
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_paal_update.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "update-client-paal/arm_uc_paal_update_api.h" 00020 00021 /* Not including arm_uc_common.h to avoid the scheduler from being 00022 included in the mbed-bootloader. 00023 */ 00024 #include "update-client-common/arm_uc_error.h" 00025 #include <stddef.h> 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 /** 00032 * @brief Set PAAL Update implementation. 00033 * 00034 * @param implementation Function pointer struct to implementation. 00035 * @return Returns ERR_NONE on accept and ERR_INVALID_PARAMETER otherwise. 00036 */ 00037 arm_uc_error_t ARM_UCP_SetPAALUpdate(const ARM_UC_PAAL_UPDATE* implementation); 00038 00039 /** 00040 * @brief Initialize the underlying storage and set the callback handler. 00041 * 00042 * @param callback Function pointer to event handler. 00043 * @return Returns ERR_NONE on accept, and signals the event handler with 00044 * either DONE or ERROR when complete. 00045 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00046 */ 00047 arm_uc_error_t ARM_UCP_Initialize(ARM_UC_PAAL_UPDATE_SignalEvent_t callback); 00048 00049 /** 00050 * @brief Get a bitmap indicating supported features. 00051 * @details The bitmap is used in conjunction with the firmware and 00052 * installer details struct to indicate what fields are supported 00053 * and which values are valid. 00054 * 00055 * @return Capability bitmap. 00056 */ 00057 ARM_UC_PAAL_UPDATE_CAPABILITIES ARM_UCP_GetCapabilities(void); 00058 00059 /** 00060 * @brief Get maximum number of supported storage locations. 00061 * 00062 * @return Number of storage locations. 00063 */ 00064 uint32_t ARM_UCP_GetMaxID(void); 00065 00066 /** 00067 * @brief Prepare the storage layer for a new firmware image. 00068 * @details The storage location is set up to receive an image with 00069 * the details passed in the details struct. 00070 * 00071 * @param location Storage location ID. 00072 * @param details Pointer to a struct with firmware details. 00073 * @param buffer Temporary buffer for formatting and storing metadata. 00074 * @return Returns ERR_NONE on accept, and signals the event handler with 00075 * either DONE or ERROR when complete. 00076 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00077 */ 00078 arm_uc_error_t ARM_UCP_Prepare(uint32_t location, 00079 const arm_uc_firmware_details_t* details, 00080 arm_uc_buffer_t* buffer); 00081 00082 /** 00083 * @brief Write a fragment to the indicated storage location. 00084 * @details The storage location must have been allocated using the Prepare 00085 * call. The call is expected to write the entire fragment before 00086 * signaling completion. 00087 * 00088 * @param location Storage location ID. 00089 * @param offset Offset in bytes to where the fragment should be written. 00090 * @param buffer Pointer to buffer struct with fragment. 00091 * @return Returns ERR_NONE on accept, and signals the event handler with 00092 * either DONE or ERROR when complete. 00093 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00094 */ 00095 arm_uc_error_t ARM_UCP_Write(uint32_t location, 00096 uint32_t offset, 00097 const arm_uc_buffer_t* buffer); 00098 00099 /** 00100 * @brief Close storage location for writing and flush pending data. 00101 * 00102 * @param location Storage location ID. 00103 * @return Returns ERR_NONE on accept, and signals the event handler with 00104 * either DONE or ERROR when complete. 00105 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00106 */ 00107 arm_uc_error_t ARM_UCP_Finalize(uint32_t location); 00108 00109 /** 00110 * @brief Read a fragment from the indicated storage location. 00111 * @details The function will read until the buffer is full or the end of 00112 * the storage location has been reached. The actual amount of 00113 * bytes read is set in the buffer struct. 00114 * 00115 * @param location Storage location ID. 00116 * @param offset Offset in bytes to read from. 00117 * @param buffer Pointer to buffer struct to store fragment. buffer->size 00118 * contains the intended read size. 00119 * @return Returns ERR_NONE on accept, and signals the event handler with 00120 * either DONE or ERROR when complete. 00121 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00122 * buffer->size contains actual bytes read on return. 00123 */ 00124 arm_uc_error_t ARM_UCP_Read(uint32_t location, 00125 uint32_t offset, 00126 arm_uc_buffer_t* buffer); 00127 00128 /** 00129 * @brief Set the firmware image in the slot to be the new active image. 00130 * @details This call is responsible for initiating the process for 00131 * applying a new/different image. Depending on the platform this 00132 * could be: 00133 * * An empty call, if the installer can deduce which slot to 00134 * choose from based on the firmware details. 00135 * * Setting a flag to indicate which slot to use next. 00136 * * Decompressing/decrypting/installing the firmware image on 00137 * top of another. 00138 * 00139 * @param location Storage location ID. 00140 * @return Returns ERR_NONE on accept, and signals the event handler with 00141 * either DONE or ERROR when complete. 00142 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00143 */ 00144 arm_uc_error_t ARM_UCP_Activate(uint32_t location); 00145 00146 /** 00147 * @brief Get firmware details for the actively running firmware. 00148 * @details This call populates the passed details struct with information 00149 * about the currently active firmware image. Only the fields 00150 * marked as supported in the capabilities bitmap will have valid 00151 * values. 00152 * 00153 * @param details Pointer to firmware details struct to be populated. 00154 * @return Returns ERR_NONE on accept, and signals the event handler with 00155 * either DONE or ERROR when complete. 00156 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00157 */ 00158 arm_uc_error_t ARM_UCP_GetActiveFirmwareDetails(arm_uc_firmware_details_t* details); 00159 00160 /** 00161 * @brief Get firmware details for the firmware image in the slot passed. 00162 * @details This call populates the passed details struct with information 00163 * about the firmware image in the slot passed. Only the fields 00164 * marked as supported in the capabilities bitmap will have valid 00165 * values. 00166 * 00167 * @param details Pointer to firmware details struct to be populated. 00168 * @return Returns ERR_NONE on accept, and signals the event handler with 00169 * either DONE or ERROR when complete. 00170 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00171 */ 00172 arm_uc_error_t ARM_UCP_GetFirmwareDetails(uint32_t location, 00173 arm_uc_firmware_details_t* details); 00174 00175 /** 00176 * @brief Get details for the component responsible for installation. 00177 * @details This call populates the passed details struct with information 00178 * about the local installer. Only the fields marked as supported 00179 * in the capabilities bitmap will have valid values. The 00180 * installer could be the bootloader, a recovery image, or some 00181 * other component responsible for applying the new firmware 00182 * image. 00183 * 00184 * @param details Pointer to installer details struct to be populated. 00185 * @return Returns ERR_NONE on accept, and signals the event handler with 00186 * either DONE or ERROR when complete. 00187 * Returns ERR_INVALID_PARAMETER on reject, and no signal is sent. 00188 */ 00189 arm_uc_error_t ARM_UCP_GetInstallerDetails(arm_uc_installer_details_t* details); 00190 00191 #ifdef __cplusplus 00192 } 00193 #endif
Generated on Tue Jul 12 2022 19:12:11 by
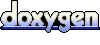