
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_metadata_header_v2.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef ARM_UC_METADATA_HEADER_V2_H 00020 #define ARM_UC_METADATA_HEADER_V2_H 00021 00022 #include "update-client-common/arm_uc_types.h" 00023 #include "update-client-common/arm_uc_error.h" 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 #define ARM_UC_INTERNAL_HEADER_MAGIC_V2 (0x5a51b3d4UL) 00030 #define ARM_UC_INTERNAL_HEADER_VERSION_V2 (2) 00031 00032 #define ARM_UC_EXTERNAL_HEADER_MAGIC_V2 (0x5a51b3d4UL) 00033 #define ARM_UC_EXTERNAL_HEADER_VERSION_V2 (2) 00034 00035 #define ARM_UC_INTERNAL_FIRMWARE_VERSION_OFFSET_V2 (8) 00036 #define ARM_UC_INTERNAL_FIRMWARE_SIZE_OFFSET_V2 (16) 00037 #define ARM_UC_INTERNAL_FIRMWARE_HASH_OFFSET_V2 (24) 00038 #define ARM_UC_INTERNAL_CAMPAIGN_OFFSET_V2 (88) 00039 #define ARM_UC_INTERNAL_SIGNATURE_SIZE_OFFSET_V2 (104) 00040 #define ARM_UC_INTERNAL_HEADER_CRC_OFFSET_V2 (108) 00041 00042 #define ARM_UC_INTERNAL_HEADER_SIZE_V2 (112) 00043 00044 #define ARM_UC_EXTERNAL_FIRMWARE_VERSION_OFFSET_V2 (8) 00045 #define ARM_UC_EXTERNAL_FIRMWARE_SIZE_OFFSET_V2 (16) 00046 #define ARM_UC_EXTERNAL_FIRMWARE_HASH_OFFSET_V2 (24) 00047 #define ARM_UC_EXTERNAL_PAYLOAD_SIZE_OFFSET_V2 (88) 00048 #define ARM_UC_EXTERNAL_PAYLOAD_HASH_OFFSET_V2 (96) 00049 #define ARM_UC_EXTERNAL_CAMPAIGN_OFFSET_V2 (160) 00050 #define ARM_UC_EXTERNAL_HMAC_OFFSET_V2 (232) 00051 00052 #define ARM_UC_EXTERNAL_HEADER_SIZE_V2 (296) 00053 00054 typedef struct _arm_uc_internal_header_t 00055 { 00056 /* Metadata-header specific magic code */ 00057 uint32_t headerMagic; 00058 00059 /* Revision number for metadata header. */ 00060 uint32_t headerVersion; 00061 00062 /* Version number accompanying the firmware. Larger numbers imply more 00063 recent and preferred versions. This is used for determining the 00064 selection order when multiple versions are available. For downloaded 00065 firmware the manifest timestamp is used as the firmware version. 00066 */ 00067 uint64_t firmwareVersion; 00068 00069 /* Total space (in bytes) occupied by the firmware BLOB. */ 00070 uint64_t firmwareSize; 00071 00072 /* Firmware hash calculated over the firmware size. Should match the hash 00073 generated by standard command line tools, e.g., shasum on Linux/Mac. 00074 */ 00075 uint8_t firmwareHash[ARM_UC_SHA512_SIZE]; 00076 00077 /* The ID for the update campaign that resulted in the firmware update. 00078 */ 00079 uint8_t campaign[ARM_UC_GUID_SIZE]; 00080 00081 /* Size of the firmware signature. Must be 0 if no signature is supplied. */ 00082 uint32_t firmwareSignatureSize; 00083 00084 /* Header 32 bit CRC. Calculated over the entire header, including the CRC 00085 field, but with the CRC set to zero. 00086 */ 00087 uint32_t headerCRC; 00088 00089 /* Optional firmware signature. Hashing algorithm should be the same as the 00090 one used for the firmware hash. The firmwareSignatureSize must be set. 00091 */ 00092 uint8_t firmwareSignature[0]; 00093 } arm_uc_internal_header_t; 00094 00095 typedef struct _arm_uc_external_header_t 00096 { 00097 /* Metadata-header specific magic code */ 00098 uint32_t headerMagic; 00099 00100 /* Revision number for metadata header. */ 00101 uint32_t headerVersion; 00102 00103 /* Version number accompanying the firmware. Larger numbers imply more 00104 recent and preferred versions. This is used for determining the 00105 selection order when multiple versions are available. For downloaded 00106 firmware the manifest timestamp is used as the firmware version. 