
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
factory_configurator_client.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #ifndef __FACTORY_CONFIGURATOR_CLIENT_H__ 00018 #define __FACTORY_CONFIGURATOR_CLIENT_H__ 00019 00020 #include <stdlib.h> 00021 #include <inttypes.h> 00022 #include "fcc_status.h" 00023 #include "fcc_output_info_handler.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 /** 00030 * @file factory_configurator_client.h 00031 * \brief factory configurator client APIs. 00032 */ 00033 00034 /* === Initialization and Finalization === */ 00035 00036 /** Initiates the FCC module. Must be called before any other fcc's APIs. Otherwise relevant error will be returned. 00037 * 00038 * @returns 00039 * FCC_STATUS_SUCCESS in case of success or one of the `::fcc_status_e` errors otherwise. 00040 */ 00041 fcc_status_e fcc_init(void); 00042 00043 00044 /** Finalizes the FCC module. 00045 * Finalizes and frees file storage resources. 00046 * 00047 * @returns 00048 * FCC_STATUS_SUCCESS in case of success or one of the `::fcc_status_e` errors otherwise. 00049 */ 00050 00051 fcc_status_e fcc_finalize(void); 00052 00053 /* === Factory clean operation === */ 00054 00055 /** Cleans from the device all data that was saved during the factory process. 00056 * Should be called if the process failed and needs to be executed again. 00057 * 00058 * @returns 00059 * FCC_STATUS_SUCCESS in case of success or one of the `::fcc_status_e` errors otherwise. 00060 */ 00061 fcc_status_e fcc_storage_delete(void); 00062 00063 00064 /* === Warning and errors data operations === */ 00065 00066 /** The function retrieves pointer to warning and errors structure. 00067 * Should be called after fcc_verify_device_configured_4mbed_cloud, when possible warning and errors was 00068 * stored in the structure. 00069 * The structure contains data of last fcc_verify_device_configured_4mbed_cloud run.* 00070 * @returns pointer to fcc_output_info_s structure. 00071 * 00072 * Example: 00073 * @code 00074 * void print_fcc_output_info(fcc_output_info_s *output_info) 00075 * { 00076 * fcc_warning_info_s *warning_list = NULL; 00077 * 00078 * if (output_info != NULL) { 00079 * // Check if there is an error 00080 * if (output_info->error_string_info != NULL) { 00081 * // Print the error string 00082 * printf("fcc output error: %s", output_info->error_string_info); 00083 * } 00084 * // Check if there are warnings 00085 * if (output_info->size_of_warning_info_list > 0) { 00086 * // Set warning_list to point on the head of the list 00087 * warning_list = output_info->head_of_warning_list; 00088 * 00089 * // Iterate the list 00090 * while (warning_list != NULL) { 00091 * // Print the warning string 00092 * printf("fcc output warning: %s", warning_list->warning_info_string); 00093 * // Move warning_list to point on the next warning in he list 00094 * warning_list = warning_list->next; 00095 * } 00096 * } 00097 * } 00098 * } 00099 * @endcode 00100 * 00101 */ 00102 fcc_output_info_s* fcc_get_error_and_warning_data(void); 00103 00104 /** The function returns status of current session between the FCC and the FCU. 00105 * If the returned value is true - the session should be finished in the communication layer after current message processing, 00106 * if the return value is false - the session should be kept alive for next message. 00107 * 00108 * @returns 00109 * bool 00110 */ 00111 bool fcc_is_session_finished(void); 00112 00113 /* === Verification === */ 00114 00115 /** Verifies that all mandatory fields needed to connect to mbed Cloud are in place on the device. 00116 * Should be called in the end of the factory process 00117 * 00118 * @returns 00119 * FCC_STATUS_SUCCESS in case of success or one of the `::fcc_status_e` errors otherwise. 