
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_pal_flashiap_mbed.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #if defined(TARGET_LIKE_MBED) 00020 00021 #include "update-client-pal-flashiap/arm_uc_pal_flashiap_platform.h" 00022 #include "mbed.h" 00023 00024 static FlashIAP flash; 00025 00026 int32_t arm_uc_flashiap_init(void) 00027 { 00028 /* Workaround for https://github.com/ARMmbed/mbed-os/issues/4967 00029 * pal_init calls flash.init() before here. Second call to flash.init() will 00030 * return -1 error state. Hence we ignore the result of flash.init here. 00031 */ 00032 flash.init(); 00033 return 0; 00034 } 00035 00036 int32_t arm_uc_flashiap_erase(uint32_t address, uint32_t size) 00037 { 00038 return flash.erase(address, size); 00039 } 00040 00041 int32_t arm_uc_flashiap_program(const uint8_t* buffer, uint32_t address, uint32_t size) 00042 { 00043 uint32_t page_size = flash.get_page_size(); 00044 int status = ARM_UC_FLASHIAP_FAIL; 00045 00046 for (uint32_t i = 0; i < size; i += page_size) 00047 { 00048 status = flash.program(buffer+i, address+i, page_size); 00049 if (status != ARM_UC_FLASHIAP_SUCCESS) 00050 { 00051 break; 00052 } 00053 } 00054 00055 return status; 00056 } 00057 00058 int32_t arm_uc_flashiap_read(uint8_t* buffer, uint32_t address, uint32_t size) 00059 { 00060 return flash.read(buffer, address, size); 00061 } 00062 00063 uint32_t arm_uc_flashiap_get_page_size(void) 00064 { 00065 return flash.get_page_size(); 00066 } 00067 00068 uint32_t arm_uc_flashiap_get_sector_size(uint32_t address) 00069 { 00070 uint32_t sector_size = flash.get_sector_size(address); 00071 if (sector_size == ARM_UC_FLASH_INVALID_SIZE || sector_size == 0) 00072 { 00073 return ARM_UC_FLASH_INVALID_SIZE; 00074 } 00075 else 00076 { 00077 return sector_size; 00078 } 00079 } 00080 00081 uint32_t arm_uc_flashiap_get_flash_size(void) 00082 { 00083 return flash.get_flash_size(); 00084 } 00085 00086 uint32_t arm_uc_flashiap_get_flash_start(void) 00087 { 00088 return flash.get_flash_start(); 00089 } 00090 #endif
Generated on Tue Jul 12 2022 19:12:11 by
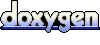