
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mdevice.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef M2M_DEVICE_H 00017 #define M2M_DEVICE_H 00018 00019 #include "mbed-client/m2mobject.h" 00020 00021 // FORWARD DECLARATION 00022 class M2MResource; 00023 class M2MResourceInstance; 00024 00025 /*! \file m2mdevice.h 00026 * \brief M2MDevice. 00027 * This class represents the Device Object model of the LWM2M framework. 00028 * It provides an interface for handling the device object 00029 * and all its corresponding resources. There can be only one instance 00030 * of a Device Object. 00031 */ 00032 class M2MDevice : public M2MObject { 00033 00034 friend class M2MInterfaceFactory; 00035 00036 public: 00037 00038 /** 00039 * \brief An enum defining all the resources associated with the 00040 * Device Object in the LWM2M framework. 00041 */ 00042 typedef enum { 00043 Manufacturer, 00044 DeviceType, 00045 ModelNumber, 00046 SerialNumber, 00047 HardwareVersion, 00048 FirmwareVersion, 00049 SoftwareVersion, 00050 Reboot, 00051 FactoryReset, 00052 AvailablePowerSources, 00053 PowerSourceVoltage, 00054 PowerSourceCurrent, 00055 BatteryLevel, 00056 BatteryStatus, 00057 MemoryFree, 00058 MemoryTotal, 00059 ErrorCode, 00060 ResetErrorCode, 00061 CurrentTime, 00062 UTCOffset, 00063 Timezone, 00064 SupportedBindingMode 00065 }DeviceResource; 00066 00067 private: 00068 00069 /** 00070 * Constructor 00071 */ 00072 M2MDevice(char *path); 00073 00074 // Prevents the use of assignment operator. 00075 M2MDevice& operator=( const M2MDevice& /*other*/ ); 00076 00077 // Prevents the use of copy constructor 00078 M2MDevice( const M2MDevice& /*other*/ ); 00079 00080 /** 00081 * Destructor 00082 */ 00083 virtual ~M2MDevice(); 00084 00085 static M2MDevice* get_instance(); 00086 00087 public: 00088 00089 /** 00090 * \brief Deletes an M2MDevice instance. 00091 */ 00092 static void delete_instance(); 00093 00094 /** 00095 * \brief Creates a new resource for the given resource enum. 00096 * \param resource With this function, the following resources can be created: 00097 * 'Manufacturer', 'DeviceType','ModelNumber','SerialNumber', 00098 * 'HardwareVersion', 'FirmwareVersion', 'SoftwareVersion', 00099 * 'UTCOffset', 'Timezone', 'SupportedBindingMode'. 00100 * \param value The value to be set on the resource, in string format. 00101 * \return M2MResource if created successfully, else NULL. 00102 */ 00103 M2MResource* create_resource(DeviceResource resource, const String &value); 00104 00105 /** 00106 * \brief Creates a new resource for given resource enum. 00107 * \param resource With this function, the following resources can be created: 00108 * 'AvailablePowerSources','PowerSourceVoltage','PowerSourceCurrent', 00109 * 'BatteryLevel', 'BatteryStatus', 'MemoryFree', 'MemoryTotal', 00110 * 'ErrorCode', 'CurrentTime'. For 'CurrentTime', pass the time value in EPOCH format, for example 00111 * 1438944683. 00112 * \param value The value to be set on the resource, in integer format. 00113 * \return M2MResource if created successfully, else NULL. 00114 */ 00115 M2MResource* create_resource(DeviceResource resource, int64_t value); 00116 00117 /** 00118 * \brief Creates a new resource instance for given resource enum. 00119 * \param resource With this function, the following resources can be created: 00120 * 'AvailablePowerSources','PowerSourceVoltage','PowerSourceCurrent', 00121 * 'ErrorCode'. 00122 * \param value The value to be set on the resource, in integer format. 00123 * \return M2MResourceInstance if created successfully, else NULL. 00124 */ 00125 M2MResourceInstance* create_resource_instance(DeviceResource resource, int64_t value, 00126 uint16_t instance_id); 00127 00128 /** 00129 * \brief Creates a new resource for given resource name. 00130 * \param resource With this function, the following resources can be created: 00131 * 'ResetErrorCode','FactoryReset'. 00132 * \return M2MResource if created successfully, else NULL. 00133 */ 00134 M2MResource* create_resource(DeviceResource resource); 00135 00136 /** 00137 * \brief Deletes the resource with the given resource enum. 00138 * Mandatory resources cannot be deleted. 00139 * \param resource The name of the resource to be deleted. 00140 * \return True if deleted, else false. 00141 */ 00142 bool delete_resource(DeviceResource resource); 00143 00144 /** 00145 * \brief Deletes the resource with the given resource enum. 00146 * Mandatory resources cannot be deleted. 