
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_mmGetLatestTimestamp.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "arm_uc_mmConfig.h" 00020 00021 #include "arm_uc_mmGetLatestTimestamp.h" 00022 #include "update-client-manifest-manager/update-client-manifest-manager-context.h" 00023 #if !MANIFEST_MANAGER_NO_STORAGE 00024 #include "cfstore-fsm.h" 00025 #endif 00026 00027 #include "update-client-common/arm_uc_error.h" 00028 #include "update-client-common/arm_uc_types.h" 00029 00030 #define ARM_UC_MM_GET_LATEST_TS_STATE_LIST\ 00031 ENUM_FIXED(ARM_UC_MM_GET_LATEST_TS_STATE_INVALID,0)\ 00032 ENUM_AUTO(ARM_UC_MM_GET_LATEST_TS_STATE_BEGIN)\ 00033 ENUM_AUTO(ARM_UC_MM_GET_LATEST_TS_STATE_FIND)\ 00034 ENUM_AUTO(ARM_UC_MM_GET_LATEST_TS_STATE_READ)\ 00035 ENUM_AUTO(ARM_UC_MM_GET_LATEST_TS_STATE_FETCH_NAME)\ 00036 00037 enum arm_uc_mm_get_latest_ts_state { 00038 #define ENUM_AUTO(name) name, 00039 #define ENUM_FIXED(name, val) name = val, 00040 ARM_UC_MM_GET_LATEST_TS_STATE_LIST 00041 #undef ENUM_AUTO 00042 #undef ENUM_FIXED 00043 }; 00044 00045 const char* ARM_UC_mmGetLatestTsState2Str(uint32_t state) 00046 { 00047 switch (state) { 00048 #define ENUM_AUTO(name) case name: return #name; 00049 #define ENUM_FIXED(name, val) ENUM_AUTO(name) 00050 ARM_UC_MM_GET_LATEST_TS_STATE_LIST 00051 #undef ENUM_FIXED 00052 #undef ENUM_AUTO 00053 default: 00054 return "Unknown State"; 00055 } 00056 } 00057 00058 /** 00059 * @brief Search the key/value store to find the latest timestamp. 00060 * @detail Searches for all *.ts entries in the manifest manager key/value store prefix. As each entry is found, 00061 * `getLatestManifestTimestampFSM()` updates ts with the largest timestamp encountered. If key is non-NULL, it is 00062 * populated with the path to the largest timestamp. 00063 * 00064 * @param[out] ts Pointer to a 64-bit unsigned integer. Contains the largest timestamp encountered when 00065 * getLatestManifestTimestampFSM completes. 00066 * @param[out] key Pointer to a buffer; the location to store the key that contained the largest timestamp. 00067 * @retval MFST_ERR_NONE Always returns success. 00068 */ 00069 arm_uc_error_t getLatestManifestTimestamp(uint64_t *ts, arm_uc_buffer_t* key) 00070 { 00071 *ts = 0; 00072 return (arm_uc_error_t){MFST_ERR_NONE}; 00073 } 00074 00075 /** 00076 * @brief Run the getLatestManifestTimestampstate machine. 00077 * @details Processes through the getLatestManifestTimestamp state machine in response to received events 00078 * 00079 * @param[in] event The event which has caused this run through the state machine 00080 * @retval MFST_ERR_NONE getLatestManifestTimestampFSM has completed 00081 * @retval MFST_ERR_PENDING getLatestManifestTimestampFSM is still on-going and waiting for an event 00082 * @return any other error code indicates an error has occurred 00083 */ 00084 arm_uc_error_t getLatestManifestTimestampFSM(uint32_t event) 00085 { 00086 return (arm_uc_error_t){MFST_ERR_NONE}; 00087 }
Generated on Tue Jul 12 2022 19:12:11 by
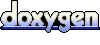