
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mobjectinstance.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_OBJECT_INSTANCE_H 00017 #define M2M_OBJECT_INSTANCE_H 00018 00019 #include "mbed-client/m2mvector.h" 00020 #include "mbed-client/m2mresource.h" 00021 00022 //FORWARD DECLARATION 00023 typedef Vector<M2MResource *> M2MResourceList; 00024 typedef Vector<M2MResourceInstance *> M2MResourceInstanceList; 00025 00026 00027 class M2MObject; 00028 00029 /*! \file m2mobjectinstance.h 00030 * \brief M2MObjectInstance. 00031 * This class is the instance class for mbed Client Objects. All defined 00032 * LWM2M object models can be created based on it. This class also holds all resource 00033 * instances associated with the given object. 00034 */ 00035 00036 class M2MObjectInstance : public M2MBase 00037 { 00038 00039 friend class M2MObject; 00040 00041 private: // Constructor and destructor are private which means 00042 // that these objects can be created or 00043 // deleted only through function provided by M2MObject. 00044 00045 /** 00046 * \brief Constructor 00047 * \param name Name of the object 00048 */ 00049 M2MObjectInstance(M2MObject& parent, 00050 const String &resource_type, 00051 char *path, 00052 bool external_blockwise_store = false); 00053 00054 M2MObjectInstance(M2MObject& parent, const lwm2m_parameters_s* static_res); 00055 00056 // Prevents the use of default constructor. 00057 M2MObjectInstance(); 00058 00059 // Prevents the use of assignment operator. 00060 M2MObjectInstance& operator=( const M2MObjectInstance& /*other*/ ); 00061 00062 // Prevents the use of copy constructor. 00063 M2MObjectInstance( const M2MObjectInstance& /*other*/ ); 00064 00065 /** 00066 * Destructor 00067 */ 00068 virtual ~M2MObjectInstance(); 00069 00070 public: 00071 00072 /** 00073 * \brief TODO! 00074 * \return M2MResource The resource for managing other client operations. 00075 */ 00076 M2MResource* create_static_resource(const lwm2m_parameters_s* static_res, 00077 M2MResourceInstance::ResourceType type); 00078 00079 /** 00080 * \brief Creates a static resource for a given mbed Client Inteface object. With this, the 00081 * client can respond to server's GET methods with the provided value. 00082 * \param resource_name The name of the resource. 00083 * \param resource_type The type of the resource. 00084 * \param value A pointer to the value of the resource. 00085 * \param value_length The length of the value in the pointer. 00086 * \param multiple_instance A resource can have 00087 * multiple instances, default is false. 00088 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00089 * otherwise handled in mbed-client-c. 00090 * \return M2MResource The resource for managing other client operations. 00091 */ 00092 M2MResource* create_static_resource(const String &resource_name, 00093 const String &resource_type, 00094 M2MResourceInstance::ResourceType type, 00095 const uint8_t *value, 00096 const uint8_t value_length, 00097 bool multiple_instance = false, 00098 bool external_blockwise_store = false); 00099 00100 /** 00101 * \brief Creates a dynamic resource for a given mbed Client Inteface object. With this, 00102 * the client can respond to different queries from the server (GET,PUT etc). 00103 * This type of resource is also observable and carries callbacks. 00104 * \param resource_name The name of the resource. 00105 * \param resource_type The type of the resource. 00106 * \param observable Indicates whether the resource is observable or not. 00107 * \param multiple_instance The resource can have 00108 * multiple instances, default is false. 00109 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00110 * otherwise handled in mbed-client-c. 00111 * \return M2MResource The resource for managing other client operations. 00112 */ 00113 M2MResource* create_dynamic_resource(const String &resource_name, 00114 const String &resource_type, 00115 M2MResourceInstance::ResourceType type, 00116 bool observable, 00117 bool multiple_instance = false, 00118 bool external_blockwise_store = false); 00119 00120 /** 00121 * \brief TODO! 00122 * \return M2MResource The resource for managing other client operations. 