
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_pal_blockdevice_mbed.cpp
00001 //---------------------------------------------------------------------------- 00002 // The confidential and proprietary information contained in this file may 00003 // only be used by a person authorised under and to the extent permitted 00004 // by a subsisting licensing agreement from ARM Limited or its affiliates. 00005 // 00006 // (C) COPYRIGHT 2017 ARM Limited or its affiliates. 00007 // ALL RIGHTS RESERVED 00008 // 00009 // This entire notice must be reproduced on all copies of this file 00010 // and copies of this file may only be made by a person if such person is 00011 // permitted to do so under the terms of a subsisting license agreement 00012 // from ARM Limited or its affiliates. 00013 //---------------------------------------------------------------------------- 00014 00015 #if defined(TARGET_LIKE_MBED) && defined(ARM_UC_USE_PAL_BLOCKDEVICE) 00016 00017 #include "update-client-pal-blockdevice/arm_uc_pal_blockdevice_platform.h" 00018 #include "mbed.h" 00019 00020 extern BlockDevice* arm_uc_blockdevice; 00021 00022 int32_t arm_uc_blockdevice_init(void) 00023 { 00024 return arm_uc_blockdevice->init(); 00025 } 00026 00027 uint32_t arm_uc_blockdevice_get_program_size(void) 00028 { 00029 return arm_uc_blockdevice->get_program_size(); 00030 } 00031 00032 uint32_t arm_uc_blockdevice_get_erase_size(void) 00033 { 00034 return arm_uc_blockdevice->get_erase_size(); 00035 } 00036 00037 int32_t arm_uc_blockdevice_erase(uint64_t address, uint64_t size) 00038 { 00039 return arm_uc_blockdevice->erase(address, size); 00040 } 00041 00042 int32_t arm_uc_blockdevice_program(const uint8_t* buffer, 00043 uint64_t address, 00044 uint32_t size) 00045 { 00046 return arm_uc_blockdevice->program(buffer, address, size); 00047 } 00048 00049 int32_t arm_uc_blockdevice_read(uint8_t* buffer, 00050 uint64_t address, 00051 uint32_t size) 00052 { 00053 return arm_uc_blockdevice->read(buffer, address, size); 00054 } 00055 00056 #endif // #if defined(TARGET_LIKE_MBED) && defined(ARM_UC_USE_PAL_BLOCKDEVICE)
Generated on Tue Jul 12 2022 19:12:11 by
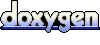