
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
fcc_bundle_key_utils.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 #ifndef USE_TINY_CBOR 00017 #include "fcc_bundle_handler.h" 00018 #include "cn-cbor.h" 00019 #include "pv_error_handling.h" 00020 #include "fcc_bundle_utils.h" 00021 #include "key_config_manager.h" 00022 #include "fcc_output_info_handler.h" 00023 #include "general_utils.h" 00024 #include "fcc_time_profiling.h" 00025 #include "fcc_utils.h" 00026 00027 #define FCC_MAX_PEM_KEY_SIZE 1024*2 00028 /** 00029 * Names of key types 00030 */ 00031 #define FCC_ECC_PRIVATE_KEY_TYPE_NAME "ECCPrivate" 00032 #define FCC_ECC_PUBLIC_KEY_TYPE_NAME "ECCPublic" 00033 #define FCC_RSA_PRIVATE_KEY_TYPE_NAME "RSAPrivate" 00034 #define FCC_RSA_PUBLIC_KEY_TYPE_NAME "RSAPublic" 00035 #define FCC_SYMMETRIC_KEY_TYPE_NAME "Symmetric" 00036 /** 00037 * Group lookup record, correlating group's type and name 00038 */ 00039 typedef struct fcc_bundle_key_type_lookup_record_ { 00040 fcc_bundle_key_type_e key_type; 00041 const char *key_type_name; 00042 } fcc_bundle_key_type_lookup_record_s; 00043 /** 00044 * Group lookup table, correlating for each group its type and name 00045 */ 00046 static const fcc_bundle_key_type_lookup_record_s fcc_bundle_key_type_lookup_table[FCC_MAX_KEY_TYPE] = { 00047 { FCC_ECC_PRIVATE_KEY_TYPE, FCC_ECC_PRIVATE_KEY_TYPE_NAME }, 00048 { FCC_ECC_PUBLIC_KEY_TYPE, FCC_ECC_PUBLIC_KEY_TYPE_NAME }, 00049 { FCC_RSA_PRIVATE_KEY_TYPE, FCC_RSA_PRIVATE_KEY_TYPE_NAME }, 00050 { FCC_RSA_PUBLIC_KEY_TYPE, FCC_RSA_PUBLIC_KEY_TYPE_NAME }, 00051 { FCC_SYM_KEY_TYPE, FCC_SYMMETRIC_KEY_TYPE_NAME } 00052 }; 00053 /** Gets type of key form cbor structure 00054 * 00055 * The function goes over all key types and compares it with type inside cbor structure. 00056 * 00057 * @param key_type_cb[in] The cbor structure with key type data. 00058 * @param key_type[out] The key type 00059 * 00060 * @return 00061 * true for success, false otherwise. 00062 */ 00063 bool fcc_bundle_get_key_type(const cn_cbor *key_type_cb, fcc_bundle_key_type_e *key_type) 00064 { 00065 00066 int key_type_index; 00067 bool res; 00068 00069 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00070 00071 SA_PV_ERR_RECOVERABLE_RETURN_IF((key_type_cb == NULL), false, "key_type_cb is null"); 00072 SA_PV_ERR_RECOVERABLE_RETURN_IF((key_type == NULL), false, "key_type is null"); 00073 SA_PV_ERR_RECOVERABLE_RETURN_IF((*key_type != FCC_INVALID_KEY_TYPE), false, "wrong key type value"); 00074 00075 for (key_type_index = 0; key_type_index < FCC_MAX_KEY_TYPE -1; key_type_index++) { 00076 res = is_memory_equal(fcc_bundle_key_type_lookup_table[key_type_index].key_type_name, 00077 strlen(fcc_bundle_key_type_lookup_table[key_type_index].key_type_name), 00078 key_type_cb->v.bytes, 00079 (size_t)key_type_cb->length); 00080 if (res) { 00081 *key_type = fcc_bundle_key_type_lookup_table[key_type_index].key_type; 00082 return true; 00083 } 00084 } 00085 SA_PV_LOG_TRACE_FUNC_EXIT("key_type is %d", (int)(*key_type)); 00086 return false; 00087 00088 } 00089 00090 /** Processes keys list. 00091 * The function extracts data parameters for each key and stores its according to it type. 00092 * 00093 * @param keys_list_cb[in] The cbor structure with keys list. 00094 * 00095 * @return 00096 * true for success, false otherwise. 