00123 */ 00124 M2MResource* create_dynamic_resource(const lwm2m_parameters_s* static_res, 00125 M2MResourceInstance::ResourceType type, 00126 bool observable); 00127 00128 /** 00129 * \brief Creates a static resource instance for a given mbed Client Inteface object. With this, 00130 * the client can respond to server's GET methods with the provided value. 00131 * \param resource_name The name of the resource. 00132 * \param resource_type The type of the resource. 00133 * \param value A pointer to the value of the resource. 00134 * \param value_length The length of the value in pointer. 00135 * \param instance_id The instance ID of the resource. 00136 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00137 * otherwise handled in mbed-client-c. 00138 * \return M2MResourceInstance The resource instance for managing other client operations. 00139 */ 00140 M2MResourceInstance* create_static_resource_instance(const String &resource_name, 00141 const String &resource_type, 00142 M2MResourceInstance::ResourceType type, 00143 const uint8_t *value, 00144 const uint8_t value_length, 00145 uint16_t instance_id, 00146 bool external_blockwise_store = false); 00147 00148 /** 00149 * \brief Creates a dynamic resource instance for a given mbed Client Inteface object. With this, 00150 * the client can respond to different queries from the server (GET,PUT etc). 00151 * This type of resource is also observable and carries callbacks. 00152 * \param resource_name The name of the resource. 00153 * \param resource_type The type of the resource. 00154 * \param observable Indicates whether the resource is observable or not. 00155 * \param instance_id The instance ID of the resource. 00156 * \param external_blockwise_store If true CoAP blocks are passed to application through callbacks 00157 * otherwise handled in mbed-client-c. 00158 * \return M2MResourceInstance The resource instance for managing other client operations. 00159 */ 00160 M2MResourceInstance* create_dynamic_resource_instance(const String &resource_name, 00161 const String &resource_type, 00162 M2MResourceInstance::ResourceType type, 00163 bool observable, 00164 uint16_t instance_id, 00165 bool external_blockwise_store = false); 00166 00167 /** 00168 * \brief Removes the resource with the given name. 00169 * \param name The name of the resource to be removed. 00170 * Note: this will be removed in next version, please use the 00171 * remove_resource(const char*) version instead. 00172 * \return True if removed, else false. 00173 */ 00174 bool remove_resource(const String &name); 00175 00176 /** 00177 * \brief Removes the resource with the given name. 00178 * \param name The name of the resource to be removed. 00179 * \return True if removed, else false. 00180 */ 00181 bool remove_resource(const char *name); 00182 00183 /** 00184 * \brief Removes the resource instance with the given name. 00185 * \param resource_name The name of the resource instance to be removed. 00186 * \param instance_id The instance ID of the instance. 00187 * \return True if removed, else false. 00188 */ 00189 bool remove_resource_instance(const String &resource_name, 00190 uint16_t instance_id); 00191 00192 /** 00193 * \brief Returns the resource with the given name. 00194 * \param name The name of the requested resource. 00195 * \return Resource reference if found, else NULL. 00196 */ 00197 M2MResource* resource(const String &name) const; 00198 00199 M2MResource* resource(const char *resource) const; 00200 00201 /** 00202 * \brief Returns a list of M2MResourceBase objects. 00203 * \return A list of M2MResourceBase objects. 00204 */ 00205 const M2MResourceList& resources() const; 00206 00207 /** 00208 * \brief Returns the total number of resources with the object. 00209 * \return Total number of the resources. 00210 */ 00211 uint16_t resource_count() const; 00212 00213 /** 00214 * \brief Returns the total number of single resource instances. 00215 * Note: this will be removed in next version, please use the 00216 * resource_count(const char*) version instead. 00217 * \param resource The name of the resource. 00218 * \return Total number of the resources. 00219 */ 00220 uint16_t resource_count(const String& resource) const; 00221 00222 /** 00223 * \brief Returns the total number of single resource instances. 00224 * \param resource The name of the resource. 00225 * \return Total number of the resources. 