
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
factory_configurator_client.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2018 ARM Ltd. 00003 // 00004 // Licensed under the Apache License, Version 2.0 (the "License"); 00005 // you may not use this file except in compliance with the License. 00006 // You may obtain a copy of the License at 00007 // 00008 // http://www.apache.org/licenses/LICENSE-2.0 00009 // 00010 // Unless required by applicable law or agreed to in writing, software 00011 // distributed under the License is distributed on an "AS IS" BASIS, 00012 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 // See the License for the specific language governing permissions and 00014 // limitations under the License. 00015 // ---------------------------------------------------------------------------- 00016 00017 #include "factory_configurator_client.h" 00018 #include "fcc_sotp.h" 00019 #include "key_config_manager.h" 00020 #include "pv_error_handling.h" 00021 #include "fcc_verification.h" 00022 #include "storage.h" 00023 #include "fcc_defs.h" 00024 #include "fcc_malloc.h" 00025 #include "common_utils.h" 00026 #include "pal.h" 00027 00028 /** 00029 * Device general info 00030 */ 00031 const char g_fcc_use_bootstrap_parameter_name[] = "mbed.UseBootstrap"; 00032 const char g_fcc_endpoint_parameter_name[] = "mbed.EndpointName"; 00033 const char g_fcc_account_id_parameter_name[] = "mbed.AccountID"; 00034 const char g_fcc_first_to_claim_parameter_name[] = "mbed.FirstToClaim"; 00035 00036 /** 00037 * Device meta data 00038 */ 00039 const char g_fcc_manufacturer_parameter_name[] = "mbed.Manufacturer"; 00040 const char g_fcc_model_number_parameter_name[] = "mbed.ModelNumber"; 00041 const char g_fcc_device_type_parameter_name[] = "mbed.DeviceType"; 00042 const char g_fcc_hardware_version_parameter_name[] = "mbed.HardwareVersion"; 00043 const char g_fcc_memory_size_parameter_name[] = "mbed.MemoryTotalKB"; 00044 const char g_fcc_device_serial_number_parameter_name[] = "mbed.SerialNumber"; 00045 /** 00046 * Time Synchronization 00047 */ 00048 const char g_fcc_current_time_parameter_name[] = "mbed.CurrentTime"; 00049 const char g_fcc_device_time_zone_parameter_name[] = "mbed.Timezone"; 00050 const char g_fcc_offset_from_utc_parameter_name[] = "mbed.UTCOffset"; 00051 /** 00052 * Bootstrap configuration 00053 */ 00054 const char g_fcc_bootstrap_server_ca_certificate_name[] = "mbed.BootstrapServerCACert"; 00055 const char g_fcc_bootstrap_server_crl_name[] = "mbed.BootstrapServerCRL"; 00056 const char g_fcc_bootstrap_server_uri_name[] = "mbed.BootstrapServerURI"; 00057 const char g_fcc_bootstrap_device_certificate_name[] = "mbed.BootstrapDeviceCert"; 00058 const char g_fcc_bootstrap_device_private_key_name[] = "mbed.BootstrapDevicePrivateKey"; 00059 /** 00060 * LWm2m configuration 00061 */ 00062 const char g_fcc_lwm2m_server_ca_certificate_name[] = "mbed.LwM2MServerCACert"; 00063 const char g_fcc_lwm2m_server_crl_name[] = "mbed.LwM2MServerCRL"; 00064 const char g_fcc_lwm2m_server_uri_name[] = "mbed.LwM2MServerURI"; 00065 const char g_fcc_lwm2m_device_certificate_name[] = "mbed.LwM2MDeviceCert"; 00066 const char g_fcc_lwm2m_device_private_key_name[] = "mbed.LwM2MDevicePrivateKey"; 00067 /** 00068 * Firmware update 00069 */ 00070 const char g_fcc_update_authentication_certificate_name[] = "mbed.UpdateAuthCert"; 00071 const char g_fcc_class_id_name[] = "mbed.ClassId"; 00072 const char g_fcc_vendor_id_name[] = "mbed.VendorId"; 00073 00074 static bool g_is_fcc_initialized = false; 00075 bool g_is_session_finished = true; 00076 00077 fcc_status_e fcc_init(void) 00078 { 00079 palStatus_t