
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_source_manager.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef ARM_UC_SOURCE_MANAGER_H 00020 #define ARM_UC_SOURCE_MANAGER_H 00021 00022 #include "update-client-common/arm_uc_common.h" 00023 #include "update-client-source/arm_uc_source.h" 00024 00025 typedef enum { 00026 ARM_UC_SM_EVENT_NOTIFICATION, 00027 ARM_UC_SM_EVENT_MANIFEST, 00028 ARM_UC_SM_EVENT_FIRMWARE, 00029 ARM_UC_SM_EVENT_KEYTABLE, 00030 ARM_UC_SM_EVENT_ERROR, 00031 ARM_UC_SM_EVENT_ERROR_BUFFER_SIZE 00032 } ARM_UC_SM_Event_t; 00033 00034 typedef struct _ARM_UC_SOURCE_MANAGER { 00035 00036 /** 00037 * @brief Initialize module and register event handler. 00038 * @details The event handler is shared among all asynchronous calls. 00039 * 00040 * @param callback Function pointer to event handler. 00041 * @return Error code. 00042 */ 00043 arm_uc_error_t (*Initialize)(ARM_SOURCE_SignalEvent_t event_cb); 00044 arm_uc_error_t (*Uninitialize)(void); 00045 00046 /** 00047 * @brief Add firmware source to manager. 00048 * @details Each source is represented as a pointer to a struct, containing 00049 * function pointers. 00050 * 00051 * For example: 00052 * typedef struct _ARM_UPDATE_SOURCE { 00053 * ARM_DRIVER_VERSION (*GetVersion) (void); 00054 * ARM_SOURC_CAPABILITIES (*GetCapabilities)(void); 00055 * int32_t (*Initialize) (ARM_SOURCE_SignalEvent_t event); 00056 * } ARM_UPDATE_SOURCE; 00057 * 00058 * @param source Collection of function pointers to source. 00059 * @return Error code. 00060 */ 00061 arm_uc_error_t (*AddSource)(const ARM_UPDATE_SOURCE* source); 00062 arm_uc_error_t (*RemoveSource)(const ARM_UPDATE_SOURCE* source); 00063 00064 /** 00065 * @brief Copy manifest into provided buffer. 00066 * @details Default manifest location is used. An event is generated when the 00067 * manifest has been received. 00068 * 00069 * @param buffer Struct holding a byte array, maximum size, and actual size. 00070 * 00071 * @return Error code. 00072 */ 00073 arm_uc_error_t (*GetManifest)(arm_uc_buffer_t* buffer, uint32_t offset); 00074 00075 /** 00076 * @brief Copy manifest into provided buffer. 00077 * @details Manifest location is provided. An event is generated when the 00078 * manifest has been received. 00079 * 00080 * @param uri Struct containing the URI to the manifest. 00081 * @param buffer Struct holding a byte array, maximum size, and actual size. 00082 * 00083 * @return Error code. 00084 */ 00085 arm_uc_error_t (*GetManifestFrom)(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer, uint32_t offset); 00086 00087 /** 00088 * @brief Copy firmware fragment into provided buffer. 00089 * @details Firmware is downloaded one fragment at a time. Each call generates 00090 * an event when the fragment has been received. 00091 * 00092 * @param uri Struct containing the URI to the manifest. 00093 * @param buffer Struct holding a byte array, maximum size, and actual size. 00094 * @param offset Firmware offset in bytes where the next fragment begins. 00095 * @return Error code. 00096 */ 00097 arm_uc_error_t (*GetFirmwareFragment)(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer, uint32_t offset); 00098 00099 /** 00100 * @brief Retrieve key table and write it into provided buffer. 00101 * @details An event is generated when the manifest has been received. 00102 * 00103 * @param uri Struct containing the URI to the keytable. 00104 * @param buffer Struct holding a byte array, maximum size, and actual size. 00105 * @return Error code. 00106 */ 00107 arm_uc_error_t (*GetKeytable)(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer); 00108 00109 } ARM_UC_SOURCE_MANAGER_t; 00110 00111 extern ARM_UC_SOURCE_MANAGER_t ARM_UC_SourceManager; 00112 00113 /** 00114 * Usage examples 00115 * 00116 * void callback(uint32_t event) 00117 * { 00118 * switch (event) 00119 * { 00120 * // New manifest is available 00121 * case ARM_UC_SM_EVENT_NOTIFICATION: 00122 * break; 00123 * 00124 * // Manifest received from default location 00125 * case ARM_UC_SM_EVENT_MANIFEST: 00126 * break; 00127 * 00128 * // Manifest received from URL 00129 * case ARM_UC_SM_EVENT_FIRMWARE: 00130 * break; 00131 * 00132 * // Firmware fragment received 00133 * case ARM_UC_SM_EVENT_KEYTABLE: 00134 * break; 00135 * } 00136 * } 00137 * 00138 * void main(int) 00139 * { 00140 * // initialize Source Manager with callback handler 00141 * ARM_UC_SourceManager.Initialise(callback); 00142 * } 00143 * 00144 */ 00145 00146 #endif /* SOURCE_MANAGER_H */
Generated on Tue Jul 12 2022 19:12:11 by
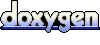