
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mvector.h
00001 /* 00002 * Copyright (c) 2015-2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef M2M_VECTOR_H 00018 #define M2M_VECTOR_H 00019 00020 /*! \file m2mvector.h 00021 * \brief A simple C++ Vector class, used as replacement for std::vector. 00022 */ 00023 00024 00025 namespace m2m 00026 { 00027 00028 template <typename ObjectTemplate> 00029 00030 class Vector 00031 { 00032 public: 00033 explicit Vector( int init_size = MIN_CAPACITY) 00034 : _size(0), 00035 _capacity((init_size >= MIN_CAPACITY) ? init_size : MIN_CAPACITY) { 00036 _object_template = new ObjectTemplate[ _capacity ]; 00037 } 00038 00039 Vector(const Vector & rhs ): _object_template(0) { 00040 operator=(rhs); 00041 } 00042 00043 ~Vector() { 00044 delete [] _object_template; 00045 } 00046 00047 const Vector & operator=(const Vector & rhs) { 00048 if(this != &rhs) { 00049 delete[] _object_template; 00050 _size = rhs.size(); 00051 _capacity = rhs._capacity; 00052 00053 _object_template = new ObjectTemplate[capacity()]; 00054 for(int k = 0; k < size(); k++) { 00055 _object_template[k] = rhs._object_template[k]; 00056 } 00057 } 00058 return *this; 00059 } 00060 00061 void resize(int new_size) { 00062 if(new_size > _capacity) { 00063 reserve(new_size * 2 + 1); 00064 } 00065 _size = new_size; 00066 } 00067 00068 void reserve(int new_capacity) { 00069 if(new_capacity < _size) { 00070 return; 00071 } 00072 ObjectTemplate *old_array = _object_template; 00073 00074 _object_template = new ObjectTemplate[new_capacity]; 00075 for(int k = 0; k < _size; k++) { 00076 _object_template[k] = old_array[k]; 00077 } 00078 _capacity = new_capacity; 00079 delete [] old_array; 00080 } 00081 00082 ObjectTemplate & operator[](int idx) { 00083 return _object_template[idx]; 00084 } 00085 00086 const ObjectTemplate& operator[](int idx) const { 00087 return _object_template[idx]; 00088 } 00089 00090 bool empty() const{ 00091 return size() == 0; 00092 } 00093 00094 int size() const { 00095 return _size; 00096 } 00097 00098 int capacity() const { 00099 return _capacity; 00100 } 00101 00102 void push_back(const ObjectTemplate& x) { 00103 if(_size == _capacity) { 00104 reserve(2 * _capacity + 1); 00105 } 00106 _object_template[_size] = x; 00107 _size++; 00108 } 00109 00110 void pop_back() { 00111 _size--; 00112 } 00113 00114 void clear() { 00115 _size = 0; 00116 } 00117 00118 const ObjectTemplate& back() const { 00119 return _object_template[_size - 1]; 00120 } 00121 00122 typedef ObjectTemplate* iterator; 00123 typedef const ObjectTemplate* const_iterator; 00124 00125 iterator begin() { 00126 return &_object_template[0]; 00127 } 00128 00129 const_iterator begin() const { 00130 return &_object_template[0]; 00131 } 00132 00133 iterator end() { 00134 return &_object_template[_size]; 00135 } 00136 00137 const_iterator end() const { 00138 return &_object_template[_size]; 00139 } 00140 00141 void erase(int position) { 00142 if(position < _size) { 00143 for(int k = position; k + 1 < _size; k++) { 00144 _object_template[k] = _object_template[k + 1]; 00145 } 00146 _size--; 00147 } 00148 } 00149 00150 enum { 00151 MIN_CAPACITY = 1 00152 }; 00153 00154 private: 00155 int _size; 00156 int _capacity; 00157 ObjectTemplate* _object_template; 00158 }; 00159 00160 } // namespace 00161 00162 #endif // M2M_VECTOR_H
Generated on Tue Jul 12 2022 19:12:13 by
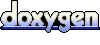