
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mstringbuffer.h
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef __STRING_BUFFER_H__ 00017 #define __STRING_BUFFER_H__ 00018 00019 #include "mbed-client/m2mstringbufferbase.h" 00020 00021 #include <assert.h> 00022 #include <stddef.h> 00023 00024 template <int SIZE> 00025 class StringBuffer : private StringBufferBase 00026 { 00027 public: 00028 /** 00029 * Initialize a empty buffer with zero length and zero content. 00030 */ 00031 inline StringBuffer(); 00032 00033 // 00034 // This is not implemented on purpose, as the given string may conflict with 00035 // templated size. Otoh, if we used compile time assert, the overflow 00036 // could be prevented at compile time. 00037 // 00038 // inline StringBuffer(const char *initial_string); 00039 00040 /** 00041 * Verify, if the buffer has still room for given amount of bytes. 00042 * Note: the given size value must include the zero terminator as it is not 00043 * implicitly taken into account for. 00044 */ 00045 bool ensure_space(size_t required_size) const; 00046 00047 /** 00048 * Append given char to end of string. 00049 * Return false if the buffer would overflow, true otherwise. 00050 */ 00051 bool append(char data); 00052 00053 /** 00054 * Append given zero terminated string to end of buffer. 00055 * 00056 * Return false if the buffer would overflow, true otherwise. 00057 * 00058 * Note: the whole string, including the zero terminator must fit 00059 * to buffer or the append operation is not done and false is returned. 00060 */ 00061 bool append(const char *data); 00062 00063 /** 00064 * Append given block of chars to end of buffer. 00065 * 00066 * Return false if the buffer would overflow, true otherwise. 00067 * 00068 * Note: the whole string, including the zero terminator must fit 00069 * to buffer or the append operation is not done and false is returned. 00070 */ 00071 bool append(const char *data, size_t data_len); 00072 00073 /** 00074 * Convert given uint16_t into string representation and add it to the 00075 * end of buffer. 00076 * 00077 * Note: the whole string, including the zero terminator must fit 00078 * to buffer or the append operation is not done and false is returned. 00079 */ 00080 bool append_int(uint16_t data); 00081 00082 /** 00083 * Get the amount of bytes added to the buffer. 00084 * 00085 * Note: the size does not include the terminating zero, so this is 00086 * functionally equal to strlen(). 00087 */ 00088 inline size_t get_size() const; 00089 00090 // API functionality copied from m2mstring: 00091 00092 // find the index of last occurance of given char in string, or negative if not found 00093 int find_last_of(char search_char) const; 00094 00095 /** 00096 * Get a read only pointer to the data. 00097 */ 00098 inline const char* c_str() const; 00099 00100 // Add this only if needed 00101 //inline char* c_str(); 00102 private: 00103 char _buff[SIZE]; 00104 }; 00105 00106 template <int SIZE> 00107 inline StringBuffer<SIZE>::StringBuffer() 00108 { 00109 // actually a assert_compile() would be better as this is completely a code problem 00110 assert(SIZE > 0); 00111 00112 _buff[0] = '\0'; 00113 } 00114 00115 template <int SIZE> 00116 bool StringBuffer<SIZE>::ensure_space(size_t required_size) const 00117 { 00118 return StringBufferBase::ensure_space(SIZE, required_size); 00119 } 00120 00121 template <int SIZE> 00122 bool StringBuffer<SIZE>::append(const char *data) 00123 { 00124 return StringBufferBase::append(_buff, SIZE, data); 00125 } 00126 00127 template <int SIZE> 00128 bool StringBuffer<SIZE>::append(const char *data, size_t data_len) 00129 { 00130 return StringBufferBase::append(_buff, SIZE, data, data_len); 00131 } 00132 00133 template <int SIZE> 00134 inline bool StringBuffer<SIZE>::append(char data) 00135 { 00136 return StringBufferBase::append(_buff, SIZE, data); 00137 } 00138 00139 template <int SIZE> 00140 bool StringBuffer<SIZE>::append_int(uint16_t data) 00141 { 00142 return StringBufferBase::append_int(_buff, SIZE, data); 00143 } 00144 00145 template <int SIZE> 00146 int StringBuffer<SIZE>::find_last_of(char search_char) const 00147 { 00148 return StringBufferBase::find_last_of(_buff, search_char); 00149 } 00150 00151 template <int SIZE> 00152 inline const char* StringBuffer<SIZE>::c_str() const 00153 { 00154 return _buff; 00155 } 00156 00157 template <int SIZE> 00158 inline size_t StringBuffer<SIZE>::get_size() const 00159 { 00160 return _curr_size; 00161 } 00162 00163 #endif // !__STRING_BUFFER_H__
Generated on Tue Jul 12 2022 19:12:13 by
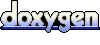