
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2minterfacefactory.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_INTERFACE_FACTORY_H 00017 #define M2M_INTERFACE_FACTORY_H 00018 00019 #include <stdlib.h> 00020 #include "mbed-client/m2msecurity.h" 00021 #include "mbed-client/m2mresource.h" 00022 #include "mbed-client/m2minterfaceobserver.h" 00023 00024 //FORWARD DECLARATION 00025 class M2MDevice; 00026 class M2MServer; 00027 class M2MInterfaceImpl; 00028 class M2MFirmware; 00029 00030 /*! \file m2minterfacefactory.h 00031 * \brief M2MInterfaceFactory. 00032 * This is a factory class that provides an interface for creating an mbed Client Interface 00033 * object for an application to utilize the LWM2M features provided by the client. 00034 */ 00035 00036 class M2MInterfaceFactory { 00037 private: 00038 // Prevents the use of an assignment operator by accident. 00039 M2MInterfaceFactory& operator=( const M2MInterfaceFactory& /*other*/ ); 00040 00041 // Prevents the use of a copy constructor by accident. 00042 M2MInterfaceFactory( const M2MInterfaceFactory& /*other*/ ); 00043 00044 public: 00045 00046 /** 00047 * \brief Creates an interface object for the mbed Client Inteface. With this, the 00048 * client can handle client operations like Bootstrapping, Client 00049 * Registration, Device Management and Information Reporting. 00050 * \param endpoint_name The endpoint name of mbed Client. 00051 * \param endpoint_type The endpoint type of mbed Client, default is empty. 00052 * \param life_time The lifetime of the endpoint in seconds, 00053 * if -1 it is optional. 00054 * \param listen_port The listening port for the endpoint, default is 5683. 00055 * \param domain The domain of the endpoint, default is empty. 00056 * \param mode The binding mode of the endpoint, default is NOT_SET. 00057 * \param stack The underlying network stack to be used for the connection, 00058 * default is LwIP_IPv4. 00059 * \param context_address The context address for M2M-HTTP, not used currently. 00060 * \return M2MInterfaceImpl An object for managing other client operations. 00061 */ 00062 static M2MInterface *create_interface(M2MInterfaceObserver &observer, 00063 const String &endpoint_name, 00064 const String &endpoint_type = "", 00065 const int32_t life_time = -1, 00066 const uint16_t listen_port = 5683, 00067 const String &domain = "", 00068 M2MInterface::BindingMode mode = M2MInterface::NOT_SET, 00069 M2MInterface::NetworkStack stack = M2MInterface::LwIP_IPv4, 00070 const String &context_address = ""); 00071 00072 /** 00073 * \brief Creates a security object for the mbed Client Inteface. With this, the 00074 * client can manage Bootstrapping and Client Registration. 00075 * \param ServerType The type of the Security Object, bootstrap or LWM2M server. 00076 * \return M2MSecurity An object for managing other client operations. 00077 */ 00078 static M2MSecurity *create_security(M2MSecurity::ServerType server_type); 00079 00080 /** 00081 * \brief Creates a server object for the mbed Client Inteface. With this, the 00082 * client can manage the server resources used for client operations 00083 * such as Client Registration, server lifetime. 00084 * \return M2MServer An object for managing server client operations. 00085 */ 00086 static M2MServer *create_server(); 00087 00088 /** 00089 * \brief Creates a device object for the mbed Client Inteface. With this, the 00090 * client can manage the device resources used for client operations 00091 * such as Client Registration, Device Management and Information Reporting. 00092 * \param name The name of the device object. 00093 * \return M2MDevice An object for managing other client operations. 00094 */ 00095 static M2MDevice *create_device(); 00096 00097 /** 00098 * \brief Creates a firmware object for the mbed Client Inteface. With this, the 00099 * client can manage the firmware resources used for the client operations 00100 * such as Client Registration, Device Management and Information Reporting. 00101 * \return M2MFirmware An object for managing other client operations. 00102 */ 00103 static M2MFirmware *create_firmware(); 00104 00105 /** 00106 * \brief Creates a generic object for the mbed Client Inteface. With this, the 00107 * client can manage its own customized resources used for registering 00108 * Device Management and Information Reporting for those resources. 00109 * \param name The name of the object. 00110 * \return M2MObject An object for managing other mbed Client operations. 00111 */ 00112 static M2MObject *create_object(const String &name); 00113 00114 #ifdef MBED_CLOUD_CLIENT_EDGE_EXTENSION 00115 /** 00116 * \brief Creates a endpoint object for the mbed Client Inteface. With this, the 00117 * client can manage multiple endpoints and their resources. Common directory path "d/" 00118 * will be prepended to the endpoint path, resulting in the endpoint having final path of 00119 * "d/name". 00120 * \param name The name of the object. 00121 * \return M2MObject An object for managing other mbed Client operations. 00122 */ 00123 static M2MEndpoint* create_endpoint(const String &name); 00124 #endif 00125 00126 friend class Test_M2MInterfaceFactory; 00127 }; 00128 00129 #endif // M2M_INTERFACE_FACTORY_H
Generated on Tue Jul 12 2022 19:12:13 by
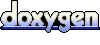