
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mnsdlobserver.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_NSDL_OBSERVER_H 00017 #define M2M_NSDL_OBSERVER_H 00018 00019 #include "include/nsdllinker.h" 00020 00021 //FORWARD DECLARATION 00022 class M2MSecurity; 00023 class M2MServer; 00024 00025 /** 00026 * @brief Observer class for informing NSDL callback to the state machine 00027 */ 00028 00029 class M2MNsdlObserver 00030 { 00031 00032 public : 00033 00034 /** 00035 * @brief Informs that coap message is ready. 00036 * @param data_ptr, Data object of coap message. 00037 * @param data_len, Length of the data object. 00038 * @param address_ptr, Address structure of the server. 00039 */ 00040 virtual void coap_message_ready(uint8_t *data_ptr, 00041 uint16_t data_len, 00042 sn_nsdl_addr_s *address_ptr) = 0; 00043 00044 /** 00045 * @brief Informs that client is registered successfully. 00046 * @param server_object, Server object associated with 00047 * registered server. 00048 */ 00049 virtual void client_registered(M2MServer *server_object) = 0; 00050 00051 /** 00052 * @brief Informs that client registration is updated successfully. 00053 * @param server_object, Server object associated with 00054 * registered server. 00055 */ 00056 virtual void registration_updated(const M2MServer &server_object) = 0; 00057 00058 /** 00059 * @brief Informs that some error occured during 00060 * registration. 00061 * @param error_code, Error code for registration error 00062 * @param retry, Indicates state machine to re-establish connection 00063 */ 00064 virtual void registration_error(uint8_t error_code, bool retry = false) = 0; 00065 00066 /** 00067 * @brief Informs that client is unregistered successfully. 00068 */ 00069 virtual void client_unregistered() = 0; 00070 00071 /** 00072 * @brief Informs that client bootstrapping is done. 00073 * @param security_object, M2MSecurity Object which contains information about 00074 * LWM2M server fetched from bootstrap server. 00075 */ 00076 virtual void bootstrap_done() = 0; 00077 00078 /** 00079 * @brief Informs that client bootstrap data has been received and final bootstrap 00080 * finish message has been handled. 00081 */ 00082 virtual void bootstrap_finish() = 0; 00083 00084 /** 00085 * @brief Informs that client bootstrapping is waiting for message to be sent. 00086 * @param security_object, M2MSecurity Object which contains information about 00087 * LWM2M server fetched from bootstrap server. 00088 */ 00089 virtual void bootstrap_wait() = 0; 00090 00091 /** 00092 * @brief Informs that client bootstrapping is waiting for error message to be sent. 00093 * @param reason, Error description. 00094 */ 00095 virtual void bootstrap_error_wait(const char *reason) = 0; 00096 00097 /** 00098 * @brief Informs that some error occured during 00099 * bootstrapping. 00100 * @param reason, Error string explaining the failure reason 00101 */ 00102 virtual void bootstrap_error(const char *reason) = 0; 00103 00104 /** 00105 * @brief Informs that received data has been processed. 00106 */ 00107 virtual void coap_data_processed() = 0; 00108 00109 /** 00110 * @brief Callback informing that the value of the resource object is updated by server. 00111 * @param base Object whose value is updated. 00112 */ 00113 virtual void value_updated(M2MBase *base) = 0; 00114 }; 00115 #endif // M2M_NSDL_OBSERVER_H
Generated on Tue Jul 12 2022 19:12:13 by
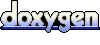