
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_http_socket.h
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #ifndef UPDATE_CLIENT_SOURCE_HTTP_SOCKET_H 00020 #define UPDATE_CLIENT_SOURCE_HTTP_SOCKET_H 00021 00022 #include "update-client-common/arm_uc_common.h" 00023 00024 #include <pal.h> 00025 00026 /** 00027 * @brief Events passed to event handler. 00028 * @details EVENT_HASH Get hash complete. 00029 * EVENT_DATE Get date complete. 00030 * EVENT_DOWNLOAD_PENDING Download complete with data pending. 00031 * EVENT_DOWNLOAD_DONE Download complete, all done. 00032 */ 00033 typedef enum { 00034 UCS_HTTP_EVENT_HASH, 00035 UCS_HTTP_EVENT_DATE, 00036 UCS_HTTP_EVENT_DOWNLOAD, 00037 UCS_HTTP_EVENT_ERROR, 00038 UCS_HTTP_EVENT_ERROR_BUFFER_SIZE 00039 } arm_ucs_http_event_t; 00040 00041 typedef enum { 00042 RQST_TYPE_NONE, // to indicate idle 00043 RQST_TYPE_HASH_ETAG, 00044 RQST_TYPE_HASH_DATE, 00045 RQST_TYPE_GET_FILE, 00046 RQST_TYPE_GET_FRAG 00047 } arm_uc_rqst_t; 00048 00049 typedef enum { 00050 STATE_DISCONNECTED, 00051 STATE_PROCESS_HEADER, 00052 STATE_PROCESS_BODY, 00053 STATE_CONNECTED_IDLE 00054 } arm_uc_socket_state_t; 00055 00056 typedef enum { 00057 SOCKET_EVENT_DNS_DONE, 00058 SOCKET_EVENT_CONNECT_DONE, 00059 SOCKET_EVENT_SEND_DONE, 00060 SOCKET_EVENT_RECEIVE_CONTINUE, 00061 SOCKET_EVENT_UNDEFINED, 00062 SOCKET_EVENT_TIMER_FIRED 00063 } arm_uc_socket_event_t; 00064 00065 /** 00066 * @brief Prototype for event handler. 00067 */ 00068 typedef void (*ARM_UCS_HttpEvent_t)(uint32_t event); 00069 00070 typedef struct { 00071 /* external callback handler */ 00072 ARM_UCS_HttpEvent_t callback_handler; 00073 00074 /* location */ 00075 arm_uc_uri_t* request_uri; 00076 00077 /* buffer to store downloaded data */ 00078 arm_uc_buffer_t* request_buffer; 00079 00080 /* fragment offset in a multi-fragment download */ 00081 uint32_t request_offset; 00082 00083 /* request type */ 00084 arm_uc_rqst_t request_type; 00085 00086 /* internal state */ 00087 arm_uc_socket_state_t socket_state; 00088 00089 /* expected socket event */ 00090 arm_uc_socket_event_t expected_event; 00091 00092 /* remaining bytes in request */ 00093 uint32_t expected_remaining; 00094 00095 /* structs for callback queue */ 00096 int32_t isr_callback_counter; 00097 arm_uc_callback_t isr_callback_struct; // initialized in source-http 00098 arm_uc_callback_t event_callback_struct; // initialized in source-http 00099 arm_uc_callback_t timer_callback_struct; // initialized in source-http 00100 00101 /* pointer to socket */ 00102 palSocket_t socket; 00103 00104 /* timer id for the socket timeout timer */ 00105 palTimerID_t timeout_timer_id; 00106 00107 /* cache for storing DNS lookup */ 00108 palSocketAddress_t cache_address; 00109 palSocketLength_t cache_address_length; 00110 } arm_uc_http_socket_context_t; 00111 00112 /** 00113 * @brief Initialize Http module. 00114 * @details A memory struct is passed as well as a function pointer for event 00115 * handling. 00116 * 00117 * @param context Struct holding all global variables. 00118 * @param handler Event handler for signaling when each operation is complete. 00119 * @return Error code. 00120 */ 00121 arm_uc_error_t ARM_UCS_HttpSocket_Initialize(arm_uc_http_socket_context_t* context, 00122 ARM_UCS_HttpEvent_t handler); 00123 00124 /** 00125 * @brief Resets HTTP socket to uninitialized state and clears memory struct. 00126 * @details HTTP sockets must be initialized again before use. 00127 * @return Error code. 00128 */ 00129 arm_uc_error_t ARM_UCS_HttpSocket_Terminate(void); 00130 00131 /** 00132 * @brief Get hash for resource at URI. 00133 * @details Store hash in provided buffer. Enclosing "" and '\0' are removed. 00134 * 00135 * Event generated: EVENT_HASH 00136 * 00137 * @param uri Pointer to struct with resource location. 00138 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00139 * @return Error code. 00140 */ 00141 arm_uc_error_t ARM_UCS_HttpSocket_GetHash(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer); 00142 00143 /** 00144 * @brief Get date for resource at URI. 00145 * @details Store Last-Modified data in provided buffer. Enclosing "" and '\0' are removed. 00146 * 00147 * Event generated: EVENT_DATE 00148 * 00149 * @param uri Pointer to struct with resource location. 00150 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00151 * @return Error code. 00152 */ 00153 arm_uc_error_t ARM_UCS_HttpSocket_GetDate(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer); 00154 00155 /** 00156 * @brief Get full resource at URI. 00157 * @details Download resource at URI and store in provided buffer. 00158 * If the provided buffer is not big enough to contain the whole resource 00159 * what can fit in the buffer will be downloaded. 00160 * The user can then use GetFragment to download the rest. 00161 00162 * Events generated: EVENT_DOWNLOAD_PENDING if there is still data to 00163 * download and EVENT_DOWNLOAD_DONE if the file is completely downloaded. 00164 * 00165 * @param uri Pointer to structure with resource location. 00166 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00167 * @return Error code. 00168 */ 00169 arm_uc_error_t ARM_UCS_HttpSocket_GetFile(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer); 00170 00171 /** 00172 * @brief Get partial resource at URI. 00173 * @details Download resource at URI from given offset and store in buffer. 00174 * 00175 * The buffer maxSize determines how big a fragment to download. If the 00176 * buffer is larger than the requested fragment (offset to end-of-file) 00177 * the buffer size is set to indicate the number of available bytes. 00178 * 00179 * Events generated: EVENT_DOWNLOAD_PENDING if there is still data to 00180 * download and EVENT_DOWNLOAD_DONE if the file is completely downloaded. 00181 * 00182 * @param uri Pointer to structure with resource location. 00183 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00184 * @param offset Offset in resource to begin download from. 00185 * @return Error code. 00186 */ 00187 arm_uc_error_t ARM_UCS_HttpSocket_GetFragment(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer, uint32_t offset); 00188 00189 #endif /* UPDATE_CLIENT_SOURCE_HTTP_SOCKET_H */
Generated on Tue Jul 12 2022 19:12:11 by
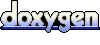