
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
kcm_status.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __KCM_STATUS_H__ 00018 #define __KCM_STATUS_H__ 00019 00020 #ifdef __cplusplus 00021 extern "C" { 00022 #endif 00023 00024 /** 00025 * @file kcm_status.h 00026 * \brief Keys and configuration manager (KCM) status/error codes. 00027 * This list may grow as needed. 00028 */ 00029 00030 typedef enum { 00031 KCM_STATUS_SUCCESS, //!< Operation completed successfully. 00032 KCM_STATUS_ERROR, //!< Operation ended with an unspecified error. 00033 KCM_STATUS_INVALID_PARAMETER, //!< A parameter provided to the function was invalid. 00034 KCM_STATUS_INSUFFICIENT_BUFFER, //!< The provided buffer size was insufficient for the required output. 00035 KCM_STATUS_OUT_OF_MEMORY, //!< An out-of-memory condition occurred. 00036 KCM_STATUS_ITEM_NOT_FOUND, //!< The item was not found in the storage. 00037 KCM_STATUS_META_DATA_NOT_FOUND, //!< The meta data was not found in the file. 00038 KCM_STATUS_META_DATA_SIZE_ERROR, //!< The meta data found but the size is different then expected. 00039 KCM_STATUS_FILE_EXIST, //!< Trying to store an item that is already in the storage. 00040 KCM_STATUS_KEY_EXIST, //!< Trying to generate a key for a CSR, but the requesested output key name already exists in the storage. 00041 KCM_STATUS_NOT_PERMITTED, //!< Trying to access an item without proper permissions. 00042 KCM_STATUS_STORAGE_ERROR, //!< File error occurred. 00043 KCM_STATUS_ITEM_IS_EMPTY, //!< The data of current item is empty. 00044 KCM_STATUS_INVALID_FILE_VERSION, //!< Invalid file version, the file can not be read 00045 KCM_STATUS_FILE_CORRUPTED, //!< File data corrupted, the file can not be read 00046 KCM_STATUS_FILE_NAME_CORRUPTED, //!< File name corrupted, the file can not be read 00047 KCM_STATUS_INVALID_FILE_ACCESS_MODE, //!< Invalid file access mode 00048 KCM_STATUS_UNKNOWN_STORAGE_ERROR, //!< KCM can not translate current storage error 00049 KCM_STATUS_NOT_INITIALIZED, //!< KCM did not initialized. 00050 KCM_STATUS_CLOSE_INCOMPLETE_CHAIN, //!< Closing KCM chain with less certificates than declared in create 00051 KCM_STATUS_CORRUPTED_CHAIN_FILE, //!< KCM attempted to open an invalid chain file 00052 KCM_STATUS_INVALID_NUM_OF_CERT_IN_CHAIN, //!< Operation failed due to invalid number of certificates. 00053 KCM_STATUS_CERTIFICATE_CHAIN_VERIFICATION_FAILED, //!< At least one of the certificates fails to verify its predecessor. 00054 KCM_STATUS_FILE_NAME_TOO_LONG, //!< Provided a file name that is longer than permitted. 00055 KCM_CRYPTO_STATUS_UNSUPPORTED_HASH_MODE, //!< Operation was called with unsupported hash mode. 00056 KCM_CRYPTO_STATUS_PARSING_DER_PRIVATE_KEY, //!< Operation failed to parse private der key. 00057 KCM_CRYPTO_STATUS_PARSING_DER_PUBLIC_KEY, //!< Operation failed to parse public der key. 00058 KCM_CRYPTO_STATUS_PK_KEY_INVALID_FORMAT, //!< Operation failed due to invalid pk key format. 00059 KCM_CRYPTO_STATUS_INVALID_PK_PUBKEY, //!< Operation failed due to invalid pk public key. 00060 KCM_CRYPTO_STATUS_ECP_INVALID_KEY, //!< Operation failed due to invalid ECP key. 00061 KCM_CRYPTO_STATUS_PK_KEY_INVALID_VERSION, //!< Operation failed due to invalid pk version of key. 00062 KCM_CRYPTO_STATUS_PK_PASSWORD_REQUIRED, //!< Operation failed due to missing password. 00063 KCM_CRYPTO_STATUS_PRIVATE_KEY_VERIFICATION_FAILED, //!< Operation failed to verify private key. 00064 KCM_CRYPTO_STATUS_PUBLIC_KEY_VERIFICATION_FAILED, //!< Operation failed to verify public key. 00065 KCM_CRYPTO_STATUS_PK_UNKNOWN_PK_ALG, //!< Operation failed due to unknown pk algorithm, 00066 KCM_CRYPTO_STATUS_UNSUPPORTED_CURVE, //!< Unsupported curve. 00067 KCM_CRYPTO_STATUS_PARSING_DER_CERT, //!< Operation failed to parse der certificate. 00068 KCM_CRYPTO_STATUS_CERT_EXPIRED, //!< Certificate validity is expired. 00069 KCM_CRYPTO_STATUS_CERT_FUTURE, //!< Certificate validity starts in future. 00070 KCM_CRYPTO_STATUS_CERT_MD_ALG, //!< Certificate with bad MD algorithm. 00071 KCM_CRYPTO_STATUS_CERT_PUB_KEY_TYPE, //!< Certificate with unsupported public key PK type. 00072 KCM_CRYPTO_STATUS_CERT_PUB_KEY, //!< Certificate with bad public key data (size or curve). 00073 KCM_CRYPTO_STATUS_CERT_NOT_TRUSTED, //!< Certificate is not trusted. 00074 KCM_CRYPTO_STATUS_INVALID_X509_ATTR, //!< Certificate with bad x509 attribute 00075 KCM_CRYPTO_STATUS_VERIFY_SIGNATURE_FAILED, //!< Operation failed to check the signature. 00076 KCM_CRYPTO_STATUS_INVALID_MD_TYPE, //!< Operation failed in check of ecc md type. 00077 KCM_CRYPTO_STATUS_FAILED_TO_WRITE_SIGNATURE, //!< Operation failed to calculate signature. 00078 KCM_CRYPTO_STATUS_FAILED_TO_WRITE_PRIVATE_KEY, //!< Operation failed to write private key to DER buffer. 00079 KCM_CRYPTO_STATUS_FAILED_TO_WRITE_PUBLIC_KEY, //!< Operation failed to write public key to DER buffer. 00080 KCM_CRYPTO_STATUS_FAILED_TO_WRITE_CSR, //!< Operation failed to write CSR to DER buffer. 00081 KCM_CRYPTO_STATUS_INVALID_OID, //!< Operation failed due to invalid OID. 00082 KCM_CRYPTO_STATUS_INVALID_NAME_FORMAT, //!< Operation failed due to invalid name format. 00083 KCM_STATUS_SELF_GENERATED_CERTIFICATE_VERIFICATION_ERROR, //!< Verification of self-generated certificate against stored private key failed 00084 KCM_MAX_STATUS, 00085 } kcm_status_e ; 00086 00087 //The macro defined for backward compatibility. Will be deprecated. 00088 #define KCM_STATUS_ESFS_ERROR KCM_STATUS_STORAGE_ERROR 00089 00090 #ifdef __cplusplus 00091 } 00092 #endif 00093 00094 #endif //__KCM_STATUS_H__
Generated on Tue Jul 12 2022 19:12:12 by
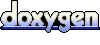