pal_status; 00080 00081 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00082 00083 if (g_is_fcc_initialized) { 00084 // No need for second initialization 00085 return FCC_STATUS_SUCCESS; 00086 } 00087 00088 pal_status = pal_init(); 00089 SA_PV_ERR_RECOVERABLE_RETURN_IF((pal_status != PAL_SUCCESS), FCC_STATUS_ERROR, "Failed initializing PAL (%" PRIu32 ")", pal_status); 00090 00091 //Initialize output info handler 00092 fcc_init_output_info_handler(); 00093 00094 g_is_fcc_initialized = true; 00095 00096 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00097 00098 return FCC_STATUS_SUCCESS; 00099 } 00100 00101 fcc_status_e fcc_finalize(void) 00102 { 00103 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00104 kcm_status_e kcm_status = KCM_STATUS_SUCCESS; 00105 00106 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00107 00108 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00109 00110 //FIXME: add relevant error handling - general task for all APIs. 00111 //It is okay to finalize KCM here since it's already initialized beforehand. 00112 kcm_status = kcm_finalize(); 00113 if (kcm_status != KCM_STATUS_SUCCESS) { 00114 fcc_status = FCC_STATUS_ERROR; 00115 SA_PV_LOG_ERR("Failed finalizing KCM"); 00116 } 00117 00118 //Finalize output info handler 00119 fcc_clean_output_info_handler(); 00120 00121 //Finalize PAL 00122 pal_destroy(); 00123 00124 g_is_fcc_initialized = false; 00125 g_is_session_finished = true; 00126 00127 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00128 00129 return fcc_status; 00130 } 00131 00132 fcc_status_e fcc_storage_delete() 00133 { 00134 kcm_status_e status = KCM_STATUS_SUCCESS; 00135 sotp_result_e sotp_status = SOTP_SUCCESS; 00136 00137 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00138 00139 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00140 00141 status = storage_reset(); 00142 SA_PV_ERR_RECOVERABLE_RETURN_IF((status == KCM_STATUS_ESFS_ERROR), FCC_STATUS_KCM_STORAGE_ERROR, "Failed in storage_reset. got ESFS error"); 00143 SA_PV_ERR_RECOVERABLE_RETURN_IF((status != KCM_STATUS_SUCCESS), FCC_STATUS_ERROR, "Failed storage reset"); 00144 00145 sotp_status = sotp_reset(); 00146 SA_PV_ERR_RECOVERABLE_RETURN_IF((sotp_status != SOTP_SUCCESS), FCC_STATUS_STORE_ERROR, "Failed to reset sotp storage "); 00147 00148 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00149 return FCC_STATUS_SUCCESS; 00150 } 00151 00152 fcc_output_info_s* fcc_get_error_and_warning_data(void) 00153 { 00154 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00155 00156 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), NULL, "FCC not initialized"); 00157 00158 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00159 00160 return get_output_info(); 00161 } 00162 00163 bool fcc_is_session_finished(void) 00164 { 00165 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00166 00167 return g_is_session_finished; 00168 } 00169 00170 fcc_status_e fcc_verify_device_configured_4mbed_cloud(void) 00171 { 00172 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00173 bool use_bootstrap = false; 00174 bool success = false; 00175 00176 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00177 00178 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00179 00180 /*Initialize fcc_output_info_s structure. 00181 In case output indo struct is not empty in the beginning of the verify process we will clean it.