
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_pal_blockdevice.c
00001 //---------------------------------------------------------------------------- 00002 // The confidential and proprietary information contained in this file may 00003 // only be used by a person authorised under and to the extent permitted 00004 // by a subsisting licensing agreement from ARM Limited or its affiliates. 00005 // 00006 // (C) COPYRIGHT 2016 ARM Limited or its affiliates. 00007 // ALL RIGHTS RESERVED 00008 // 00009 // This entire notice must be reproduced on all copies of this file 00010 // and copies of this file may only be made by a person if such person is 00011 // permitted to do so under the terms of a subsisting license agreement 00012 // from ARM Limited or its affiliates. 00013 //---------------------------------------------------------------------------- 00014 00015 #if defined(ARM_UC_USE_PAL_BLOCKDEVICE) 00016 00017 #include "update-client-paal/arm_uc_paal_update_api.h" 00018 00019 #include "update-client-pal-blockdevice/arm_uc_pal_blockdevice_implementation.h" 00020 #include "update-client-pal-flashiap/arm_uc_pal_flashiap_implementation.h" 00021 00022 /** 00023 * @brief Initialize the underlying storage and set the callback handler. 00024 * 00025 * @param callback Function pointer to event handler. 00026 * @return Returns ERR_NONE on accept, and signals the event handler with 00027 * either DONE or ERROR when complete. 00028 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00029 */ 00030 arm_uc_error_t ARM_UCP_FashIAP_BlockDevice_Initialize(ARM_UC_PAAL_UPDATE_SignalEvent_t callback) 00031 { 00032 arm_uc_error_t result = { .code = ERR_INVALID_PARAMETER }; 00033 00034 if (callback) 00035 { 00036 arm_uc_error_t status1 = ARM_UC_PAL_FlashIAP_Initialize(callback); 00037 arm_uc_error_t status2 = ARM_UC_PAL_BlockDevice_Initialize(callback); 00038 00039 if ((status1.error == ERR_NONE) && (status2.error == ERR_NONE)) 00040 { 00041 result.code = ERR_NONE; 00042 } 00043 else 00044 { 00045 result.code = ERR_NOT_READY; 00046 } 00047 } 00048 00049 return result; 00050 } 00051 00052 ARM_UC_PAAL_UPDATE_CAPABILITIES ARM_UCP_FashIAP_BlockDevice_GetCapabilities(void) 00053 { 00054 ARM_UC_PAAL_UPDATE_CAPABILITIES result = { 00055 .installer_arm_hash = 0, 00056 .installer_oem_hash = 0, 00057 .installer_layout = 0, 00058 .firmware_hash = 1, 00059 .firmware_hmac = 0, 00060 .firmware_campaign = 0, 00061 .firmware_version = 1, 00062 .firmware_size = 1 00063 }; 00064 00065 return result; 00066 } 00067 00068 const ARM_UC_PAAL_UPDATE ARM_UCP_FLASHIAP_BLOCKDEVICE = 00069 { 00070 .Initialize = ARM_UCP_FashIAP_BlockDevice_Initialize, 00071 .GetCapabilities = ARM_UCP_FashIAP_BlockDevice_GetCapabilities, 00072 .GetMaxID = ARM_UC_PAL_BlockDevice_GetMaxID, 00073 .Prepare = ARM_UC_PAL_BlockDevice_Prepare, 00074 .Write = ARM_UC_PAL_BlockDevice_Write, 00075 .Finalize = ARM_UC_PAL_BlockDevice_Finalize, 00076 .Read = ARM_UC_PAL_BlockDevice_Read, 00077 .Activate = ARM_UC_PAL_BlockDevice_Activate, 00078 .GetActiveFirmwareDetails = ARM_UC_PAL_FlashIAP_GetActiveDetails, 00079 .GetFirmwareDetails = ARM_UC_PAL_BlockDevice_GetFirmwareDetails, 00080 .GetInstallerDetails = ARM_UC_PAL_FlashIAP_GetInstallerDetails 00081 }; 00082 00083 #endif // #if defined(ARM_UC_USE_PAL_BLOCKDEVICE)
Generated on Tue Jul 12 2022 19:12:11 by
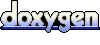