00107 */ 00108 uint64_t firmwareVersion; 00109 00110 /* Total space (in bytes) occupied by the firmware BLOB. */ 00111 uint64_t firmwareSize; 00112 00113 /* Firmware hash calculated over the firmware size. Should match the hash 00114 generated by standard command line tools, e.g., shasum on Linux/Mac. 00115 */ 00116 uint8_t firmwareHash[ARM_UC_SHA512_SIZE]; 00117 00118 /* Total space (in bytes) occupied by the payload BLOB. 00119 The payload is the firmware after some form of transformation like 00120 encryption and/or compression. 00121 */ 00122 uint64_t payloadSize; 00123 00124 /* Payload hash calculated over the payload size. Should match the hash 00125 generated by standard command line tools, e.g., shasum on Linux/Mac. 00126 The payload is the firmware after some form of transformation like 00127 encryption and/or compression. 00128 */ 00129 uint8_t payloadHash[ARM_UC_SHA512_SIZE]; 00130 00131 /* The ID for the update campaign that resulted in the firmware update. 00132 */ 00133 uint8_t campaign[ARM_UC_GUID_SIZE]; 00134 00135 /* Type of transformation used to turn the payload into the firmware image. 00136 Possible values are: 00137 * * NONE 00138 * * AES128_CTR 00139 * * AES128_CBC 00140 * * AES256_CTR 00141 * * AES256_CBC 00142 */ 00143 uint32_t firmwareTransformationMode; 00144 00145 /* Encrypted firmware encryption key. 00146 * To decrypt the firmware, the bootloader combines the bootloader secret 00147 * and the firmwareKeyDerivationFunctionSeed to create an AES key. It uses 00148 * This AES key to decrypt the firmwareCipherKey. The decrypted 00149 * firmwareCipherKey is the FirmwareKey, which is used with the 00150 * firmwareInitVector to decrypt the firmware. 00151 */ 00152 uint8_t firmwareCipherKey[ARM_UC_AES256_KEY_SIZE]; 00153 00154 /* AES Initialization vector. This is a random number used to protect the 00155 encryption algorithm from attack. It must be unique for every firmware. 00156 */ 00157 uint8_t firmwareInitVector[ARM_UC_AES_BLOCK_SIZE]; 00158 00159 /* Size of the firmware signature. Must be 0 if no signature is supplied. */ 00160 uint32_t firmwareSignatureSize; 00161 00162 /* Hash based message authentication code for the metadata header. Uses per 00163 device secret as key. Should use same hash algorithm as firmware hash. 00164 The headerHMAC field and firmwareSignature field are not part of the hash. 00165 */ 00166 uint8_t headerHMAC[ARM_UC_SHA512_SIZE]; 00167 00168 /* Optional firmware signature. Hashing algorithm should be the same as the 00169 one used for the firmware hash. The firmwareSignatureSize must be set. 00170 */ 00171 uint8_t firmwareSignature[0]; 00172 } arm_uc_external_header_t; 00173 00174 arm_uc_error_t arm_uc_parse_internal_header_v2(const uint8_t* input, 00175 arm_uc_firmware_details_t* output); 00176 00177 arm_uc_error_t arm_uc_create_internal_header_v2(const arm_uc_firmware_details_t* input, 00178 arm_uc_buffer_t* output); 00179 00180 arm_uc_error_t arm_uc_parse_external_header_v2(const uint8_t* input, 00181 arm_uc_firmware_details_t* output); 00182 00183 arm_uc_error_t arm_uc_create_external_header_v2(const arm_uc_firmware_details_t* input, 00184 arm_uc_buffer_t* output); 00185 00186 #ifdef __cplusplus 00187 } 00188 #endif 00189 00190 #endif // ARM_UC_METADATA_HEADER_V2_H
Generated on Tue Jul 12 2022 19:12:11 by
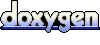