
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
fcc_bundle_utils.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 #ifndef USE_TINY_CBOR 00017 00018 #ifndef __FCC_BUNDLE_UTILS_H__ 00019 #define __FCC_BUNDLE_UTILS_H__ 00020 00021 #include <stdlib.h> 00022 #include <stdbool.h> 00023 #include <inttypes.h> 00024 #include "fcc_status.h" 00025 #include "key_config_manager.h" 00026 #include "fcc_sotp.h" 00027 #include "cn-cbor.h" 00028 #include "fcc_bundle_fields.h" 00029 00030 #ifdef __cplusplus 00031 extern "C" { 00032 #endif 00033 00034 #define FCC_CBOR_MAP_LENGTH 2 00035 #define CSR_MAX_NUMBER_OF_CSRS 5 00036 00037 /** 00038 * Types of key parameters 00039 */ 00040 typedef enum { 00041 FCC_BUNDLE_DATA_PARAM_NAME_TYPE, 00042 FCC_BUNDLE_DATA_PARAM_SCHEME_TYPE, 00043 FCC_BUNDLE_DATA_PARAM_FORMAT_TYPE, 00044 FCC_BUNDLE_DATA_PARAM_DATA_TYPE, 00045 FCC_BUNDLE_DATA_PARAM_ACL_TYPE, 00046 FCC_BUNDLE_DATA_PARAM_ARRAY_TYPE, 00047 FCC_BUNDLE_DATA_PARAMETER_PRIVATE_KEY_NAME_TYPE, 00048 FCC_BUNDLE_DATA_PARAM_MAX_TYPE 00049 } fcc_bundle_data_param_type_e; 00050 00051 /** 00052 * Key lookup record, correlating key's param type and name 00053 */ 00054 typedef struct fcc_bundle_data_param_lookup_record_ { 00055 fcc_bundle_data_param_type_e data_param_type; 00056 const char *data_param_name; 00057 } fcc_bundle_data_param_lookup_record_s; 00058 00059 /** 00060 * Key lookup table, correlating for each key its param type and param name 00061 */ 00062 static const fcc_bundle_data_param_lookup_record_s fcc_bundle_data_param_lookup_table[FCC_BUNDLE_DATA_PARAM_MAX_TYPE] = { 00063 { FCC_BUNDLE_DATA_PARAM_NAME_TYPE, FCC_BUNDLE_DATA_PARAMETER_NAME }, 00064 { FCC_BUNDLE_DATA_PARAM_SCHEME_TYPE, FCC_BUNDLE_DATA_PARAMETER_SCHEME }, 00065 { FCC_BUNDLE_DATA_PARAM_FORMAT_TYPE, FCC_BUNDLE_DATA_PARAMETER_FORMAT }, 00066 { FCC_BUNDLE_DATA_PARAM_DATA_TYPE, FCC_BUNDLE_DATA_PARAMETER_DATA }, 00067 { FCC_BUNDLE_DATA_PARAM_ACL_TYPE, FCC_BUNDLE_DATA_PARAMETER_ACL }, 00068 { FCC_BUNDLE_DATA_PARAM_ARRAY_TYPE, FCC_BUNDLE_DATA_PARAMETER_ARRAY }, 00069 { FCC_BUNDLE_DATA_PARAMETER_PRIVATE_KEY_NAME_TYPE, FCC_BUNDLE_DATA_PARAMETER_PRIVATE_KEY_NAME } 00070 }; 00071 00072 /** 00073 * Source type of buffer 00074 */ 00075 typedef enum { 00076 FCC_EXTERNAL_BUFFER_TYPE, 00077 FCC_INTERNAL_BUFFER_TYPE, 00078 FCC_MAX_BUFFER_TYPE 00079 } fcc_bundle_buffer_type_e; 00080 00081 /** 00082 * Data formats supported by FC 00083 */ 00084 typedef enum { 00085 FCC_INVALID_DATA_FORMAT, 00086 FCC_DER_DATA_FORMAT, 00087 FCC_PEM_DATA_FORMAT, 00088 FCC_MAX_DATA_FORMAT 00089 } fcc_bundle_data_format_e; 00090 00091 /** 00092 * Group lookup record, correlating group's type and name 00093 */ 00094 typedef struct fcc_bundle_data_format_lookup_record_ { 00095 fcc_bundle_data_format_e data_format_type; 00096 const char *data_format_name; 00097 } fcc_bundle_data_format_lookup_record_s; 00098 00099 /** 00100 * Group lookup table, correlating for each group its type and name 00101 */ 00102 static const fcc_bundle_data_format_lookup_record_s fcc_bundle_data_format_lookup_table[FCC_MAX_DATA_FORMAT] = { 00103 { FCC_DER_DATA_FORMAT, FCC_BUNDLE_DER_DATA_FORMAT_NAME }, 00104 { FCC_PEM_DATA_FORMAT, FCC_BUNDLE_PEM_DATA_FORMAT_NAME }, 00105 }; 00106 00107 /** 00108 * Key types supported by FC 00109 */ 00110 typedef enum { 00111 FCC_INVALID_KEY_TYPE, 00112 FCC_ECC_PRIVATE_KEY_TYPE,//do not change this type's place.FCC_ECC_PRIVATE_KEY_TYPE should be at first place. 