
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
m2mcallbackstorage.cpp
00001 /* 00002 * Copyright (c) 2017 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "include/m2mcallbackstorage.h" 00018 00019 #include <cstddef> 00020 00021 // Dummy constructor, which does not init any value to something meaningful but needed for array construction. 00022 // It is better to leave values unintialized, so the Valgrind will point out if the Vector is used without 00023 // setting real values in there. 00024 M2MCallbackAssociation::M2MCallbackAssociation() 00025 { 00026 } 00027 00028 M2MCallbackAssociation::M2MCallbackAssociation(const M2MBase *object, void *callback, M2MCallbackType type, void *client_args) 00029 : _object(object), _callback(callback), _type(type), _client_args(client_args) 00030 { 00031 } 00032 00033 M2MCallbackStorage* M2MCallbackStorage::_static_instance = NULL; 00034 00035 M2MCallbackStorage *M2MCallbackStorage::get_instance() 00036 { 00037 if (M2MCallbackStorage::_static_instance == NULL) { 00038 M2MCallbackStorage::_static_instance = new M2MCallbackStorage(); 00039 } 00040 return M2MCallbackStorage::_static_instance; 00041 } 00042 00043 void M2MCallbackStorage::delete_instance() 00044 { 00045 delete M2MCallbackStorage::_static_instance; 00046 M2MCallbackStorage::_static_instance = NULL; 00047 } 00048 00049 M2MCallbackStorage::~M2MCallbackStorage() 00050 { 00051 // TODO: go through the list and delete all the FP<n> objects if there are any. 00052 // On the other hand, if the system is done properly, each m2mobject should actually 00053 // remove its callbacks from its destructor so there is nothing here to do 00054 } 00055 00056 bool M2MCallbackStorage::add_callback(const M2MBase &object, 00057 void *callback, 00058 M2MCallbackAssociation::M2MCallbackType type, 00059 void *client_args) 00060 { 00061 bool add_success = false; 00062 M2MCallbackStorage* instance = get_instance(); 00063 if (instance) { 00064 00065 add_success = instance->do_add_callback(object, callback, type, client_args); 00066 } 00067 return add_success; 00068 } 00069 00070 bool M2MCallbackStorage::do_add_callback(const M2MBase &object, void *callback, M2MCallbackAssociation::M2MCallbackType type, void *client_args) 00071 { 00072 bool add_success = false; 00073 00074 // verify that the same callback is not re-added. 00075 if (does_callback_exist(object, callback, type) == false) { 00076 00077 const M2MCallbackAssociation association(&object, callback, type, client_args); 00078 _callbacks.push_back(association); 00079 add_success = true; 00080 } 00081 00082 return add_success; 00083 } 00084 00085 #if 0 // Functions not used currently 00086 void M2MCallbackStorage::remove_callbacks(const M2MBase &object) 00087 { 00088 // do not use the get_instance() here as it might create the instance 00089 M2MCallbackStorage* instance = M2MCallbackStorage::_static_instance; 00090 if (instance) { 00091 00092 instance->do_remove_callbacks(object); 00093 } 00094 } 00095 00096 void M2MCallbackStorage::do_remove_callbacks(const M2MBase &object) 00097 { 00098 // find any association to given object and delete them from the vector 00099 for (int index = 0; index < _callbacks.size();) { 00100 if (_callbacks[index]._object == &object) { 00101 _callbacks.erase(index); 00102 } else { 00103 index++; 00104 } 00105 } 00106 } 00107 #endif 00108 00109 void* M2MCallbackStorage::remove_callback(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) 00110 { 00111 void* callback = NULL; 00112 00113 // do not use the get_instance() here as it might create the instance 00114 M2MCallbackStorage* instance = M2MCallbackStorage::_static_instance; 00115 if (instance) { 00116 00117 callback = instance->do_remove_callback(object, type); 00118 } 00119 return callback; 00120 } 00121 00122 void* M2MCallbackStorage::do_remove_callback(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) 00123 { 00124 void* callback = NULL; 00125 for (int index = 0; index < _callbacks.size(); index++) { 00126 00127 if ((_callbacks[index]._object == &object) && (_callbacks[index]._type == type)) { 00128 callback = _callbacks[index]._callback; 00129 _callbacks.erase(index); 00130 break; 00131 } 00132 } 00133 return callback; 00134 } 00135 00136 void* M2MCallbackStorage::get_callback(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) 00137 { 00138 void* callback = NULL; 00139 const M2MCallbackStorage* instance = get_instance(); 00140 if (instance) { 00141 00142 callback = instance->do_get_callback(object, type); 00143 } 00144 return callback; 00145 } 00146 00147 void* M2MCallbackStorage::do_get_callback(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) const 00148 { 00149 void* callback = NULL; 00150 if (!_callbacks.empty()) { 00151 M2MCallbackAssociationList::const_iterator it = _callbacks.begin(); 00152 00153 for ( ; it != _callbacks.end(); it++ ) { 00154 00155 if ((it->_object == &object) && (it->_type == type)) { 00156 callback = it->_callback; 00157 break; 00158 } 00159 } 00160 } 00161 return callback; 00162 } 00163 00164 M2MCallbackAssociation* M2MCallbackStorage::get_association_item(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) 00165 { 00166 M2MCallbackAssociation* callback_association = NULL; 00167 const M2MCallbackStorage* instance = get_instance(); 00168 if (instance) { 00169 00170 callback_association = instance->do_get_association_item(object, type); 00171 } 00172 return callback_association; 00173 } 00174 00175 M2MCallbackAssociation* M2MCallbackStorage::do_get_association_item(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) const 00176 { 00177 M2MCallbackAssociation* callback_association = NULL; 00178 if (!_callbacks.empty()) { 00179 M2MCallbackAssociationList::const_iterator it = _callbacks.begin(); 00180 00181 for ( ; it != _callbacks.end(); it++ ) { 00182 00183 if ((it->_object == &object) && (it->_type == type)) { 00184 callback_association = (M2MCallbackAssociation*)it; 00185 break; 00186 } 00187 } 00188 } 00189 return callback_association; 00190 } 00191 00192 bool M2MCallbackStorage::does_callback_exist(const M2MBase &object, M2MCallbackAssociation::M2MCallbackType type) 00193 { 00194 bool found = false; 00195 if (get_callback(object, type) != NULL) { 00196 found = true; 00197 } 00198 return found; 00199 } 00200 00201 bool M2MCallbackStorage::does_callback_exist(const M2MBase &object, void *callback, M2MCallbackAssociation::M2MCallbackType type) const 00202 { 00203 bool match_found = false; 00204 00205 if (!_callbacks.empty()) { 00206 M2MCallbackAssociationList::const_iterator it = _callbacks.begin(); 00207 00208 for ( ; it != _callbacks.end(); it++ ) { 00209 00210 if ((it->_object == &object) && (it->_callback == callback) && (it->_type == type)) { 00211 match_found = true; 00212 break; 00213 } 00214 } 00215 } 00216 00217 return match_found; 00218 }
Generated on Tue Jul 12 2022 19:12:12 by
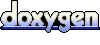