
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
kcm_internal.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef KEYS_CONFIG_MANAGER_INTERNAL_H 00018 #define KEYS_CONFIG_MANAGER_INTERNAL_H 00019 00020 #include <stdlib.h> 00021 #include <stdbool.h> 00022 #include <inttypes.h> 00023 #include "esfs.h" 00024 #include "cs_hash.h" 00025 00026 #ifdef __cplusplus 00027 extern "C" { 00028 #endif 00029 00030 /* === Definitions and Prototypes === */ 00031 00032 /* === Defines === */ 00033 #define FCC_ENTROPY_SIZE 48 00034 #define FCC_ROT_SIZE 16 00035 #define FCC_CA_IDENTIFICATION_SIZE 33 //PAL_CERT_ID_SIZE 00036 00037 /* === EC max sizes === */ 00038 #define KCM_EC_SECP256R1_MAX_PRIV_KEY_DER_SIZE 130 00039 #define KCM_EC_SECP256R1_MAX_PUB_KEY_RAW_SIZE 65 00040 #define KCM_EC_SECP256R1_MAX_PUB_KEY_DER_SIZE 91 00041 #define KCM_ECDSA_SECP256R1_MAX_SIGNATURE_SIZE_IN_BYTES (256/8)*2 + 10 //74 bytes 00042 00043 /** 00044 * KCM file prefixes defines 00045 */ 00046 #define KCM_FILE_PREFIX_PRIVATE_KEY "PrvKey_" 00047 #define KCM_FILE_PREFIX_PUBLIC_KEY "PubKey_" 00048 #define KCM_FILE_PREFIX_SYMMETRIC_KEY "SymKey_" 00049 #define KCM_FILE_PREFIX_CERTIFICATE "Cert_" 00050 #define KCM_FILE_PREFIX_CONFIG_PARAM "CfgParam_" 00051 #define KCM_FILE_PREFIX_CERT_CHAIN_0 KCM_FILE_PREFIX_CERTIFICATE 00052 #define KCM_FILE_PREFIX_CERT_CHAIN_X "Crt1_" // must be same length as KCM_FILE_PREFIX_CERT_CHAIN_0 00053 #define KCM_FILE_PREFIX_CERT_CHAIN_X_OFFSET 3 00054 00055 #define KCM_FILE_PREFIX_MAX_SIZE 12 00056 00057 00058 // Make sure that pointer_to_complete_name points to a type of size 1 (char or uint8_t) so that arithmetic works correctly 00059 #define KCM_FILE_BASENAME(pointer_to_complete_name, prefix_define) (pointer_to_complete_name + sizeof(prefix_define) - 1) 00060 // Complete name is the prefix+name (without '/0') 00061 #define KCM_FILE_BASENAME_LEN(complete_name_size, prefix_define) (complete_name_size - (sizeof(prefix_define) - 1)) 00062 00063 00064 typedef enum { 00065 /* KCM_LOCAL_ACL_MD_TYPE, 00066 KCM_REMOTE_ACL_MD_TYPE, 00067 KCM_AUDIT_MD_TYPE, 00068 KCM_NAME_MD_TYPE, 00069 KCM_USAGE_MD_TYPE,*/ 00070 KCM_CERT_CHAIN_LEN_MD_TYPE, 00071 KCM_MD_TYPE_MAX_SIZE // can't be bigger than ESFS_MAX_TYPE_LENGTH_VALUES 00072 } kcm_meta_data_type_e; 00073 00074 #if ESFS_MAX_TYPE_LENGTH_VALUES < KCM_MD_TYPE_MAX_SIZE 00075 #error "KCM_MD_TYPE_MAX_SIZE can't be greater than ESFS_MAX_TYPE_LENGTH_VALUES" 00076 #endif 00077 00078 typedef struct kcm_meta_data_ { 00079 kcm_meta_data_type_e type; 00080 size_t data_size; 00081 uint8_t *data; 00082 } kcm_meta_data_s; 00083 00084 typedef struct kcm_meta_data_list_ { 00085 // allocate a single meta data for each type 00086 kcm_meta_data_s meta_data[KCM_MD_TYPE_MAX_SIZE]; 00087 size_t meta_data_count; 00088 } kcm_meta_data_list_s; 00089 00090 typedef struct kcm_ctx_ { 00091 esfs_file_t esfs_file_h; 00092 size_t file_size; 00093 bool is_file_size_checked; 00094 } kcm_ctx_s; 00095 00096 typedef enum { 00097 KCM_CHAIN_OP_TYPE_CREATE = 1, 00098 KCM_CHAIN_OP_TYPE_OPEN, 00099 KCM_CHAIN_OP_TYPE_MAX 00100 } kcm_chain_operation_type_e; 00101 00102 00103 /* 00104 * Structure containing all necessary data of a child X509 Certificate to be validated with its signers public key 00105 */ 00106 typedef struct kcm_cert_chain_prev_params_int_ { 00107 uint8_t signature[KCM_ECDSA_SECP256R1_MAX_SIGNATURE_SIZE_IN_BYTES]; //!< The signature of certificate. 00108 size_t signature_actual_size; //!< The size of signature. 00109 uint8_t htbs[CS_SHA256_SIZE]; //!< The hash of certificate's tbs. 00110 size_t htbs_actual_size; //!< The size of hash digest. 00111 } kcm_cert_chain_prev_params_int_s; 00112 00113 00114 /** The chain context used internally only and should not be changed by user. 00115 */ 00116 typedef struct kcm_cert_chain_context_int_ { 00117 uint8_t *chain_name; //!< The name of certificate chain. 00118 size_t chain_name_len; //!< The size of certificate chain name. 00119 size_t num_of_certificates_in_chain; //!< The number of certificate in the chain. 00120 kcm_ctx_s current_kcm_ctx; //!< Current KCM operation context. 00121 uint32_t current_cert_index; //!< Current certificate iterator. 00122 kcm_chain_operation_type_e operation_type;//!< Type of Current operation. 00123 bool chain_is_factory; //!< Is chain is a factory item, otherwise false. 00124 kcm_cert_chain_prev_params_int_s prev_cert_params; //!< Saved params of previous parsed certificate. used only in create operation 00125 } kcm_cert_chain_context_int_s; 00126 00127 00128 #ifdef __cplusplus 00129 } 00130 #endif 00131 00132 #endif //KEYS_CONFIG_MANAGER_INTERNAL_H 00133
Generated on Tue Jul 12 2022 19:12:12 by
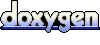