
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mconnectionhandler.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed-client-classic/m2mconnectionhandlerpimpl.h" 00017 #include "mbed-client/m2mconnectionobserver.h" 00018 #include "mbed-client/m2mconnectionhandler.h" 00019 #include "mbed-client/m2mconstants.h" 00020 00021 M2MConnectionHandler::M2MConnectionHandler(M2MConnectionObserver &observer, 00022 M2MConnectionSecurity* sec, 00023 M2MInterface::BindingMode mode, 00024 M2MInterface::NetworkStack stack) 00025 :_observer(observer) 00026 { 00027 _private_impl = new M2MConnectionHandlerPimpl(this, observer, sec, mode, stack); 00028 } 00029 00030 M2MConnectionHandler::~M2MConnectionHandler() 00031 { 00032 delete _private_impl; 00033 } 00034 00035 bool M2MConnectionHandler::bind_connection(const uint16_t listen_port) 00036 { 00037 00038 return _private_impl->bind_connection(listen_port); 00039 } 00040 00041 bool M2MConnectionHandler::resolve_server_address(const String& server_address, 00042 const uint16_t server_port, 00043 M2MConnectionObserver::ServerType server_type, 00044 const M2MSecurity* security) 00045 { 00046 return _private_impl->resolve_server_address(server_address, server_port, 00047 server_type, security); 00048 } 00049 00050 bool M2MConnectionHandler::start_listening_for_data() 00051 { 00052 return _private_impl->start_listening_for_data(); 00053 } 00054 00055 void M2MConnectionHandler::stop_listening() 00056 { 00057 _private_impl->stop_listening(); 00058 } 00059 00060 bool M2MConnectionHandler::send_data(uint8_t *data, 00061 uint16_t data_len, 00062 sn_nsdl_addr_s *address) 00063 { 00064 return _private_impl->send_data(data, data_len, address); 00065 } 00066 00067 void M2MConnectionHandler::handle_connection_error(int error) 00068 { 00069 _private_impl->handle_connection_error(error); 00070 } 00071 00072 void M2MConnectionHandler::set_platform_network_handler(void *handler) 00073 { 00074 _private_impl->set_platform_network_handler(handler); 00075 } 00076 00077 void M2MConnectionHandler::claim_mutex() 00078 { 00079 _private_impl->claim_mutex(); 00080 } 00081 00082 void M2MConnectionHandler::release_mutex() 00083 { 00084 _private_impl->release_mutex(); 00085 } 00086 00087 void M2MConnectionHandler::force_close() 00088 { 00089 _private_impl->force_close(); 00090 }
Generated on Tue Jul 12 2022 19:12:12 by
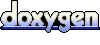