
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
fcc_malloc.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FCC_MALLOC_H__ 00018 #define __FCC_MALLOC_H__ 00019 00020 #include <stddef.h> 00021 00022 #ifdef __cplusplus 00023 extern "C" { 00024 #endif 00025 00026 00027 /** 00028 * Allocate the requested amount of bytes and log heap statistics. 00029 * - It is assumed FCC running in a single thread (no thread safety) 00030 * - This function does not allows re-entrance 00031 * 00032 * @param size The amount of bytes to allocate on the heap memory 00033 * 00034 * @returns 00035 * If allocation succeeded - a valid pointer to the beginning of the allocated heap memory 00036 * If allocation failed - a NULL pointer will be returned 00037 */ 00038 void *fcc_malloc(size_t size); 00039 00040 /** 00041 * Free the heap bytes followed by the given pointer and log heap statistics. 00042 * - It is assumed FCC running in a single thread (no thread safety) 00043 * - This function does not allows re-entrance 00044 * 00045 * @param ptr A pointer to the beginning of bytes allocated on the heap memory 00046 */ 00047 void fcc_free(void *ptr); 00048 00049 #ifndef FCC_MEM_STATS_ENABLED 00050 #define fcc_malloc(size) malloc( (size) ) 00051 #define fcc_free(ptr) free( (ptr) ) 00052 #endif 00053 00054 #ifdef __cplusplus 00055 } 00056 #endif 00057 00058 #endif
Generated on Tue Jul 12 2022 19:12:12 by
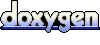