
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
eventOS_event_timer.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef EVENTOS_EVENT_TIMER_H_ 00017 #define EVENTOS_EVENT_TIMER_H_ 00018 #ifdef __cplusplus 00019 extern "C" { 00020 #endif 00021 #include "ns_types.h" 00022 #include "eventOS_event.h" 00023 00024 struct arm_event_s; 00025 typedef struct sys_timer_struct_s sys_timer_struct_t; 00026 00027 /* 100 Hz ticker, so 10 milliseconds per tick */ 00028 #define EVENTOS_EVENT_TIMER_HZ 100 00029 00030 static inline uint32_t eventOS_event_timer_ticks_to_ms(uint32_t ticks) 00031 { 00032 NS_STATIC_ASSERT(1000 % EVENTOS_EVENT_TIMER_HZ == 0, "Assuming whole number of ms per tick") 00033 return ticks * (1000 / EVENTOS_EVENT_TIMER_HZ); 00034 } 00035 00036 /* Convert ms to ticks, rounding up (so 9ms = 1 tick, 10ms = 1 tick, 11ms = 2 ticks) */ 00037 static inline uint32_t eventOS_event_timer_ms_to_ticks(uint32_t ms) 00038 { 00039 NS_STATIC_ASSERT(1000 % EVENTOS_EVENT_TIMER_HZ == 0, "Assuming whole number of ms per tick") 00040 return (ms + (1000 / EVENTOS_EVENT_TIMER_HZ) - 1) / (1000 / EVENTOS_EVENT_TIMER_HZ); 00041 } 00042 00043 /** 00044 * Read current timer tick count. 00045 * 00046 * Can be used as a monotonic time source, and to schedule events with 00047 * eventOS_event_timer_send. 00048 * 00049 * Note that the value will wrap, so take care on comparisons. 00050 * 00051 * \return tick count. 00052 */ 00053 extern uint32_t eventOS_event_timer_ticks(void); 00054 00055 /* Comparison macros handling wrap efficiently (assuming a conventional compiler 00056 * which converts 0x80000000 to 0xFFFFFFFF to negative when casting to int32_t). 00057 */ 00058 #define TICKS_AFTER(a, b) ((int32_t) ((a)-(b)) > 0) 00059 #define TICKS_BEFORE(a, b) ((int32_t) ((a)-(b)) < 0) 00060 #define TICKS_AFTER_OR_AT(a, b) ((int32_t) ((a)-(b)) >= 0) 00061 #define TICKS_BEFORE_OR_AT(a, b) ((int32_t) ((a)-(b)) <= 0) 00062 00063 /** 00064 * Send an event after time expired (in milliseconds) 00065 * 00066 * Note that the current implementation has the "feature" that rounding 00067 * varies depending on the precise timing requested: 00068 * 0-20 ms => 2 x 10ms tick 00069 * 21-29 ms => 3 x 10ms tick 00070 * 30-39 ms => 4 x 10ms tick 00071 * 40-49 ms => 5 x 10ms tick 00072 * ... etc 00073 * 00074 * For improved flexibility on the event, and for more control of time, 00075 * you should use eventOS_event_timer_request_at(). 00076 * 00077 * \param event_id event_id for event 00078 * \param event_type event_type for event 00079 * \param tasklet_id receiver for event 00080 * \param time time to sleep in milliseconds 00081 * 00082 * \return 0 on success 00083 * \return -1 on error (invalid tasklet_id or allocation failure) 00084 * 00085 * */ 00086 extern int8_t eventOS_event_timer_request(uint8_t event_id, uint8_t event_type, int8_t tasklet_id, uint32_t time); 00087 00088 /** 00089 * Send an event at specified time 00090 * 00091 * The event will be sent when eventOS_event_timer_ticks() reaches the 00092 * specified value. 00093 * 00094 * If the specified time is in the past (ie "at" is before or at the current 00095 * tick value), the event will be sent immediately. 00096 * 00097 * Can also be invoked using the eventOS_event_send_at() macro in eventOS_event.h 00098 * 00099 * \param event event to send 00100 * \param at absolute tick time to run event at 00101 * 00102 * \return pointer to timer structure on success 00103 * \return NULL on error (invalid tasklet_id or allocation failure) 00104 * 00105 */ 00106 extern arm_event_storage_t *eventOS_event_timer_request_at(const struct arm_event_s *event, uint32_t at); 00107 00108 /** 00109 * Send an event in a specified time 00110 * 00111 * The event will be sent in the specified number of ticks - to 00112 * be precise, it is equivalent to requesting an event at 00113 * 00114 * eventOS_event_timer_ticks() + ticks 00115 * 00116 * Because of timer granularity, the elapsed time between issuing the request 00117 * and it running may be up to 1 tick less than the specified time. 00118 * 00119 * eg requesting 2 ticks will cause the event to be sent on the second tick from 00120 * now. If requested just after a tick, the delay will be nearly 2 ticks, but if 00121 * requested just before a tick, the delay will be just over 1 tick. 00122 * 00123 * If `in` is <= 0, the event will be sent immediately. 