00113 FCC_ECC_PUBLIC_KEY_TYPE, 00114 FCC_RSA_PRIVATE_KEY_TYPE, 00115 FCC_RSA_PUBLIC_KEY_TYPE, 00116 FCC_SYM_KEY_TYPE, 00117 FCC_MAX_KEY_TYPE 00118 } fcc_bundle_key_type_e; 00119 00120 typedef struct fcc_bundle_data_param_ { 00121 uint8_t *name; 00122 size_t name_len; 00123 fcc_bundle_data_format_e format; 00124 fcc_bundle_key_type_e type; 00125 uint8_t *data; 00126 size_t data_size; 00127 uint8_t *data_der; 00128 size_t data_der_size; 00129 fcc_bundle_buffer_type_e data_type; 00130 uint8_t *acl; 00131 size_t acl_size; 00132 cn_cbor *array_cn; 00133 uint8_t *private_key_name; 00134 size_t private_key_name_len; 00135 } fcc_bundle_data_param_s; 00136 00137 00138 /** Frees all allocated memory of data parameter struct and sets initial values. 00139 * 00140 * @param data_param[in/out] The data parameter structure 00141 */ 00142 void fcc_bundle_clean_and_free_data_param(fcc_bundle_data_param_s *data_param); 00143 00144 /** Gets data buffer from cbor struct. 00145 * 00146 * @param data_cb[in] The cbor text structure 00147 * @param out_data_buffer[out] The out buffer for string data 00148 * @param out_size[out] The actual size of output buffer 00149 * 00150 * @return 00151 * true for success, false otherwise. 00152 */ 00153 bool get_data_buffer_from_cbor(const cn_cbor *data_cb, uint8_t **out_data_buffer, size_t *out_size); 00154 00155 /** Processes keys list. 00156 * The function extracts data parameters for each key and stores its according to it type. 00157 * 00158 * @param keys_list_cb[in] The cbor structure with keys list. 00159 * 00160 * @return 00161 * fcc_status_e status. 00162 */ 00163 fcc_status_e fcc_bundle_process_keys(const cn_cbor *keys_list_cb); 00164 00165 /** Processes certificate list. 00166 * The function extracts data parameters for each certificate and stores it. 00167 * 00168 * @param certs_list_cb[in] The cbor structure with certificate list. 00169 * 00170 * @return 00171 * fcc_status_e status. 00172 */ 00173 fcc_status_e fcc_bundle_process_certificates(const cn_cbor *certs_list_cb); 00174 /** Processes certificate chain list. 00175 * The function extracts data parameters for each certificate chain and stores it. 00176 * 00177 * @param certs_list_cb[in] The cbor structure with certificate chain list. 00178 * 00179 * @return 00180 * fcc_status_e status. 00181 */ 00182 fcc_status_e fcc_bundle_process_certificate_chains(const cn_cbor *cert_chains_list_cb); 00183 00184 /** Processes configuration parameters list. 00185 * The function extracts data parameters for each config param and stores it. 00186 * 00187 * @param config_params_list_cb[in] The cbor structure with config param list. 00188 * 00189 * @return 00190 * fcc_status_e status. 