
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
fcc_status.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FCC_STATUS_H__ 00018 #define __FCC_STATUS_H__ 00019 00020 #ifdef __cplusplus 00021 extern "C" { 00022 #endif 00023 /** 00024 * @file fcc_status.h 00025 * \brief factory configurator client status/error codes. 00026 * This list may grow as needed. 00027 */ 00028 typedef enum { 00029 FCC_STATUS_SUCCESS = 0, //!< Operation completed successfully. 00030 FCC_STATUS_ERROR, //!< Operation ended with an unspecified error. 00031 FCC_STATUS_MEMORY_OUT, //!< An out-of-memory condition occurred. 00032 FCC_STATUS_INVALID_PARAMETER, //!< A parameter provided to the function was invalid. 00033 FCC_STATUS_STORE_ERROR, //!< Storage internal error. 00034 FCC_STATUS_INTERNAL_ITEM_ALREADY_EXIST,//!< Current item already exists in storage. 00035 FCC_STATUS_CA_ERROR, //!< CA Certificate already exist in storage (currently only bootstrap CA) 00036 FCC_STATUS_ROT_ERROR, //!< ROT already exist in storage 00037 FCC_STATUS_ENTROPY_ERROR, //!< Entropy already exist in storage 00038 FCC_STATUS_FACTORY_DISABLED_ERROR, //!< FCC flow was disabled - denial of service error. 00039 FCC_STATUS_INVALID_CERTIFICATE, //!< Invalid certificate found. 00040 FCC_STATUS_INVALID_CERT_ATTRIBUTE, //!< Operation failed to get an attribute. 00041 FCC_STATUS_INVALID_CA_CERT_SIGNATURE, //!< Invalid ca signature. 00042 FCC_STATUS_EXPIRED_CERTIFICATE, //!< Certificate is expired. 00043 FCC_STATUS_INVALID_LWM2M_CN_ATTR, //!< Invalid CN field of certificate. 00044 FCC_STATUS_KCM_ERROR, //!< KCM basic functionality failed. 00045 FCC_STATUS_KCM_STORAGE_ERROR, //!< KCM failed to read, write or get size of item from/to storage. 00046 FCC_STATUS_KCM_FILE_EXIST_ERROR, //!< KCM tried to create existing storage item. 00047 FCC_STATUS_KCM_CRYPTO_ERROR, //!< KCM returned error upon cryptographic check of an certificate or key. 00048 FCC_STATUS_NOT_INITIALIZED, //!< FCC failed or did not initialized. 00049 FCC_STATUS_BUNDLE_ERROR, //!< Protocol layer general error. 00050 FCC_STATUS_BUNDLE_RESPONSE_ERROR, //!< Protocol layer failed to create response buffer. 00051 FCC_STATUS_BUNDLE_UNSUPPORTED_GROUP, //!< Protocol layer detected unsupported group was found in a message. 00052 FCC_STATUS_BUNDLE_INVALID_GROUP, //!< Protocol layer detected invalid group in a message. 00053 FCC_STATUS_BUNDLE_INVALID_SCHEME, //!< The scheme version of a message in the protocol layer is wrong. 00054 FCC_STATUS_ITEM_NOT_EXIST, //!< Current item wasn't found in the storage 00055 FCC_STATUS_EMPTY_ITEM, //!< Current item's size is 0 00056 FCC_STATUS_WRONG_ITEM_DATA_SIZE, //!< Current item's size is different then expected 00057 FCC_STATUS_URI_WRONG_FORMAT, //!< Current URI is different than expected. 00058 FCC_STATUS_FIRST_TO_CLAIM_NOT_ALLOWED, //!< Can't use first to claim without bootstrap or with account ID 00059 FCC_STATUS_BOOTSTRAP_MODE_ERROR, //!< Wrong value of bootstrapUse mode. 00060 FCC_STATUS_OUTPUT_INFO_ERROR, //!< The process failed in output info creation. 00061 FCC_STATUS_WARNING_CREATE_ERROR, //!< The process failed in output info creation. 00062 FCC_STATUS_UTC_OFFSET_WRONG_FORMAT, //!< Current UTC is wrong. 00063 FCC_STATUS_CERTIFICATE_PUBLIC_KEY_CORRELATION_ERROR, //!< Certificate's public key failed do not matches to corresponding private key 00064 FCC_STATUS_BUNDLE_INVALID_KEEP_ALIVE_SESSION_STATUS,//!< The message status is invalid. 00065 FCC_STATUS_TOO_MANY_CSR_REQUESTS, //!< The message contained more than CSR_MAX_NUMBER_OF_CSRS CSR requests 00066 FCC_MAX_STATUS = 0x7fffffff 00067 } fcc_status_e ; 00068 00069 #ifdef __cplusplus 00070 } 00071 #endif 00072 00073 #endif //__FCC_STATUS_H__
Generated on Tue Jul 12 2022 19:12:12 by
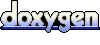