
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
key_config_manager.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __KEYS_CONFIG_MANAGER_H__ 00018 #define __KEYS_CONFIG_MANAGER_H__ 00019 00020 #include <stdlib.h> 00021 #include <stdbool.h> 00022 #include <inttypes.h> 00023 #include "kcm_status.h" 00024 #include "kcm_defs.h" 00025 00026 #ifdef __cplusplus 00027 extern "C" { 00028 #endif 00029 00030 /** 00031 * @file key_config_manager.h 00032 * \brief Keys and Configuration Manager (KCM) APIs. 00033 */ 00034 00035 /* === Initialization and Finalization === */ 00036 00037 /** 00038 * Initiate the KCM module. 00039 * Allocates and initializes file storage resources. 00040 * 00041 * @returns 00042 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00043 */ 00044 kcm_status_e kcm_init(void); 00045 00046 /** 00047 * Finalize the KCM module. 00048 * Finalizes and frees file storage resources. 00049 * 00050 * @returns 00051 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00052 */ 00053 kcm_status_e kcm_finalize(void); 00054 00055 /* === Keys, Certificates and Configuration data storage === */ 00056 00057 /** Store the KCM item into a secure storage. 00058 * 00059 * @param[in] kcm_item_name KCM item name. 00060 * @param[in] kcm_item_name_len KCM item name length. 00061 * @param[in] kcm_item_type KCM item type as defined in `::kcm_item_type_e` 00062 * @param[in] kcm_item_is_factory True if the KCM item is a factory item, otherwise false. 00063 * @param[in] kcm_item_data KCM item data buffer. Can be NULL if `kcm_item_data_size` is 0. 00064 * @param[in] kcm_item_data_size KCM item data buffer size in bytes. Can be 0 if you wish to store an empty file. 00065 * @param[in] security_desc Security descriptor. 00066 * 00067 * @returns 00068 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00069 */ 00070 kcm_status_e kcm_item_store(const uint8_t *kcm_item_name, size_t kcm_item_name_len, kcm_item_type_e kcm_item_type, bool kcm_item_is_factory, const uint8_t *kcm_item_data, size_t kcm_item_data_size, const kcm_security_desc_s security_desc); 00071 00072 /* === Keys, Certificates and Configuration data retrieval === */ 00073 00074 /** Retrieve the KCM item data size from a secure storage. 00075 * 00076 * @param[in] kcm_item_name KCM item name. 00077 * @param[in] kcm_item_name_len KCM item name length. 00078 * @param[in] kcm_item_type KCM item type as defined in `::kcm_item_type_e` 00079 * @param[out] kcm_item_data_size_out KCM item data size in bytes. 00080 * 00081 * @returns 00082 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00083 */ 00084 kcm_status_e kcm_item_get_data_size(const uint8_t *kcm_item_name, size_t kcm_item_name_len, kcm_item_type_e kcm_item_type, size_t *kcm_item_data_size_out); 00085 00086 /** Retrieve KCM item data from a secure storage. 00087 * 00088 * @param[in] kcm_item_name KCM item name. 00089 * @param[in] kcm_item_name_len KCM item name length. 00090 * @param[in] kcm_item_type KCM item type as defined in `::kcm_item_type_e` 00091 * @param[out] kcm_item_data_out KCM item data output buffer. Can be NULL if `kcm_item_data_size` is 0. 00092 * @param[in] kcm_item_data_max_size The maximum size of the KCM item data output buffer in bytes. 00093 * @param[out] kcm_item_data_act_size_out Actual KCM item data output buffer size in bytes. 00094 * 00095 * @returns 00096 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00097 */ 00098 kcm_status_e kcm_item_get_data(const uint8_t *kcm_item_name, size_t kcm_item_name_len, kcm_item_type_e kcm_item_type, uint8_t *kcm_item_data_out, size_t kcm_item_data_max_size, size_t * kcm_item_data_act_size_out); 00099 00100 /* === Keys, Certificates and Configuration delete === */ 00101 00102 /** Delete a KCM item from a secure storage. 