
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_http_socket_private.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef __ARM_UC_HTTP_SOCKET_PRIVATE_H__ 00020 #define __ARM_UC_HTTP_SOCKET_PRIVATE_H__ 00021 00022 #include "update-client-source-http-socket/arm_uc_http_socket.h" 00023 #include "update-client-common/arm_uc_common.h" 00024 #include <pal.h> 00025 00026 /** 00027 * @brief Initialize Http module. 00028 * @details A memory struct is passed as well as a function pointer for event 00029 * handling. 00030 * 00031 * @param context Struct holding all global variables. 00032 * @param handler Event handler for signaling when each operation is complete. 00033 * @return Error code. 00034 */ 00035 arm_uc_error_t arm_uc_socket_initialize(arm_uc_http_socket_context_t* context, 00036 ARM_UCS_HttpEvent_t handler); 00037 00038 /** 00039 * @brief Resets HTTP socket to uninitialized state and clears memory struct. 00040 * @details HTTP sockets must be initialized again before use. 00041 * @return Error code. 00042 */ 00043 arm_uc_error_t arm_uc_socket_terminate(void); 00044 00045 /** 00046 * @brief Get resource at URI. 00047 * @details Download resource at URI from given offset and store in buffer. 00048 * Events are generated when download finish or on error 00049 * 00050 * @param uri Pointer to structure with resource location. 00051 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00052 * @param offset Offset in resource to begin download from. 00053 * @param type Indicate what type of request that was initiated. 00054 * @return Error code. 00055 */ 00056 arm_uc_error_t arm_uc_socket_get(arm_uc_uri_t* uri, 00057 arm_uc_buffer_t* buffer, 00058 uint32_t offset, 00059 arm_uc_rqst_t type); 00060 00061 /** 00062 * @brief Connect to server set in the global URI struct. 00063 * @details Connecting generates a socket event, which automatically processes 00064 * the request passed in arm_uc_socket_get. If a DNS request must 00065 * be made, this call initiates an asynchronous DNS request. After 00066 * the request is done, the connection process will be resumed in 00067 * arm_uc_socket_finish_connect(). 00068 * @return Error code. 00069 */ 00070 arm_uc_error_t arm_uc_socket_connect(void); 00071 00072 /** 00073 * @brief Finishes connecting to the server requested in a previous call to 00074 * arm_uc_socket_connect(). 00075 * @details This function is called after the DNS resolution for the host 00076 * requested in arm_uc_socket_get() above is done. It finishes the 00077 * connection process by creating a socket and connecting it. 00078 * @return Error code. 00079 */ 00080 arm_uc_error_t arm_uc_socket_finish_connect(void); 00081 00082 /** 00083 * @brief Send request passed in arm_uc_socket_get. 00084 * @details This call assumes the HTTP socket is already connected to server. 00085 * @return Error code. 00086 */ 00087 arm_uc_error_t arm_uc_socket_send_request(void); 00088 00089 /** 00090 * @brief Receive data from HTTP socket. 00091 * @details Data is stored in global buffer. The call will automatically retry 00092 * if the socket is busy. 00093 */ 00094 void arm_uc_socket_receive(void); 00095 00096 /** 00097 * @brief Function is called when some data has been received but an HTTP 00098 * header has yet to be processed. 00099 * @details Function is called repeatedly until a header is found or the buffer 00100 * is full. Once a header is found, the ETag, date, or content length 00101 * is parsed. For file and fragment downloads the receive process is 00102 * restarted and the header is erased. 00103 */ 00104 void arm_uc_socket_process_header(void); 00105 00106 /** 00107 * @brief Function is called when file or fragment is being downloaded. 00108 * @details Function drives the download and continues until the buffer is full 00109 * or the expected amount of data has been downloaded. 00110 */ 00111 void arm_uc_socket_process_body(void); 00112 00113 /** 00114 * @brief Close socket and set internal state to disconnected. 00115 */ 00116 void arm_uc_socket_close(void); 00117 00118 /** 00119 * @brief Close socket, set internal state to disconnected and generate error 00120 * event. 00121 * @param error The code of the error event. 00122 */ 00123 void arm_uc_socket_error(arm_ucs_http_event_t error); 00124 00125 /** 00126 * @brief Callback function for handling events in application context. 00127 * @param unused Unused. 00128 */ 00129 void arm_uc_socket_callback(uint32_t unused); 00130 00131 /** 00132 * @brief Callback function for handling events in interrupt context. 00133 * @details All events are de-escalated through the callback queue. 00134 */ 00135 void arm_uc_socket_isr(void*); 00136 00137 /** 00138 * @brief Callback handler for the socket timeout timer callback. 00139 * Callbacks go through the task queue because we don't know 00140 * what context we are running from. 00141 */ 00142 void arm_uc_timeout_timer_callback(void const *); 00143 00144 /** 00145 * @brief Callback handler for the asynchronous DNS resolver. 00146 * Callbacks go through the task queue because we don't know 00147 * what context we are running from. 00148 */ 00149 void arm_uc_dns_callback(const char* url, 00150 palSocketAddress_t* address, 00151 palSocketLength_t* address_length, 00152 palStatus_t status, 00153 void* argument); 00154 00155 #endif // __ARM_UC_HTTP_SOCKET_PRIVATE_H__
Generated on Tue Jul 12 2022 19:12:11 by
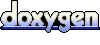