
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
eventOS_scheduler.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef EVENTOS_SCHEDULER_H_ 00017 #define EVENTOS_SCHEDULER_H_ 00018 00019 #ifdef __cplusplus 00020 extern "C" { 00021 #endif 00022 #include "ns_types.h" 00023 00024 /* Compatibility with older ns_types.h */ 00025 #ifndef NS_NORETURN 00026 #define NS_NORETURN 00027 #endif 00028 00029 /** 00030 * \brief Initialise event scheduler. 00031 * 00032 */ 00033 extern void eventOS_scheduler_init(void); 00034 00035 /** 00036 * Process one event from event queue. 00037 * Do not call this directly from application. Requires to be public so that simulator can call this. 00038 * Use eventOS_scheduler_run() or eventOS_scheduler_run_until_idle(). 00039 * \return true If there was event processed, false if the event queue was empty. 00040 */ 00041 bool eventOS_scheduler_dispatch_event(void); 00042 00043 /** 00044 * \brief Process events until no more events to process. 00045 */ 00046 extern void eventOS_scheduler_run_until_idle(void); 00047 00048 /** 00049 * \brief Start Event scheduler. 00050 * Loops forever processing events from the queue. 00051 * Calls eventOS_scheduler_idle() whenever event queue is empty. 00052 */ 00053 NS_NORETURN extern void eventOS_scheduler_run(void); 00054 /** 00055 * \brief Disable Event scheduler Timers 00056 * 00057 * \return 0 Timer Stop OK 00058 * \return -1 Timer Stop Fail 00059 * 00060 * */ 00061 int eventOS_scheduler_timer_stop(void); 00062 00063 /** 00064 * \brief Synch Event scheduler timer after sleep 00065 * 00066 * \param sleep_ticks time in milli seconds 00067 * 00068 * \return 0 Timer Synch OK 00069 * \return -1 Timer Synch & Start Fail 00070 * 00071 * */ 00072 int eventOS_scheduler_timer_synch_after_sleep(uint32_t sleep_ticks); 00073 00074 /** 00075 * \brief Read current active Tasklet ID 00076 * 00077 * This function not return valid information called inside interrupt 00078 * 00079 * \return curret active tasklet id 00080 * 00081 * */ 00082 extern int8_t eventOS_scheduler_get_active_tasklet(void); 00083 00084 /** 00085 * \brief Set manually Active Tasklet ID 00086 * 00087 * \param tasklet requested tasklet ID 00088 * 00089 * */ 00090 extern void eventOS_scheduler_set_active_tasklet(int8_t tasklet); 00091 00092 /** 00093 * \brief Event scheduler loop idle Callback. 00094 00095 * Note! This method is called only by eventOS_scheduler_run, needs to be 00096 * ported for the platform only if you are using eventOS_scheduler_run(). 00097 */ 00098 extern void eventOS_scheduler_idle(void); 00099 00100 /** 00101 * \brief This function will be called when stack enter idle state and start 00102 * waiting signal. 00103 * 00104 * Note! This method is called only by reference implementation of idle. Needs 00105 * to be ported for the platform only if you are using reference implementation. 00106 */ 00107 extern void eventOS_scheduler_wait(void); 00108 00109 /** 00110 * \brief This function will be called when stack receives an event. 00111 */ 00112 extern void eventOS_scheduler_signal(void); 00113 00114 /** 00115 * \brief This function will be called when stack can enter deep sleep state in detected time. 00116 * 00117 * Note! This method is called only by reference implementation of idle. Needs to be 00118 * ported for the platform only if you are using reference implementation. 00119 * 00120 * \param sleep_time_ms Time in milliseconds to sleep 00121 * \return time slept in milliseconds 00122 */ 00123 extern uint32_t eventOS_scheduler_sleep(uint32_t sleep_time_ms); 00124 00125 /** 00126 * \brief Lock a thread against the event loop thread 00127 * 00128 * This method can be provided by multi-threaded platforms to allow 00129 * mutual exclusion with the event loop thread, for cases where 00130 * code wants to work with both the event loop and other threads. 00131 * 00132 * A typical platform implementation would claim the same mutex 00133 * before calling eventOS_scheduler_run() or 00134 * eventOS_scheduler_dispatch(), and release it during 00135 * eventOS_scheduler_idle(). 00136 * 00137 * The mutex must count - nested calls from one thread return 00138 * immediately. Thus calling this from inside an event callback 00139 * is harmless. 00140 */ 00141 extern void eventOS_scheduler_mutex_wait(void); 00142 00143 /** 00144 * \brief Release the event loop mutex 00145 * 00146 * Release the mutex claimed with eventOS_scheduler_mutex_wait(), 00147 * allowing the event loop to continue processing. 00148 */ 00149 extern void eventOS_scheduler_mutex_release(void); 00150 00151 /** 00152 * \brief Check if the current thread owns the event mutex 00153 * 00154 * Check if the calling thread owns the scheduler mutex. 00155 * This allows the ownership to be asserted if a function 00156 * requires the mutex to be locked externally. 00157 * 00158 * The function is only intended as a debugging aid for 00159 * users of eventOS_scheduler_mutex_wait() - it is not 00160 * used by the event loop core itself. 00161 * 00162 * If the underlying mutex system does not support it, 00163 * this may be implemented to always return true. 00164 * 00165 * \return true if the current thread owns the mutex 00166 */ 00167 extern bool eventOS_scheduler_mutex_am_owner(void); 00168 00169 #ifdef __cplusplus 00170 } 00171 #endif 00172 00173 #endif /* EVENTOS_SCHEDULER_H_ */
Generated on Tue Jul 12 2022 19:12:12 by
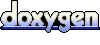