00226 */ 00227 uint16_t resource_count(const char *resource) const; 00228 00229 /** 00230 * \brief Adds the observation level for the object. 00231 * \param observation_level The level of observation. 00232 */ 00233 virtual void add_observation_level(M2MBase::Observation observation_level); 00234 00235 /** 00236 * \brief Removes the observation level from the object. 00237 * \param observation_level The level of observation. 00238 */ 00239 virtual void remove_observation_level(M2MBase::Observation observation_level); 00240 00241 /** 00242 * \brief Returns the Observation Handler object. 00243 * \return M2MObservationHandler object. 00244 */ 00245 virtual M2MObservationHandler* observation_handler() const; 00246 00247 /** 00248 * \brief Sets the observation handler 00249 * \param handler Observation handler 00250 */ 00251 virtual void set_observation_handler(M2MObservationHandler *handler); 00252 00253 /** 00254 * \brief Handles GET request for the registered objects. 00255 * \param nsdl The NSDL handler for the CoAP library. 00256 * \param received_coap_header The CoAP message received from the server. 00257 * \param observation_handler The handler object for sending 00258 * observation callbacks. 00259 * return sn_coap_hdr_s The message that needs to be sent to the server. 00260 */ 00261 virtual sn_coap_hdr_s* handle_get_request(nsdl_s *nsdl, 00262 sn_coap_hdr_s *received_coap_header, 00263 M2MObservationHandler *observation_handler = NULL); 00264 /** 00265 * \brief Handles PUT request for the registered objects. 00266 * \param nsdl The NSDL handler for the CoAP library. 00267 * \param received_coap_header The CoAP message received from the server. 00268 * \param observation_handler The handler object for sending 00269 * observation callbacks. 00270 * \param execute_value_updated True will execute the "value_updated" callback. 00271 * \return sn_coap_hdr_s The message that needs to be sent to server. 00272 */ 00273 virtual sn_coap_hdr_s* handle_put_request(nsdl_s *nsdl, 00274 sn_coap_hdr_s *received_coap_header, 00275 M2MObservationHandler *observation_handler, 00276 bool &execute_value_updated); 00277 00278 /** 00279 * \brief Handles POST request for the registered objects. 00280 * \param nsdl The NSDL handler for the CoAP library. 00281 * \param received_coap_header The CoAP message received from the server. 00282 * \param observation_handler The handler object for sending 00283 * observation callbacks. 00284 * \param execute_value_updated True will execute the "value_updated" callback. 00285 * \return sn_coap_hdr_s The message that needs to be sent to server. 00286 */ 00287 virtual sn_coap_hdr_s* handle_post_request(nsdl_s *nsdl, 00288 sn_coap_hdr_s *received_coap_header, 00289 M2MObservationHandler *observation_handler, 00290 bool &execute_value_updated, 00291 sn_nsdl_addr_s *address = NULL); 00292 00293 inline M2MObject& get_parent_object() const; 00294 00295 // callback used from M2MResource/M2MResourceInstance 00296 void notification_update(M2MBase::Observation observation_level); 00297 00298 protected: 00299 virtual M2MBase *get_parent() const; 00300 00301 private: 00302 00303 /** 00304 * \brief Utility function to map M2MResourceInstance ResourceType 00305 * to M2MBase::DataType. 00306 * \param resource_type M2MResourceInstance::ResourceType. 00307 * \return M2MBase::DataType. 00308 */ 00309 M2MBase::DataType convert_resource_type(M2MResourceInstance::ResourceType); 00310 00311 private: 00312 00313 M2MObject &_parent; 00314 00315 M2MResourceList _resource_list; // owned 00316 00317 friend class Test_M2MObjectInstance; 00318 friend class Test_M2MObject; 00319 friend class Test_M2MDevice; 00320 friend class Test_M2MSecurity; 00321 friend class Test_M2MServer; 00322 friend class Test_M2MNsdlInterface; 00323 friend class Test_M2MFirmware; 00324 friend class Test_M2MTLVSerializer; 00325 friend class Test_M2MTLVDeserializer; 00326 friend class Test_M2MBase; 00327 friend class Test_M2MResource; 00328 friend class Test_M2MResourceInstance; 00329 friend class TestFactory; 00330 }; 00331 00332 inline M2MObject& M2MObjectInstance::get_parent_object() const 00333 { 00334 return _parent; 00335 } 00336 00337 #endif // M2M_OBJECT_INSTANCE_H
Generated on Tue Jul 12 2022 19:12:13 by
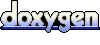