00120 */ 00121 fcc_status_e fcc_verify_device_configured_4mbed_cloud(void); 00122 00123 00124 /* === Secure Time === */ 00125 00126 /** Sets device time. This function will set the device time to what the user provides. 00127 * Device time must be set in order to enable certificate expiration validations. 00128 * 00129 * @param time The device time to set. As epoch time (number of seconds that have elapsed since January 1, 1970) 00130 * 00131 * @returns 00132 * Operation status. 00133 */ 00134 fcc_status_e fcc_time_set(uint64_t time); 00135 00136 00137 /* === Entropy and RoT injection === */ 00138 00139 /** Sets Entropy. 00140 * If device does not have its own entropy - this function must be called after fcc_init() and prior to any other FCC or KCM functions. 00141 * This API should be used if device has its own entropy and user wishes to add his own entropy. 00142 * 00143 * @param buf The buffer containing the entropy. 00144 * @param buf_size The size of buf in bytes. Must be exactly FCC_ENTROPY_SIZE. 00145 * 00146 * @returns 00147 * Operation status. 00148 */ 00149 fcc_status_e fcc_entropy_set(const uint8_t *buf, size_t buf_size); 00150 00151 /** Sets root of trust 00152 * If user wishes to set his own root of trust, this function must be called after fcc_init() and fcc_entropy_set() (if user sets his own entropy), 00153 * and prior to any other FCC or KCM functions. 00154 * 00155 * @param buf The buffer containing the root of trust. 00156 * @param buf_size The size of buf in bytes. Must be exactly FCC_ROT_SIZE. 00157 * 00158 * @returns 00159 * Operation status. 00160 */ 00161 fcc_status_e fcc_rot_set(const uint8_t *buf, size_t buf_size); 00162 00163 /* === Bootstrap CA certificate identification storage === */ 00164 00165 /** The function sets bootstrap ca identification and stores it. 00166 * Should be called only after storing bootstrap ca certificate on the device. 00167 * 00168 * @returns 00169 * Operation status. 00170 */ 00171 fcc_status_e fcc_trust_ca_cert_id_set(void); 00172 00173 00174 /* === Factory flow disable === */ 00175 /** Sets Factory disabled flag to disable further use of the factory flow. 00176 * 00177 * @returns 00178 * Operation status. 00179 */ 00180 fcc_status_e fcc_factory_disable(void); 00181 00182 /** Returns true if the factory flow was disabled by calling fcc_factory_disable() API, outherwise 00183 * returns false. 00184 * 00185 * - If the factory flow is already disabled any FCC API(s) will fail. 00186 * 00187 * @param fcc_factory_disable An output parameter, will be set to "true" in case factory 00188 * flow is already disabled, "false" otherwise. 00189 * 00190 * @returns 00191 * FCC_STATUS_SUCCESS in case of success or one of the `::fcc_status_e` errors otherwise. 00192 */ 00193 fcc_status_e fcc_is_factory_disabled(bool *fcc_factory_disable); 00194 00195 /* === Developer flow === */ 00196 00197 /** This API is for developers only. 00198 * You can download the `mbed_cloud_dev_credentials.c` file from the portal and thus, skip running FCU on PC side. 00199 * The API reads all credentials from the `mbed_cloud_dev_credentials.c` file and stores them in the KCM. 00200 * RoT, Entropy and Time configurations are not a part of fcc_developer_flow() API. Devices that need to set RoT or Entropy 00201 * should call `fcc_rot_set()`/`fcc_entropy_set()` APIs before fcc_developer_flow(). 00202 * If device does not have it's own time configuration and `fcc_time_set()` was not called before fcc_developer_flow(), 00203 * during fcc_verify_device_configured_4mbed_cloud() certificate time validity will not be checked. 00204 * 00205 * 00206 * @returns 00207 * FCC_STATUS_SUCCESS in case of success or one of the `::fcc_status_e` errors otherwise. 00208 */ 00209 fcc_status_e fcc_developer_flow(void); 00210 00211 00212 #ifdef __cplusplus 00213 } 00214 #endif 00215 00216 #endif //__FACTORY_CONFIGURATOR_CLIENT_H__
Generated on Tue Jul 12 2022 19:12:12 by
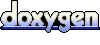