00103 * 00104 * @param[in] kcm_item_name KCM item name. 00105 * @param[in] kcm_item_name_len KCM item name length. 00106 * @param[in] kcm_item_type KCM item type as defined in `::kcm_item_type_e` 00107 * 00108 * @returns 00109 * KCM_STATUS_SUCCESS status in case of success or one of ::kcm_status_e errors otherwise. 00110 */ 00111 kcm_status_e kcm_item_delete(const uint8_t *kcm_item_name, size_t kcm_item_name_len, kcm_item_type_e kcm_item_type); 00112 00113 /* === Certificates chain APIs === */ 00114 00115 /** The API initializes chain context for write chain operation, 00116 * This API should be called prior to ::kcm_cert_chain_add_next API. 00117 * 00118 * @param[out] kcm_chain_handle pointer to certificate chain handle. 00119 * @param[in] kcm_chain_name pointer to certificate chain name. 00120 * @param[in] kcm_chain_name_len length of certificate name buffer. 00121 * @param[in] kcm_chain_len number of certificates in the chain. 00122 * @param[in] kcm_chain_is_factory True if the KCM chain is a factory item, otherwise false. 00123 * 00124 * @returns 00125 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00126 */ 00127 kcm_status_e kcm_cert_chain_create(kcm_cert_chain_handle *kcm_chain_handle, 00128 const uint8_t *kcm_chain_name, 00129 size_t kcm_chain_name_len, 00130 size_t kcm_chain_len, 00131 bool kcm_chain_is_factory); 00132 00133 /** The API initializes chain context for read chain operation. 00134 * This API should be called prior to ::kcm_cert_chain_get_next_size and ::kcm_cert_chain_get_next_data APIs 00135 * 00136 * @param[out] kcm_chain_handle pointer to certificate chain handle. 00137 * @param[in] kcm_chain_name pointer to certificate chain name. 00138 * @param[in] kcm_chain_name_len size of certificate name buffer. 00139 * @param[out] kcm_chain_len length of certificate chain. 00140 * 00141 * @returns 00142 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00143 */ 00144 kcm_status_e kcm_cert_chain_open(kcm_cert_chain_handle *kcm_chain_handle, 00145 const uint8_t *kcm_chain_name, 00146 size_t kcm_chain_name_len, 00147 size_t *kcm_chain_len_out); 00148 00149 /** This API adds next certificate of chain to the storage. 00150 * 00151 * It also validates the previous certificate (unless it is the first certificate) with the public key from kcm_cert_data. 00152 * The certificates should be added in the order from lowest child, followed by the certificate that signs it and so on, all the way to the root of the chain. 00153 * 00154 * @param[in] kcm_chain_handle certificate chain handle. 00155 * @param[in] kcm_cert_data pointer to certificate data in DER format. 00156 * @param[in] kcm_cert_data_size size of certificate data buffer. 00157 * 00158 * @returns 00159 * KCM_STATUS_SUCCESS in case of success. 00160 * KCM_STATUS_CERTIFICATE_CHAIN_VERIFICATION_FAILED in case that one of the certificate in the chain failed to verify its predecessor 00161 * In other casese - one of the `::kcm_status_e` errors. 00162 * 00163 */ 00164 kcm_status_e kcm_cert_chain_add_next(kcm_cert_chain_handle kcm_chain_handle, 00165 const uint8_t *kcm_cert_data, 00166 size_t kcm_cert_data_size); 00167 00168 /** The API deletes all certificates of the chain from the storage. 00169 * 00170 * @param[in] kcm_chain_name pointer to certificate chain name. 00171 * @param[in] kcm_chain_name_len length of certificate chain name. 00172 * 00173 * @returns 00174 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00175 */ 00176 kcm_status_e kcm_cert_chain_delete(const uint8_t *kcm_chain_name, 00177 size_t kcm_chain_name_len); 00178 00179 /** The API returns size of the next certificate in the chain. 00180 * This API should be called prior to ::kcm_cert_chain_get_next_data. 00181 * This operation does not increase chain's context iterator. 