
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
TestProductionCode.c
00001 00002 #include "ProductionCode.h" 00003 #include "unity.h" 00004 00005 //sometimes you may want to get at local data in a module. 00006 //for example: If you plan to pass by reference, this could be useful 00007 //however, it should often be avoided 00008 extern int Counter; 00009 00010 void setUp(void) 00011 { 00012 //This is run before EACH TEST 00013 Counter = 0x5a5a; 00014 } 00015 00016 void tearDown(void) 00017 { 00018 } 00019 00020 void test_FindFunction_WhichIsBroken_ShouldReturnZeroIfItemIsNotInList_WhichWorksEvenInOurBrokenCode(void) 00021 { 00022 //All of these should pass 00023 TEST_ASSERT_EQUAL(0, FindFunction_WhichIsBroken(78)); 00024 TEST_ASSERT_EQUAL(0, FindFunction_WhichIsBroken(1)); 00025 TEST_ASSERT_EQUAL(0, FindFunction_WhichIsBroken(33)); 00026 TEST_ASSERT_EQUAL(0, FindFunction_WhichIsBroken(999)); 00027 TEST_ASSERT_EQUAL(0, FindFunction_WhichIsBroken(-1)); 00028 } 00029 00030 void test_FindFunction_WhichIsBroken_ShouldReturnTheIndexForItemsInList_WhichWillFailBecauseOurFunctionUnderTestIsBroken(void) 00031 { 00032 // You should see this line fail in your test summary 00033 TEST_ASSERT_EQUAL(1, FindFunction_WhichIsBroken(34)); 00034 00035 // Notice the rest of these didn't get a chance to run because the line above failed. 00036 // Unit tests abort each test function on the first sign of trouble. 00037 // Then NEXT test function runs as normal. 00038 TEST_ASSERT_EQUAL(8, FindFunction_WhichIsBroken(8888)); 00039 } 00040 00041 void test_FunctionWhichReturnsLocalVariable_ShouldReturnTheCurrentCounterValue(void) 00042 { 00043 //This should be true because setUp set this up for us before this test 00044 TEST_ASSERT_EQUAL_HEX(0x5a5a, FunctionWhichReturnsLocalVariable()); 00045 00046 //This should be true because we can still change our answer 00047 Counter = 0x1234; 00048 TEST_ASSERT_EQUAL_HEX(0x1234, FunctionWhichReturnsLocalVariable()); 00049 } 00050 00051 void test_FunctionWhichReturnsLocalVariable_ShouldReturnTheCurrentCounterValueAgain(void) 00052 { 00053 //This should be true again because setup was rerun before this test (and after we changed it to 0x1234) 00054 TEST_ASSERT_EQUAL_HEX(0x5a5a, FunctionWhichReturnsLocalVariable()); 00055 } 00056 00057 void test_FunctionWhichReturnsLocalVariable_ShouldReturnCurrentCounter_ButFailsBecauseThisTestIsActuallyFlawed(void) 00058 { 00059 //Sometimes you get the test wrong. When that happens, you get a failure too... and a quick look should tell 00060 // you what actually happened...which in this case was a failure to setup the initial condition. 00061 TEST_ASSERT_EQUAL_HEX(0x1234, FunctionWhichReturnsLocalVariable()); 00062 }
Generated on Tue Jul 12 2022 19:12:16 by
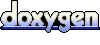