
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_pal_blockdevice_platform.h
00001 //---------------------------------------------------------------------------- 00002 // The confidential and proprietary information contained in this file may 00003 // only be used by a person authorised under and to the extent permitted 00004 // by a subsisting licensing agreement from ARM Limited or its affiliates. 00005 // 00006 // (C) COPYRIGHT 2017 ARM Limited or its affiliates. 00007 // ALL RIGHTS RESERVED 00008 // 00009 // This entire notice must be reproduced on all copies of this file 00010 // and copies of this file may only be made by a person if such person is 00011 // permitted to do so under the terms of a subsisting license agreement 00012 // from ARM Limited or its affiliates. 00013 //---------------------------------------------------------------------------- 00014 00015 #ifndef ARM_UC_PAL_BLOCKDEVICE_PLATFORM_H 00016 #define ARM_UC_PAL_BLOCKDEVICE_PLATFORM_H 00017 00018 #include <stdint.h> 00019 00020 #ifdef __cplusplus 00021 extern "C" { 00022 #endif 00023 00024 enum { 00025 ARM_UC_BLOCKDEVICE_SUCCESS = 0, 00026 ARM_UC_BLOCKDEVICE_FAIL = -1 00027 }; 00028 00029 #define ARM_UC_BLOCKDEVICE_INVALID_SIZE 0xFFFFFFFF 00030 00031 /** Initialize a block device 00032 * 00033 * @return 0 on success or a negative error code on failure 00034 */ 00035 int32_t arm_uc_blockdevice_init(void); 00036 00037 /** Erase blocks on a block device 00038 * 00039 * The state of an erased block is undefined until it has been programmed 00040 * 00041 * @param address Address of block to begin erasing 00042 * @param size Size to erase in bytes, must be a multiple of erase block size 00043 * @return 0 on success, negative error code on failure 00044 */ 00045 int32_t arm_uc_blockdevice_erase(uint64_t address, uint64_t size); 00046 00047 /** Program blocks to a block device 00048 * 00049 * The blocks must have been erased prior to being programmed 00050 * 00051 * If a failure occurs, it is not possible to determine how many bytes succeeded 00052 * 00053 * @param buffer Buffer of data to write to blocks 00054 * @param address Address of block to begin writing to 00055 * @param size Size to write in bytes, must be a multiple of program block size 00056 * @return 0 on success, negative error code on failure 00057 */ 00058 int32_t arm_uc_blockdevice_program(const uint8_t* buffer, 00059 uint64_t address, 00060 uint32_t size); 00061 00062 /** Read blocks from a block device 00063 * 00064 * If a failure occurs, it is not possible to determine how many bytes succeeded 00065 * 00066 * @param buffer Buffer to write blocks to 00067 * @param address Address of block to begin reading from 00068 * @param size Size to read in bytes, must be a multiple of read block size 00069 * @return 0 on success, negative error code on failure 00070 */ 00071 int32_t arm_uc_blockdevice_read(uint8_t* buffer, 00072 uint64_t address, 00073 uint32_t size); 00074 00075 /** Get the size of a programable block 00076 * 00077 * @return Size of a programable block in bytes 00078 * @note Must be a multiple of the read size 00079 */ 00080 uint32_t arm_uc_blockdevice_get_program_size(void); 00081 00082 /** Get the size of a eraseable block 00083 * 00084 * @return Size of a eraseable block in bytes 00085 * @note Must be a multiple of the program size 00086 */ 00087 uint32_t arm_uc_blockdevice_get_erase_size(void); 00088 00089 #ifdef __cplusplus 00090 } 00091 #endif 00092 00093 #endif /* ARM_UC_PAL_BLOCKDEVICE_PLATFORM_H */
Generated on Tue Jul 12 2022 19:12:11 by
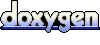