00182 * 00183 * @param[in] kcm_chain_handle certificate chain handle. 00184 * @param[out] kcm_cert_data_size pointer size of next certificate. 00185 * 00186 * @returns 00187 * KCM_STATUS_SUCCESS in case of success. 00188 * KCM_STATUS_INVALID_NUM_OF_CERT_IN_CHAIN in case we reached the end of the chain 00189 * Otherwise one of the `::kcm_status_e` errors. 00190 */ 00191 kcm_status_e kcm_cert_chain_get_next_size(kcm_cert_chain_handle kcm_chain_handle, 00192 size_t *kcm_cert_data_size); 00193 00194 /** The API returns data of the next certificate in the chain. 00195 * To get exact size of a next certificate use ::kcm_cert_chain_get_next_size. 00196 * In the end of get data operation, chain context points to the next certificate of current chain. 00197 * 00198 * @param[in] kcm_chain_handle certificate chain handle. 00199 * @param[in/out] kcm_cert_data pointer to certificate data in DER format. 00200 * @param[in] kcm_max_cert_data_size max size of certificate data buffer. 00201 * @param[out] kcm_actual_cert_data_size actual size of certificate data. 00202 * 00203 * @returns 00204 * KCM_STATUS_SUCCESS in case of success. 00205 * KCM_STATUS_INVALID_NUM_OF_CERT_IN_CHAIN in case we reached the end of the chain 00206 * Otherwise one of the `::kcm_status_e` errors. 00207 */ 00208 kcm_status_e kcm_cert_chain_get_next_data(kcm_cert_chain_handle kcm_chain_handle, 00209 uint8_t *kcm_cert_data, 00210 size_t kcm_max_cert_data_size, 00211 size_t *kcm_actual_cert_data_size); 00212 00213 00214 /** The API releases the context and frees allocated resources. 00215 * When operation type is creation--> if total number of added/stored certificates is not equal to number 00216 * of certificates in the chain, the API will return an error. 00217 * 00218 * @param[in] kcm_chain_handle certificate chain handle. 00219 * 00220 * @returns 00221 * KCM_STATUS_SUCCESS in case of success. 00222 * KCM_STATUS_CLOSE_INCOMPLETE_CHAIN in case of not all certificates were saved. In this case the chain will be deleted. 00223 * Otherwise one of the `::kcm_status_e` errors. 00224 */ 00225 kcm_status_e kcm_cert_chain_close(kcm_cert_chain_handle kcm_chain_handle); 00226 00227 00228 /* === Factory Reset === */ 00229 00230 /** Reset the KCM secure storage to factory state. 00231 * 00232 * @returns 00233 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00234 */ 00235 kcm_status_e kcm_factory_reset(void); 00236 00237 00238 /** Generate a key pair complying the given cryptographic scheme in DER format. 00239 * Saves private key and public key if provided. 00240 * 00241 * @param key_scheme The cryptographic scheme. 00242 * @param private_key_name The private key name for which a key pair is generated. 00243 * @param private_key_name_len Private key name length 00244 * @param public_key_name The public key name for which a key pair is generated. 00245 * This parameter is optional. If not provided, the key will be generated, but not stored. 00246 * @param public_key_name_len Public key name length. 00247 * Must be 0, if ::public_key_name not provided. 00248 * @param kcm_item_is_factory True if the KCM item is a factory item, otherwise false. 00249 * @param kcm_params Additional kcm_params. Currently void. 00250 * 00251 * @returns 00252 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00253 */ 00254 kcm_status_e kcm_key_pair_generate_and_store( 00255 const kcm_crypto_key_scheme_e key_scheme, 00256 const uint8_t *private_key_name, 00257 size_t private_key_name_len, 00258 const uint8_t *public_key_name, 00259 size_t public_key_name_len, 00260 bool kcm_item_is_factory, 00261 const kcm_security_desc_s *kcm_params 00262 ); 00263 00264 00265 /** Generate a general CSR from the given private key. 00266 * 00267 * @param private_key_name The private key name to fetch from storage. 