*/ 00182 fcc_clean_output_info_handler(); 00183 00184 //Check entropy initialization 00185 success = fcc_is_entropy_initialized(); 00186 SA_PV_ERR_RECOVERABLE_RETURN_IF((success != true), fcc_status = FCC_STATUS_ENTROPY_ERROR, "Entropy is not initialized"); 00187 00188 //Check time synchronization 00189 fcc_status = fcc_check_time_synchronization(); 00190 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to check time synhronization"); 00191 00192 //Get bootstrap mode 00193 fcc_status = fcc_get_bootstrap_mode(&use_bootstrap); 00194 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to get bootstrap mode"); 00195 00196 // Check general info 00197 fcc_status = fcc_check_device_general_info(); 00198 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to check general info"); 00199 00200 //Check device meta-data 00201 fcc_status = fcc_check_device_meta_data(); 00202 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to check configuration parameters"); 00203 00204 //Check device security objects 00205 fcc_status = fcc_check_device_security_objects(use_bootstrap); 00206 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to check device security objects"); 00207 00208 //Check firmware integrity 00209 fcc_status = fcc_check_firmware_update_integrity(); 00210 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to check device security objects"); 00211 00212 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00213 00214 return fcc_status; 00215 } 00216 00217 fcc_status_e fcc_entropy_set(const uint8_t *buf, size_t buf_size) 00218 { 00219 fcc_status_e fcc_status; 00220 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00221 00222 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00223 00224 fcc_status = fcc_sotp_data_store(buf, buf_size, SOTP_TYPE_RANDOM_SEED); 00225 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status == FCC_STATUS_INTERNAL_ITEM_ALREADY_EXIST), FCC_STATUS_ENTROPY_ERROR, "Entropy already exist in storage"); 00226 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to set entropy"); 00227 00228 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00229 return FCC_STATUS_SUCCESS; 00230 } 00231 00232 fcc_status_e fcc_rot_set(const uint8_t *buf, size_t buf_size) 00233 { 00234 fcc_status_e fcc_status; 00235 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00236 00237 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00238 00239 fcc_status = fcc_sotp_data_store(buf, buf_size, SOTP_TYPE_ROT); 00240 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status == FCC_STATUS_INTERNAL_ITEM_ALREADY_EXIST), FCC_STATUS_ROT_ERROR, "RoT already exist in storage"); 00241 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to set RoT"); 00242 00243 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00244 return FCC_STATUS_SUCCESS; 00245 } 00246 00247 fcc_status_e fcc_time_set(uint64_t time) 00248 { 00249 palStatus_t pal_status; 00250 00251 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00252 00253 pal_status = pal_osSetStrongTime(time); 00254 SA_PV_ERR_RECOVERABLE_RETURN_IF((pal_status != PAL_SUCCESS), FCC_STATUS_ERROR, "Failed to set new EPOCH time (pal_status = %" PRIu32 ")", pal_status); 00255 00256 return FCC_STATUS_SUCCESS; 00257 } 00258 00259 fcc_status_e fcc_is_factory_disabled(bool *is_factory_disabled) 00260 { 00261 fcc_status_e fcc_status; 00262 int64_t factory_disable_flag = 0; 00263 size_t data_actual_size_out = 0; 00264 00265 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00266 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00267 SA_PV_ERR_RECOVERABLE_RETURN_IF((is_factory_disabled == NULL), FCC_STATUS_INVALID_PARAMETER, "Invalid param is_factory_disabled"); 00268 00269 fcc_status = fcc_sotp_data_retrieve((uint8_t *)(&factory_disable_flag), sizeof(factory_disable_flag), &data_actual_size_out, SOTP_TYPE_FACTORY_DONE); 00270 SA_PV_LOG_INFO("fcc_status: %d, factory_disable_flag:%" PRIuMAX "\n", fcc_status, factory_disable_flag); 00271 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS && fcc_status != FCC_STATUS_ITEM_NOT_EXIST), fcc_status, "Failed for fcc_sotp_buffer_retrieve"); 00272 SA_PV_ERR_RECOVERABLE_RETURN_IF(((factory_disable_flag != 0) && (factory_disable_flag != 1)), FCC_STATUS_FACTORY_DISABLED_ERROR, "Failed for fcc_sotp_buffer_retrieve"); 00273 00274 // If we get here - it must be either "0" or "1" 00275 *is_factory_disabled = (factory_disable_flag == 1) ? true : false; 00276 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00277 return FCC_STATUS_SUCCESS; 00278 } 00279 00280 00281 fcc_status_e fcc_factory_disable(void) 00282 { 00283 fcc_status_e fcc_status; 00284 int64_t factory_disable_flag = 1; 00285 00286 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00287 00288 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00289 00290 fcc_status = fcc_sotp_data_store((uint8_t *)(&factory_disable_flag), sizeof(factory_disable_flag), SOTP_TYPE_FACTORY_DONE); 00291 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status == FCC_STATUS_INTERNAL_ITEM_ALREADY_EXIST), FCC_STATUS_FACTORY_DISABLED_ERROR, "FCC already disabled in storage"); 00292 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed for fcc_sotp_buffer_store"); 00293 00294 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00295 return FCC_STATUS_SUCCESS; 00296 } 00297 00298 00299 fcc_status_e fcc_trust_ca_cert_id_set(void) 00300 { 00301 fcc_status_e fcc_status = FCC_STATUS_SUCCESS; 00302 fcc_status_e output_info_fcc_status = FCC_STATUS_SUCCESS; 00303 uint8_t attribute_data[PAL_CERT_ID_SIZE] = {0}; 00304 size_t size_of_attribute_data = 0; 00305 bool use_bootstrap = false; 00306 00307 SA_PV_LOG_INFO_FUNC_ENTER_NO_ARGS(); 00308 00309 SA_PV_ERR_RECOVERABLE_RETURN_IF((!g_is_fcc_initialized), FCC_STATUS_NOT_INITIALIZED, "FCC not initialized"); 00310 00311 fcc_status = fcc_get_bootstrap_mode(&use_bootstrap); 00312 SA_PV_ERR_RECOVERABLE_RETURN_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status, "Failed to get bootstrap mode"); 00313 00314 00315 //For now this API relevant only for bootstrap certificate. 00316 if (use_bootstrap == true) { 00317 fcc_status = fcc_get_certificate_attribute_by_name((const uint8_t*)g_fcc_bootstrap_server_ca_certificate_name, 00318 (size_t)(strlen(g_fcc_bootstrap_server_ca_certificate_name)), 00319 CS_CERT_ID_ATTR, 00320 attribute_data, 00321 sizeof(attribute_data), 00322 &size_of_attribute_data); 00323 SA_PV_ERR_RECOVERABLE_GOTO_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status = fcc_status, exit, "Failed to get ca id"); 00324 00325 fcc_status = fcc_sotp_data_store(attribute_data, size_of_attribute_data, SOTP_TYPE_TRUSTED_TIME_SRV_ID); 00326 SA_PV_ERR_RECOVERABLE_GOTO_IF((fcc_status == FCC_STATUS_INTERNAL_ITEM_ALREADY_EXIST), (fcc_status = FCC_STATUS_CA_ERROR), exit, "CA already exist in storage"); 00327 SA_PV_ERR_RECOVERABLE_GOTO_IF((fcc_status != FCC_STATUS_SUCCESS), fcc_status = fcc_status, exit, "Failed to set ca id"); 00328 } 00329 00330 exit: 00331 if (fcc_status != FCC_STATUS_SUCCESS) { 00332 output_info_fcc_status = fcc_store_error_info((const uint8_t*)g_fcc_bootstrap_server_ca_certificate_name, strlen(g_fcc_bootstrap_server_ca_certificate_name), fcc_status); 00333 SA_PV_ERR_RECOVERABLE_RETURN_IF((output_info_fcc_status != FCC_STATUS_SUCCESS), 00334 fcc_status = FCC_STATUS_OUTPUT_INFO_ERROR, 00335 "Failed to set ca identifier error %d", 00336 fcc_status); 00337 } 00338 00339 SA_PV_LOG_INFO_FUNC_EXIT_NO_ARGS(); 00340 return fcc_status; 00341 }
Generated on Tue Jul 12 2022 19:12:12 by
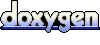