00191 */ 00192 fcc_status_e fcc_bundle_process_config_params(const cn_cbor *config_params_list_cb); 00193 00194 /** Gets data parameters. 00195 * 00196 * The function goes over all existing parameters (name,type,format,data,acl and etc) and 00197 * tries to find correlating parameter in cbor structure and saves it to data parameter structure. 00198 * 00199 * @param data_param_cb[in] The cbor structure with relevant data parameters. 00200 * @param data_param[out] The data parameter structure 00201 * 00202 * @return 00203 * true for success, false otherwise. 00204 */ 00205 bool fcc_bundle_get_data_param(const cn_cbor *data_param_list_cb, fcc_bundle_data_param_s *data_param); 00206 00207 /** Gets type of key form cbor structure 00208 * 00209 * The function goes over all key types and compares it with type inside cbor structure. 00210 * 00211 * @param key_type_cb[in] The cbor structure with key type data. 00212 * @param key_type[out] The key type 00213 * 00214 * @return 00215 * true for success, false otherwise. 00216 */ 00217 bool fcc_bundle_get_key_type(const cn_cbor *key_type_cb, fcc_bundle_key_type_e *key_type); 00218 00219 /** Writes buffer to SOTP 00220 * 00221 * @param cbor_bytes[in] The pointer to a cn_cbor object of type CN_CBOR_BYTES. 00222 * @param sotp_type[in] enum representing the type of the item to be stored in SOTP. 00223 * @return 00224 * true for success, false otherwise. 00225 */ 00226 00227 fcc_status_e fcc_bundle_process_sotp_buffer(cn_cbor *cbor_bytes, sotp_type_e sotp_type); 00228 00229 /** Gets the status groups value 00230 * 00231 * - if value is '0' - set status to false 00232 * - if value is '1' - set status to true 00233 * 00234 * @param cbor_blob[in] The pointer to main CBOR blob. 00235 * @param cbor_group_name[in] CBOT group name. 00236 * @param cbor_group_name_size[in] CBOR group name size . 00237 * @param fcc_field_status[out] Status of the field. 00238 * 00239 * @return 00240 * One of FCC_STATUS_* error codes 00241 */ 00242 fcc_status_e bundle_process_status_field(const cn_cbor *cbor_blob, char *cbor_group_name, size_t cbor_group_name_size, bool *fcc_field_status); 00243 00244 /** The function sets factory disable flag to sotp. 00245 * 00246 * @return 00247 * One of FCC_STATUS_* error codes 00248 */ 00249 fcc_status_e fcc_bundle_factory_disable(void); 00250 00251 /** Process the the CSR requests from the incoming message, generate the keys (and store them) and CSRs, and append the CSRs to the encoder in the proper format. 00252 * 00253 * 00254 * @param csrs_list_cb[in] The pointer to a cn_cbor object of type CN_CBOR_ARRAY which is an array of CSR request maps. 00255 * @param response_encoder[in/out] encoder that points to the response map. 00256 * @return 00257 * One of FCC_STATUS_* error codes 00258 */ 00259 fcc_status_e fcc_bundle_process_csrs(const cn_cbor *csrs_list_cb, cn_cbor *response_encoder); 00260 00261 #ifdef __cplusplus 00262 } 00263 #endif 00264 00265 #endif //__FCC_BUNDLE_UTILS_H__ 00266 #endif
Generated on Tue Jul 12 2022 19:12:12 by
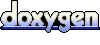