00268 * @param private_key_name_len The private key name len. 00269 * @param csr_params CSR parameters. 00270 * @param csr_buff_out Pointer to generated CSR buffer to fill. 00271 * @param csr_buff_max_size Size of the supplied CSR buffer. 00272 * @param csr_buff_act_size Actual size of the filled CSR buffer. 00273 * 00274 * @returns 00275 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00276 */ 00277 kcm_status_e kcm_csr_generate( 00278 const uint8_t *private_key_name, 00279 size_t private_key_name_len, 00280 const kcm_csr_params_s *csr_params, 00281 uint8_t *csr_buff_out, 00282 size_t csr_buff_max_size, 00283 size_t *csr_buff_act_size 00284 ); 00285 00286 00287 /** Generate private and public key and CSR from the generated keys. 00288 * 00289 * @param key_scheme The cryptographic scheme. 00290 * @param private_key_name The private key name to generate. 00291 * @param private_key_name_len The private key name len. 00292 * @param public_key_name The public key name for which a key pair is generated. 00293 * This parameter is optional. If not provided, the key will be generated, but not stored. 00294 * @param public_key_name_len Public key name length. 00295 * Must be 0, if ::public_key_name not provided. 00296 * @param kcm_item_is_factory True if the KCM item is a factory item, otherwise false. 00297 * @param csr_params CSR parameters. 00298 * @param csr_buff_out Pointer to generated CSR buffer to fill. 00299 * @param csr_buff_max_size Size of the supplied CSR buffer. 00300 * @param csr_buff_act_size Actual size of the filled CSR buffer. 00301 * @param kcm_data_pkcm_params Additional kcm_params. Currently void. 00302 * 00303 * @returns 00304 * KCM_STATUS_SUCCESS in case of success or one of the `::kcm_status_e` errors otherwise. 00305 */ 00306 kcm_status_e kcm_generate_keys_and_csr( 00307 kcm_crypto_key_scheme_e key_scheme, 00308 const uint8_t *private_key_name, 00309 size_t private_key_name_len, 00310 const uint8_t *public_key_name, 00311 size_t public_key_name_len, 00312 bool kcm_item_is_factory, 00313 const kcm_csr_params_s *csr_params, 00314 uint8_t *csr_buff_out, 00315 size_t csr_buff_max_size, 00316 size_t *csr_buff_act_size_out, 00317 const kcm_security_desc_s *kcm_params 00318 ); 00319 00320 /** Verify the device-generated certificate against given private key name from storage. 00321 * This function can be called when certificate creation is initiated by device using `kcm_generate_keys_and_csr` or `kcm_csr_generate` functions. 00322 * In this case, the function checks correlation between certificate's public key and given private key generated by the device and saved in device storage. 00323 * 00324 * @param[in] kcm_cert_data DER certificate data buffer. 00325 * @param[in] kcm_cert_data_size DER certificate data buffer size in bytes. 00326 * @param[in] kcm_priv_key_name Private key name of the certificate, the function assumes that the key was generated by the device and saved in the storage. 00327 * @param[in] kcm_priv_key_name_len Private key name length of the certificate. 00328 * 00329 * @returns 00330 * KCM_STATUS_SUCCESS in case of success. 00331 * KCM_STATUS_ITEM_NOT_FOUND in case private key wasn't found in the storage, 00332 * otherwise one of the `::kcm_status_e` errors. 00333 */ 00334 kcm_status_e kcm_certificate_verify_with_private_key( 00335 const uint8_t * kcm_cert_data, 00336 size_t kcm_cert_data_size, 00337 const uint8_t * kcm_priv_key_name, 00338 size_t kcm_priv_key_name_len); 00339 00340 00341 00342 #ifdef __cplusplus 00343 } 00344 #endif 00345 00346 #endif //__KEYS_CONFIG_MANAGER_H__ 00347
Generated on Tue Jul 12 2022 19:12:12 by
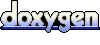