00124 * 00125 * Can also be invoked using the eventOS_event_send_in() macro in eventOS_event.h 00126 * 00127 * \param event event to send 00128 * \param in tick delay for event 00129 * 00130 * \return pointer to timer structure on success 00131 * \return NULL on error (invalid tasklet_id or allocation failure) 00132 * 00133 */ 00134 extern arm_event_storage_t *eventOS_event_timer_request_in(const struct arm_event_s *event, int32_t in); 00135 00136 /** 00137 * Send an event after a specified time 00138 * 00139 * The event will be sent after the specified number of ticks - to 00140 * be precise, it is equivalent to requesting an event at 00141 * 00142 * eventOS_event_timer_ticks() + ticks + 1 00143 * 00144 * Because of timer granularity, the elapsed time between issuing the request 00145 * and it running may be up to 1 tick more than the specified time. 00146 * 00147 * eg requesting 2 ticks will cause the event to be sent on the third tick from 00148 * now. If requested just after a tick, the delay will be nearly 3 ticks, but if 00149 * requested just before a tick, the delay will be just over 2 ticks. 00150 * 00151 * If `after` is < 0, the event will be sent immediately. If it is 0, the event 00152 * is sent on the next tick. 00153 * 00154 * Can also be invoked using the eventOS_event_send_after() macro in eventOS_event.h 00155 * 00156 * \param event event to send 00157 * \param after tick delay for event 00158 * 00159 * \return pointer to timer structure on success 00160 * \return NULL on error (invalid tasklet_id or allocation failure) 00161 * 00162 */ 00163 #define eventOS_event_timer_request_after(event, after) \ 00164 eventOS_event_timer_request_in(event, (after) + 1) 00165 00166 /** 00167 * Send an event periodically 00168 * 00169 * The event will be sent repeatedly using the specified ticks period. 00170 * 00171 * The first call is sent at 00172 * 00173 * eventOS_event_timer_ticks() + ticks 00174 * 00175 * Subsequent events will be sent at N*ticks from the initial time. 00176 * 00177 * Period will be maintained while the device is awake, regardless of delays to 00178 * event scheduling. If an event has not been delivered and completed by the 00179 * next scheduled time, the next event will be sent immediately when it 00180 * finishes. This could cause a continuous stream of events if unable to keep 00181 * up with the period. 00182 * 00183 * Can also be invoked using the eventOS_event_send_every() macro in eventOS_event.h 00184 * 00185 * \param event event to send 00186 * \param period period for event 00187 * 00188 * \return pointer to timer structure on success 00189 * \return NULL on error (invalid tasklet_id or allocation failure) 00190 * 00191 */ 00192 extern arm_event_storage_t *eventOS_event_timer_request_every(const struct arm_event_s *event, int32_t period); 00193 00194 /** 00195 * Cancel an event timer 00196 * 00197 * This cancels a pending timed event, matched by event_id and tasklet_id. 00198 * 00199 * \param event_id event_id for event 00200 * \param tasklet_id receiver for event 00201 * 00202 * \return 0 on success 00203 * \return -1 on error (event not found) 00204 * 00205 * */ 00206 extern int8_t eventOS_event_timer_cancel(uint8_t event_id, int8_t tasklet_id); 00207 00208 /** 00209 * System Timer shortest time in milli seconds 00210 * 00211 * \param ticks Time in 10 ms resolution 00212 * 00213 * \return none 00214 * 00215 * */ 00216 extern uint32_t eventOS_event_timer_shortest_active_timer(void); 00217 00218 00219 /** Timeout structure. Not to be modified by user */ 00220 typedef struct timeout_entry_t timeout_t; 00221 00222 /** Request timeout callback. 00223 * 00224 * Create timeout request for specific callback. 00225 * 00226 * \param ms timeout in milliseconds. Maximum range is same as for eventOS_event_timer_request(). 00227 * \param callback function to call after timeout 00228 * \param arg arquement to pass to callback 00229 * \return pointer to timeout structure or NULL on errors 00230 */ 00231 timeout_t *eventOS_timeout_ms(void (*callback)(void *), uint32_t ms, void *arg); 00232 00233 /** Request periodic callback. 00234 * 00235 * Create timeout request for specific callback. Called periodically until eventOS_timeout_cancel() is called. 00236 * 00237 * \param every period in milliseconds. Maximum range is same as for eventOS_event_timer_request(). 00238 * \param callback function to call after timeout 00239 * \param arg arquement to pass to callback 00240 * \return pointer to timeout structure or NULL on errors 00241 */ 00242 timeout_t *eventOS_timeout_every_ms(void (*callback)(void *), uint32_t every, void *arg); 00243 00244 /** Cancell timeout request. 00245 * 00246 * \param t timeout request id. 00247 */ 00248 void eventOS_timeout_cancel(timeout_t *t); 00249 00250 00251 #ifdef __cplusplus 00252 } 00253 #endif 00254 00255 #endif /* EVENTOS_EVENT_TIMER_H_ */
Generated on Tue Jul 12 2022 19:12:12 by
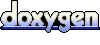