
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
eventOS_event.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef EVENTOS_EVENT_H_ 00017 #define EVENTOS_EVENT_H_ 00018 #ifdef __cplusplus 00019 extern "C" { 00020 #endif 00021 00022 #include "ns_types.h" 00023 #include "ns_list.h" 00024 00025 /** 00026 * \enum arm_library_event_priority_e 00027 * \brief Event Priority level. 00028 */ 00029 typedef enum arm_library_event_priority_e { 00030 ARM_LIB_HIGH_PRIORITY_EVENT = 0, /**< High Priority Event (Function CB) */ 00031 ARM_LIB_MED_PRIORITY_EVENT = 1, /**< Medium Priority (Timer) */ 00032 ARM_LIB_LOW_PRIORITY_EVENT = 2, /*!*< Normal Event and ECC / Security */ 00033 } arm_library_event_priority_e; 00034 00035 /** 00036 * \struct arm_event_s 00037 * \brief Event structure. 00038 */ 00039 typedef struct arm_event_s { 00040 int8_t receiver; /**< Event handler Tasklet ID */ 00041 int8_t sender; /**< Event sender Tasklet ID */ 00042 uint8_t event_type; /**< This will be typecast arm_library_event_type_e, arm_internal_event_type_e or application specific define */ 00043 uint8_t event_id; /**< Timer ID, NWK interface ID or application specific ID */ 00044 void *data_ptr; /**< Application could share data pointer tasklet to tasklet */ 00045 arm_library_event_priority_e priority; 00046 uint32_t event_data; 00047 } arm_event_t; 00048 00049 /* Backwards compatibility */ 00050 typedef arm_event_t arm_event_s; 00051 00052 /** 00053 * \struct arm_event_storage 00054 * \brief Event structure storage, including list link. 00055 00056 @startuml 00057 00058 partition "Event loop" { 00059 (*) -->[event created] "UNQUEUED" 00060 "UNQUEUED" -->[event_core_write()] "QUEUED" 00061 "QUEUED" -->[event_core_read()] "RUNNING" 00062 "RUNNING" ->[event_core_free_push()] "UNQUEUED" 00063 } 00064 00065 partition "system_timer.c" { 00066 "UNQUEUED:timer" -->[eventOS_event_send_timer_allocated()] "QUEUED" 00067 } 00068 @enduml 00069 00070 */ 00071 typedef struct arm_event_storage { 00072 arm_event_s data; 00073 enum { 00074 ARM_LIB_EVENT_STARTUP_POOL, 00075 ARM_LIB_EVENT_DYNAMIC, 00076 ARM_LIB_EVENT_USER, 00077 ARM_LIB_EVENT_TIMER, 00078 } allocator; 00079 enum { 00080 ARM_LIB_EVENT_UNQUEUED, 00081 ARM_LIB_EVENT_QUEUED, 00082 ARM_LIB_EVENT_RUNNING, 00083 } state; 00084 ns_list_link_t link; 00085 } arm_event_storage_t; 00086 00087 /** 00088 * \brief Send event to event scheduler. 00089 * 00090 * \param event pointer to pushed event. 00091 * 00092 * Event data is copied by the call, and this copy persists until the 00093 * recipient's callback function returns. The callback function is passed 00094 * a pointer to a copy of the data, not the original pointer. 00095 * 00096 * \return 0 Event push OK 00097 * \return -1 Memory allocation Fail 00098 */ 00099 extern int8_t eventOS_event_send(const arm_event_t *event); 00100 00101 /* Alternate names for timer function from eventOS_event_timer.h; 00102 * implementations may one day merge */ 00103 #define eventOS_event_send_at(event, at) eventOS_event_timer_request_at(event, at) 00104 #define eventOS_event_send_in(event, in) eventOS_event_timer_request_in(event, in) 00105 #define eventOS_event_send_after(event, after) eventOS_event_timer_request_after(event, after) 00106 #define eventOS_event_send_every(event, every) eventOS_event_timer_request_every(event, every) 00107 00108 /** 00109 * \brief Send user-allocated event to event scheduler. 00110 * 00111 * \param event pointer to pushed event storage. 00112 * 00113 * The event structure is not copied by the call, the event system takes 00114 * ownership and it is threaded directly into the event queue. This avoids the 00115 * possibility of event sending failing due to memory exhaustion. 00116 * 00117 * event->data must be filled in on entry - the rest of the structure (link and 00118 * allocator) need not be. 00119 * 00120 * The structure must remain valid until the recipient is called - the 00121 * event system passes ownership to the receiving event handler, who may then 00122 * invalidate it, or send it again. 00123 * 00124 * The recipient receives a pointer to the arm_event_t data member of the 00125 * event - it can use NS_CONTAINER_OF() to get a pointer to the original 00126 * event passed to this call, or to its outer container. 00127 * 00128 * It is a program error to send a user-allocated event to a non-existent task. 00129 */ 00130 extern void eventOS_event_send_user_allocated(arm_event_storage_t *event); 00131 00132 /** 00133 * \brief Event handler callback register 00134 * 00135 * Function will register and allocate unique event id handler 00136 * 00137 * \param handler_func_ptr function pointer for event handler 00138 * \param init_event_type generated event type for init purpose 00139 * 00140 * \return >= 0 Unique event ID for this handler 00141 * \return < 0 Register fail 00142 * 00143 * */ 00144 extern int8_t eventOS_event_handler_create(void (*handler_func_ptr)(arm_event_t *), uint8_t init_event_type); 00145 00146 /** 00147 * Cancel an event. 00148 * 00149 * Queued events are removed from the event-loop queue and/or the timer queue. 00150 * 00151 * Passing a NULL pointer is allowed, and does nothing. 00152 * 00153 * Event pointers are valid from the time they are queued until the event 00154 * has finished running or is cancelled. 00155 * 00156 * Cancelling a currently-running event is only useful to stop scheduling 00157 * it if it is on a periodic timer; it has no other effect. 00158 * 00159 * Cancelling an already-cancelled or already-run single-shot event 00160 * is undefined behaviour. 00161 * 00162 * \param event Pointer to event handle or NULL. 00163 */ 00164 extern void eventOS_cancel(arm_event_storage_t *event); 00165 00166 #ifdef __cplusplus 00167 } 00168 #endif 00169 #endif /* EVENTOS_EVENT_H_ */
Generated on Tue Jul 12 2022 19:12:12 by
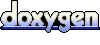