
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
eventdata.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef EVENT_DATA_H 00017 #define EVENT_DATA_H 00018 00019 #include "mbed-client/m2mvector.h" 00020 00021 //FORWARD DECLARATION 00022 class M2MObject; 00023 00024 00025 typedef Vector<M2MObject *> M2MObjectList; 00026 00027 class M2MSecurity; 00028 00029 class EventData 00030 { 00031 public: 00032 virtual ~EventData() {} 00033 }; 00034 00035 class M2MSecurityData : public EventData 00036 { 00037 public: 00038 M2MSecurityData() 00039 :_object(NULL){} 00040 virtual ~M2MSecurityData() {} 00041 M2MSecurity *_object; 00042 }; 00043 00044 class ResolvedAddressData : public EventData 00045 { 00046 public: 00047 ResolvedAddressData() 00048 :_address(NULL), 00049 _port(0){} 00050 virtual ~ResolvedAddressData() {} 00051 const M2MConnectionObserver::SocketAddress *_address; 00052 uint16_t _port; 00053 }; 00054 00055 class ReceivedData : public EventData 00056 { 00057 public: 00058 ReceivedData() 00059 :_data(NULL), 00060 _size(0), 00061 _port(0), 00062 _address(NULL){} 00063 virtual ~ReceivedData() {} 00064 uint8_t *_data; 00065 uint16_t _size; 00066 uint16_t _port; 00067 const M2MConnectionObserver::SocketAddress *_address; 00068 }; 00069 00070 class M2MRegisterData : public EventData 00071 { 00072 public: 00073 M2MRegisterData() 00074 :_object(NULL){} 00075 virtual ~M2MRegisterData() {} 00076 M2MSecurity *_object; 00077 M2MBaseList _base_list; 00078 }; 00079 00080 class M2MUpdateRegisterData : public EventData 00081 { 00082 public: 00083 M2MUpdateRegisterData() 00084 :_object(NULL), 00085 _lifetime(0){} 00086 virtual ~M2MUpdateRegisterData() {} 00087 M2MSecurity *_object; 00088 uint32_t _lifetime; 00089 M2MBaseList _base_list; 00090 }; 00091 00092 00093 #endif //EVENT_DATA_H 00094
Generated on Tue Jul 12 2022 19:12:12 by
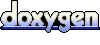