
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_pal_linux_implementation_internal.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef ARM_UC_PAL_LINUX_IMPLEMENTATION_INTERNAL_H 00020 #define ARM_UC_PAL_LINUX_IMPLEMENTATION_INTERNAL_H 00021 00022 #include "update-client-paal/arm_uc_paal_update_api.h" 00023 00024 #include <stdbool.h> 00025 #include <stdio.h> 00026 00027 #ifdef PAL_UPDATE_FIRMWARE_DIR 00028 #define ARM_UC_FIRMWARE_FOLDER_PATH PAL_UPDATE_FIRMWARE_DIR 00029 #define ARM_UC_HEADER_FOLDER_PATH PAL_UPDATE_FIRMWARE_DIR 00030 #endif 00031 00032 #ifndef ARM_UC_FIRMWARE_FOLDER_PATH 00033 #define ARM_UC_FIRMWARE_FOLDER_PATH "/tmp" 00034 #endif 00035 00036 #ifndef ARM_UC_HEADER_FOLDER_PATH 00037 #define ARM_UC_HEADER_FOLDER_PATH "/tmp" 00038 #endif 00039 00040 #ifndef ARM_UC_INSTALLER_FOLDER_PATH 00041 #define ARM_UC_INSTALLER_FOLDER_PATH "/tmp" 00042 #endif 00043 00044 #ifndef ARM_UC_USE_EXTERNAL_HEADER 00045 #define ARM_UC_USE_EXTERNAL_HEADER 0 00046 #endif 00047 00048 #define ARM_UC_MAXIMUM_FILE_AND_PATH_LENGTH 128 00049 #define ARM_UC_MAXIMUM_COMMAND_LENGTH 256 00050 00051 /* This is the type of the "post_runner" function in the worker struct below. 00052 This function is called if the worker's command executed succesfully. 00053 The callback returns the event that the worker will signal. */ 00054 typedef int32_t (*arm_ucp_c_runner_t)(); 00055 00056 typedef struct { 00057 const char* command; 00058 bool header; 00059 bool firmware; 00060 bool location; 00061 bool offset; 00062 bool size; 00063 int32_t success_event; 00064 int32_t failure_event; 00065 arm_ucp_c_runner_t post_runner; 00066 } arm_ucp_worker_t; 00067 00068 typedef struct { 00069 arm_ucp_worker_t* activate; 00070 arm_ucp_worker_t* active_details; 00071 arm_ucp_worker_t* details; 00072 arm_ucp_worker_t* finalize; 00073 arm_ucp_worker_t* initialize; 00074 arm_ucp_worker_t* installer; 00075 arm_ucp_worker_t* prepare; 00076 arm_ucp_worker_t* read; 00077 arm_ucp_worker_t* write; 00078 } arm_ucp_worker_config_t; 00079 00080 #if defined(TARGET_IS_PC_LINUX) 00081 #include <pthread.h> 00082 typedef struct LinuxWorkerThreadInfo { 00083 pthread_mutex_t mutex; 00084 pthread_t thread; 00085 pthread_attr_t attr; 00086 int attr_initialized; 00087 } linux_worker_thread_info_t; 00088 #endif 00089 00090 void arm_uc_pal_linux_signal_callback(uint32_t event, bool from_thread); 00091 00092 /* set module variables */ 00093 void arm_uc_pal_linux_internal_set_callback(ARM_UC_PAAL_UPDATE_SignalEvent_t callback); 00094 void arm_uc_pal_linux_internal_set_offset(uint32_t offset); 00095 void arm_uc_pal_linux_internal_set_buffer(arm_uc_buffer_t* buffer); 00096 void arm_uc_pal_linux_internal_set_details(arm_uc_firmware_details_t* details); 00097 void arm_uc_pal_linux_internal_set_installer(arm_uc_installer_details_t* details); 00098 void arm_uc_pal_linux_internal_set_location(uint32_t* location); 00099 00100 /* construct file path */ 00101 arm_uc_error_t arm_uc_pal_linux_internal_file_path(char* buffer, 00102 size_t buffer_length, 00103 const char* folder, 00104 const char* type, 00105 uint32_t* location); 00106 00107 /* read firmware header */ 00108 arm_uc_error_t arm_uc_pal_linux_internal_read_header(uint32_t* location, 00109 arm_uc_firmware_details_t* details); 00110 00111 /* read installer header */ 00112 arm_uc_error_t arm_uc_pal_linux_internal_read_installer(arm_uc_installer_details_t* details); 00113 00114 /* write firmware header*/ 00115 arm_uc_error_t arm_uc_pal_linux_internal_write_header(uint32_t* location, 00116 const arm_uc_firmware_details_t* details); 00117 00118 /* read file */ 00119 arm_uc_error_t arm_uc_pal_linux_internal_read(const char* file_path, 00120 uint32_t offset, 00121 arm_uc_buffer_t* buffer); 00122 00123 /** 00124 * @brief Function to run script in a worker thread before file operations. 00125 * 00126 * @param params Pointer to arm_ucp_worker_t struct. 00127 */ 00128 void* arm_uc_pal_linux_extended_pre_worker(void* params); 00129 00130 /** 00131 * @brief Function to run script in a worker thread before file operations. 00132 * 00133 * @param params Pointer to arm_ucp_worker_t struct. 00134 */ 00135 void* arm_uc_pal_linux_extended_post_worker(void* params); 00136 00137 #endif /* ARM_UC_PAL_LINUX_IMPLEMENTATION_INTERNAL_H */
Generated on Tue Jul 12 2022 19:12:11 by
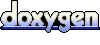