Tue Jul 12 2022 19:12:14 by
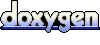