00097 */ 00098 fcc_status_e fcc_bundle_process_keys(const cn_cbor *keys_list_cb) 00099 { 00100 00101 bool status = false; 00102 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00103 fcc_status_e output_info_fcc_status = FCC_STATUS_SUCCESS; 00104 kcm_status_e kcm_result = KCM_STATUS_SUCCESS; 00105 uint32_t key_index = 0; 00106 cn_cbor *key_cb; 00107 fcc_bundle_data_param_s key; 00108 00109 SA_PV_LOG_TRACE_FUNC_ENTER_NO_ARGS(); 00110 SA_PV_ERR_RECOVERABLE_RETURN_IF((keys_list_cb == NULL), fcc_status = FCC_STATUS_INVALID_PARAMETER, "Invalid keys_list_cb pointer"); 00111 00112 //Initialize data struct 00113 memset(&key,0,sizeof(fcc_bundle_data_param_s)); 00114 00115 for (key_index = 0; key_index < (uint32_t)keys_list_cb->length; key_index++) { 00116 00117 FCC_SET_START_TIMER(fcc_key_timer); 00118 00119 //fcc_bundle_clean_and_free_data_param(&key); 00120 00121 //Get key CBOR struct at index key_index 00122 key_cb = cn_cbor_index(keys_list_cb, key_index); 00123 SA_PV_ERR_RECOVERABLE_RETURN_IF((key_cb == NULL), fcc_status = FCC_STATUS_BUNDLE_ERROR, "Failed to get key at index (%" PRIu32 ") ", key_index); 00124 SA_PV_ERR_RECOVERABLE_RETURN_IF((key_cb->type != CN_CBOR_MAP), fcc_status = FCC_STATUS_BUNDLE_ERROR, "Wrong type of key CBOR struct at index (%" PRIu32 ")", key_index); 00125 00126 status = fcc_bundle_get_data_param(key_cb, &key); 00127 SA_PV_ERR_RECOVERABLE_RETURN_IF((status != true), fcc_status = FCC_STATUS_BUNDLE_ERROR, "Failed to get key data at index (%" PRIu32 ") ", key_index); 00128 00129 switch (key.type) { 00130 case FCC_ECC_PRIVATE_KEY_TYPE: 00131 case FCC_RSA_PRIVATE_KEY_TYPE: 00132 kcm_result = kcm_item_store(key.name, key.name_len, KCM_PRIVATE_KEY_ITEM, true, key.data, key.data_size, key.acl); 00133 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_result != KCM_STATUS_SUCCESS), fcc_status = fcc_convert_kcm_to_fcc_status(kcm_result), exit, "Failed to store key private at index (%" PRIu32 ") ", key_index); 00134 break; 00135 00136 case FCC_ECC_PUBLIC_KEY_TYPE: 00137 case FCC_RSA_PUBLIC_KEY_TYPE: 00138 kcm_result = kcm_item_store(key.name, key.name_len, KCM_PUBLIC_KEY_ITEM, true, key.data, key.data_size, key.acl); 00139 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_result != KCM_STATUS_SUCCESS), fcc_status = fcc_convert_kcm_to_fcc_status(kcm_result), exit, "Failed to store key public at index (%" PRIu32 ") ", key_index); 00140 break; 00141 00142 case (FCC_SYM_KEY_TYPE): 00143 kcm_result = kcm_item_store(key.name, key.name_len, KCM_SYMMETRIC_KEY_ITEM, true, key.data, key.data_size, key.acl); 00144 SA_PV_ERR_RECOVERABLE_GOTO_IF((kcm_result != KCM_STATUS_SUCCESS), fcc_status = fcc_convert_kcm_to_fcc_status(kcm_result), exit, "Failed to store symmetric key at index (%" PRIu32 ") ", key_index); 00145 break; 00146 default: 00147 SA_PV_LOG_ERR("Invalid key type (%u)!", key.type); 00148 goto exit; 00149 } 00150 FCC_END_TIMER((char*)key.name, key.name_len, fcc_key_timer); 00151 } 00152 00153 exit: 00154 if (kcm_result != KCM_STATUS_SUCCESS) { 00155 output_info_fcc_status = fcc_bundle_store_error_info(key.name, key.name_len, kcm_result); 00156 SA_PV_ERR_RECOVERABLE_RETURN_IF((output_info_fcc_status != FCC_STATUS_SUCCESS), 00157 fcc_status = FCC_STATUS_OUTPUT_INFO_ERROR, 00158 "Failed to create output kcm_status error %d", kcm_result); 00159 } 00160 fcc_bundle_clean_and_free_data_param(&key); 00161 SA_PV_LOG_TRACE_FUNC_EXIT_NO_ARGS(); 00162 return fcc_status; 00163 } 00164 #endif
Generated on Tue Jul 12 2022 19:12:12 by
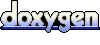