00147 * \param resource The name of the resource to be deleted. 00148 * \param instance_id The instance ID of the resource. 00149 * \return True if deleted, else false. 00150 */ 00151 bool delete_resource_instance(DeviceResource resource, 00152 uint16_t instance_id); 00153 00154 /** 00155 * \brief Sets the value of the given resource enum. 00156 * \param resource With this function, a value can be set for the following resources: 00157 * 'Manufacturer', 'DeviceType','ModelNumber','SerialNumber', 00158 * 'HardwareVersion', 'FirmwareVersion', 'SoftwareVersion', 00159 * 'UTCOffset', 'Timezone', 'SupportedBindingMode'. 00160 * \param value The value to be set on the resource, in string format. 00161 * \param instance_id The instance ID of the resource, default is 0. 00162 * \return True if successfully set, else false. 00163 */ 00164 bool set_resource_value(DeviceResource resource, 00165 const String &value, 00166 uint16_t instance_id = 0); 00167 00168 /** 00169 * \brief Sets the value of the given resource enum. 00170 * \param resource With this function, a value can be set for the following resources: 00171 * 'AvailablePowerSources','PowerSourceVoltage','PowerSourceCurrent', 00172 * 'BatteryLevel', 'BatteryStatus', 'MemoryFree', 'MemoryTotal', 00173 * 'ErrorCode', 'CurrentTime'. 00174 * \param value The value to be set on the resource, in integer format. 00175 * \param instance_id The instance ID of the resource, default is 0. 00176 * \return True if successfully set, else false. 00177 */ 00178 bool set_resource_value(DeviceResource resource, 00179 int64_t value, 00180 uint16_t instance_id = 0); 00181 00182 /** 00183 * \brief Returns the value of the given resource enum, in string format. 00184 * \param resource With this function, the following resources can return a value: 00185 * 'Manufacturer', 'DeviceType','ModelNumber','SerialNumber', 00186 * 'HardwareVersion', 'FirmwareVersion', 'SoftwareVersion', 00187 * 'UTCOffset', 'Timezone', 'SupportedBindingMode'. 00188 * \param instance_id The instance ID of the resource, default is 0. 00189 * \return The value associated with that resource. If the resource is not valid NULL is returned. 00190 */ 00191 String resource_value_string(DeviceResource resource, 00192 uint16_t instance_id = 0) const; 00193 00194 /** 00195 * \brief Returns the value of the given resource key name, in integer format. 00196 * \param resource With this function, the following resources can return a value: 00197 * 'AvailablePowerSources','PowerSourceVoltage','PowerSourceCurrent', 00198 * 'BatteryLevel', 'BatteryStatus', 'MemoryFree', 'MemoryTotal', 00199 * 'ErrorCode', 'CurrentTime'. 00200 * \param instance_id The instance ID of the resource, default is 0 00201 * \return The value associated with that resource. If the resource is not valid -1 is returned. 00202 */ 00203 int64_t resource_value_int(DeviceResource resource, 00204 uint16_t instance_id = 0) const; 00205 00206 /** 00207 * \brief Indicates whether the resource instance with the given resource enum exists or not. 00208 * \param resource The resource enum. 00209 * \return True if at least one instance exists, else false. 00210 */ 00211 bool is_resource_present(DeviceResource resource)const; 00212 00213 /** 00214 * \brief Returns the number of resources for the whole device object. 00215 * \return Total number of resources belonging to the device object. 00216 */ 00217 uint16_t total_resource_count()const; 00218 00219 /** 00220 * \brief Returns the number of resources for a given resource enum. 00221 * \param resource The resource enum. 00222 * \return The number of resources for a given resource enum. Returns 1 for the 00223 * mandatory resources. Can be 0 as well if no instances exist for an 00224 * optional resource. 00225 */ 00226 uint16_t per_resource_count(DeviceResource resource)const; 00227 00228 private: 00229 00230 M2MResourceBase* get_resource_instance(DeviceResource dev_res, 00231 uint16_t instance_id) const; 00232 00233 static const char* resource_name(DeviceResource resource); 00234 00235 static bool check_value_range(DeviceResource resource, const int64_t value); 00236 00237 private : 00238 00239 M2MObjectInstance* _device_instance; //Not owned 00240 00241 protected: 00242 00243 static M2MDevice* _instance; 00244 00245 friend class Test_M2MDevice; 00246 friend class Test_M2MInterfaceFactory; 00247 }; 00248 00249 #endif // M2M_DEVICE_H 00250
Generated on Tue Jul 12 2022 19:12:12 by
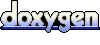