
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
fcc_output_info_handler.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FCC_OUTPUT_INFO_HANDLER_H__ 00018 #define __FCC_OUTPUT_INFO_HANDLER_H__ 00019 00020 #include <stdlib.h> 00021 #include <stdbool.h> 00022 #include <inttypes.h> 00023 #include "kcm_status.h" 00024 #include "fcc_output_info_handler_defines.h" 00025 #include "fcc_defs.h" 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 /** 00032 * Initializes resources of output info handler 00033 */ 00034 void fcc_init_output_info_handler( void ); 00035 00036 /** 00037 * Finalizes resources of output info handler 00038 */ 00039 void fcc_clean_output_info_handler( void ); 00040 00041 /** 00042 * Returns true if FCC was initialized false otherwise 00043 */ 00044 bool is_fcc_initialized(void); 00045 00046 /** The function stores the name of failed item and kcm error string in global variables 00047 * The error returned by fcc_bundle_handler API. 00048 * 00049 * @param failed_item_name[in] The name of failed item 00050 * @param failed_item_name_size[in] The size of failed item name. 00051 * @param kcm_status[in] The kcm status value. 00052 * 00053 * @return 00054 * true for success, false otherwise. 00055 */ 00056 fcc_status_e fcc_bundle_store_error_info(const uint8_t *failed_item_name, size_t failed_item_name_size, kcm_status_e kcm_status); 00057 00058 /** The function stores the name of failed item and fcc error string in global variables 00059 *The error returned by fcc_verify_device_configured_4mbed_cloud API. 00060 00061 * @param failed_item_name[in] The name of failed item. If NULL, error will be stored without an item name. 00062 * @param failed_item_name_size[in] The size of failed item name. 00063 * @param fcc_status[in] The fcc status value. 00064 * 00065 * @return 00066 * true for success, false otherwise. 00067 */ 00068 fcc_status_e fcc_store_error_info(const uint8_t *failed_item_name, size_t failed_item_name_size, fcc_status_e fcc_status); 00069 00070 /** The function stores the all collected warnings and relevant item names during fcc_verify_device_configured_4mbed_cloud API. 00071 * 00072 * @param failed_item_name[in] The name of failed item 00073 * @param failed_item_name_size[in] The size of failed item name. 00074 * @param fcc_status[in] The fcc status value. 00075 * 00076 * @return 00077 * true for success, false otherwise. 00078 */ 00079 fcc_status_e fcc_store_warning_info(const uint8_t *failed_item_name, size_t failed_item_name_size, const char *warning_string); 00080 /** The function return saved failed item name 00081 * 00082 * @return 00083 * NULL if no errors or char* pointer to the saved item name 00084 */ 00085 char* fcc_get_output_error_info( void ); 00086 /** The function return saved warnings as single string 00087 * 00088 * @return 00089 * NULL if no warnings exist or char* pointer to the string of all warnings 00090 */ 00091 char* fcc_get_output_warning_info(void); 00092 00093 /** The function returns relevant pointer to string of passed fcc_status. 00094 * 00095 * @return 00096 * string /NULL in case the fcc_status string wasn't found 00097 */ 00098 char* fcc_get_fcc_error_string(fcc_status_e fcc_status); 00099 00100 /** The function returns relevant pointer to string of passed kcm_status. 00101 * 00102 * @return 00103 * string /NULL in case the kcm_status string wasn't found 00104 */ 00105 char* fcc_get_kcm_error_string(kcm_status_e kcm_status); 00106 00107 /** The function gets output info structure 00108 * 00109 * @return 00110 */ 00111 fcc_output_info_s* get_output_info(void); 00112 00113 /** The function gets status of warning info 00114 * 00115 * @return 00116 * true - if warnings were stored, false in case of no warning 00117 */ 00118 bool fcc_get_warning_status(void); 00119 00120 #ifdef __cplusplus 00121 } 00122 #endif 00123 00124 #endif //__FCC_OUTPUT_INFO_HANDLER_H__
Generated on Tue Jul 12 2022 19:12:12 by
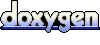