
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
manifest-manager-api.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "arm_uc_mmCommon.h" 00020 #include "arm_uc_mmConfig.h" 00021 #include "arm_uc_mmStateSelector.h" 00022 #include "arm_uc_mmInit.h" 00023 00024 #include "update-client-manifest-manager/update-client-manifest-manager.h" 00025 #include "update-client-manifest-manager/update-client-manifest-manager-context.h" 00026 #include "update-client-manifest-manager/update-client-manifest-types.h" 00027 #include "update-client-manifest-manager/arm-pal-kv.h" 00028 00029 #include "update-client-common/arm_uc_scheduler.h" 00030 #include "update-client-common/arm_uc_utilities.h" 00031 #include "update-client-common/arm_uc_error.h" 00032 00033 #include <stdint.h> 00034 #include <stdio.h> 00035 #include <stddef.h> 00036 #include <string.h> 00037 00038 /** 00039 * @file manifest_manager.c 00040 * @brief Manifest Manager API 00041 * @details This file specifies the API used to interact with the manifest manager 00042 */ 00043 00044 arm_uc_error_t ARM_UC_mmInit(arm_uc_mmContext_t** mmCtx, void (*event_handler)(uint32_t), const arm_pal_key_value_api* api) 00045 { 00046 arm_uc_error_t err = {MFST_ERR_NONE}; 00047 if (mmCtx == NULL || *mmCtx == NULL) 00048 { 00049 return (arm_uc_error_t){MFST_ERR_NULL_PTR}; 00050 } 00051 00052 arm_uc_mmPersistentContext.ctx = mmCtx; 00053 arm_uc_mmPersistentContext.applicationEventHandler = event_handler; 00054 arm_uc_mmPersistentContext.testFSM = NULL; 00055 00056 // initialize callback node 00057 arm_uc_mmPersistentContext.applicationCallbackStorage.lock = 0; 00058 00059 ARM_UC_PostCallback(&arm_uc_mmPersistentContext.applicationCallbackStorage, event_handler, ARM_UC_MM_RC_DONE); 00060 // This code will be re-enabled when storage is available 00061 #if 0 00062 ARM_UC_mmCfStoreInit(api); 00063 00064 // Initialize the Init FSM 00065 arm_uc_mmContext_t* ctx = *mmCtx; 00066 ctx->init.state = ARM_UC_MM_INIT_BEGIN; 00067 00068 err = ARM_UC_mmSetState(ARM_UC_MM_STATE_INIT); 00069 if (err.code != MFST_ERR_NONE) 00070 { 00071 return err; 00072 } 00073 // Start the Init FSM 00074 ARM_UC_PostCallback(&ctx->init.callbackStorage, ARM_UC_mmCallbackFSMEntry, ARM_UC_MM_EVENT_BEGIN); 00075 #endif 00076 return err; 00077 } 00078 00079 arm_uc_error_t ARM_UC_mmInsert(arm_uc_mmContext_t** ctx, arm_uc_buffer_t* buffer, arm_uc_buffer_t* certificateStorage, arm_uc_manifest_handle_t* ID) 00080 { 00081 if (ctx == NULL || *ctx == NULL || buffer == NULL ) 00082 { 00083 return (arm_uc_error_t){MFST_ERR_NULL_PTR}; 00084 } 00085 arm_uc_mmPersistentContext.ctx = ctx; 00086 // Setup the state machine 00087 arm_uc_error_t err = ARM_UC_mmSetState(ARM_UC_MM_STATE_INSERTING); 00088 if (err.code != MFST_ERR_NONE) 00089 { 00090 return err; 00091 } 00092 struct arm_uc_mmInsertContext_t* insertCtx = &(*arm_uc_mmPersistentContext.ctx)->insert; 00093 // Store the buffer pointer 00094 ARM_UC_buffer_shallow_copy(&insertCtx->manifest, buffer); 00095 insertCtx->state = ARM_UC_MM_INS_STATE_BEGIN; 00096 // Store the ID pointer 00097 insertCtx->ID = ID; 00098 // Store the certificate buffer 00099 ARM_UC_buffer_shallow_copy(&insertCtx->certificateStorage, certificateStorage); 00100 00101 // initialize callback node 00102 insertCtx->callbackStorage.lock = 0; 00103 00104 // Start the FSM 00105 ARM_UC_PostCallback(&insertCtx->callbackStorage, ARM_UC_mmCallbackFSMEntry, ARM_UC_MM_EVENT_BEGIN); 00106 return (arm_uc_error_t){MFST_ERR_NONE}; 00107 } 00108 arm_uc_error_t ARM_UC_mmFetchFirmwareInfo(arm_uc_mmContext_t** ctx, struct manifest_firmware_info_t* info, const arm_uc_manifest_handle_t* ID) 00109 { 00110 if (ctx == NULL || *ctx == NULL || info == NULL ) 00111 { 00112 return (arm_uc_error_t){MFST_ERR_NULL_PTR}; 00113 } 00114 arm_uc_mmPersistentContext.ctx = ctx; 00115 // Initialize the state machine 00116 arm_uc_error_t err = ARM_UC_mmSetState(ARM_UC_MM_STATE_FWINFO); 00117 if (err.code != MFST_ERR_NONE) 00118 { 00119 return err; 00120 } 00121 struct arm_uc_mm_fw_context_t* fwCtx = &(*arm_uc_mmPersistentContext.ctx)->getFw; 00122 fwCtx->state = ARM_UC_MM_FW_STATE_BEGIN; 00123 fwCtx->info = info; 00124 00125 // initialize callback node 00126 fwCtx->callbackStorage.lock = 0; 00127 00128 // Start the state machine 00129 ARM_UC_PostCallback(&fwCtx->callbackStorage, ARM_UC_mmCallbackFSMEntry, ARM_UC_MM_EVENT_BEGIN); 00130 00131 return (arm_uc_error_t){MFST_ERR_NONE}; 00132 } 00133 arm_uc_error_t ARM_UC_mmFetchNextFirmwareInfo(struct manifest_firmware_info_t* info) 00134 { 00135 struct arm_uc_mm_fw_context_t* fwCtx = &(*arm_uc_mmPersistentContext.ctx)->getFw; 00136 fwCtx->info = info; 00137 00138 // initialize callback node 00139 fwCtx->callbackStorage.lock = 0; 00140 00141 // Continue the state machine 00142 ARM_UC_PostCallback(&fwCtx->callbackStorage, ARM_UC_mmCallbackFSMEntry, ARM_UC_MM_EVENT_BEGIN); 00143 return (arm_uc_error_t){MFST_ERR_NONE}; 00144 } 00145 arm_uc_error_t ARM_UC_mmGetError() 00146 { 00147 return arm_uc_mmPersistentContext.reportedError; 00148 } 00149 00150 #if ARM_UC_MM_ENABLE_TEST_VECTORS 00151 arm_uc_error_t ARM_UC_mmRegisterTestHook(ARM_UC_mmTestHook_t hook) 00152 { 00153 arm_uc_error_t err = {MFST_ERR_NONE}; 00154 arm_uc_mmPersistentContext.testHook = hook; 00155 return err; 00156 } 00157 #endif
Generated on Tue Jul 12 2022 19:12:13 by
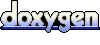