
Simulated product dispenser
Fork of mbed-cloud-workshop-connect-HTS221 by
arm_uc_http_socket.c
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2017 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 00019 #include "update-client-source-http-socket/arm_uc_http_socket.h" 00020 #include "update-client-common/arm_uc_common.h" 00021 00022 #include "arm_uc_http_socket_private.h" 00023 00024 /*****************************************************************************/ 00025 /* Public Function */ 00026 /*****************************************************************************/ 00027 00028 /** 00029 * @brief Initialize Http module. 00030 * @details Function pointer to event handler is passed as argument. 00031 * 00032 * @param context Struct holding all global variables. 00033 * @param handler Event handler for signaling when each operation is complete. 00034 * @return Error code. 00035 */ 00036 arm_uc_error_t ARM_UCS_HttpSocket_Initialize(arm_uc_http_socket_context_t* context, 00037 ARM_UCS_HttpEvent_t handler) 00038 { 00039 return arm_uc_socket_initialize(context, handler); 00040 } 00041 00042 /** 00043 * @brief Resets HTTP socket to uninitialized state and clears memory struct. 00044 * @details HTTP sockets must be initialized again before use. 00045 * @return Error code. 00046 */ 00047 arm_uc_error_t ARM_UCS_HttpSocket_Terminate() 00048 { 00049 return arm_uc_socket_terminate(); 00050 } 00051 00052 /** 00053 * @brief Get hash for resource at URI. 00054 * @details Store hash in provided buffer. Enclosing "" and '\0' are removed. 00055 * 00056 * Event generated: EVENT_HASH 00057 * 00058 * @param uri Pointer to struct with resource location. 00059 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00060 * @return Error code. 00061 */ 00062 arm_uc_error_t ARM_UCS_HttpSocket_GetHash(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer) 00063 { 00064 return arm_uc_socket_get(uri, buffer, UINT32_MAX, RQST_TYPE_HASH_ETAG); 00065 } 00066 00067 /** 00068 * @brief Get date for resource at URI. 00069 * @details Store Last-Modified data in provided buffer. Enclosing "" and '\0' are removed. 00070 * 00071 * Event generated: EVENT_DATE 00072 * 00073 * @param uri Pointer to struct with resource location. 00074 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00075 * @return Error code. 00076 */ 00077 arm_uc_error_t ARM_UCS_HttpSocket_GetDate(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer) 00078 { 00079 return arm_uc_socket_get(uri, buffer, UINT32_MAX, RQST_TYPE_HASH_DATE); 00080 } 00081 00082 /** 00083 * @brief Get full resource at URI. 00084 * @details Download resource at URI and store in provided buffer. 00085 * If the provided buffer is not big enough to contain the whole resource 00086 * what can fit in the buffer will be downloaded. 00087 * The user can then use GetFragment to download the rest. 00088 00089 * Events generated: EVENT_DOWNLOAD_PENDING if there is still data to 00090 * download and EVENT_DOWNLOAD_DONE if the file is completely downloaded. 00091 * 00092 * @param uri Pointer to structure with resource location. 00093 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00094 * @return Error code. 00095 */ 00096 arm_uc_error_t ARM_UCS_HttpSocket_GetFile(arm_uc_uri_t* uri, arm_uc_buffer_t* buffer) 00097 { 00098 return arm_uc_socket_get(uri, buffer, UINT32_MAX, RQST_TYPE_GET_FILE); 00099 } 00100 00101 /** 00102 * @brief Get partial resource at URI. 00103 * @details Download resource at URI from given offset and store in buffer. 00104 * 00105 * The buffer maxSize determines how big a fragment to download. If the 00106 * buffer is larger than the requested fragment (offset to end-of-file) 00107 * the buffer size is set to indicate the number of available bytes. 00108 * 00109 * Events generated: EVENT_DOWNLOAD_PENDING if there is still data to 00110 * download and EVENT_DOWNLOAD_DONE if the file is completely downloaded. 00111 * 00112 * @param uri Pointer to structure with resource location. 00113 * @param buffer Pointer to structure with buffer location, maxSize, and size. 00114 * @param offset Offset in resource to begin download from. 00115 * @return Error code. 00116 */ 00117 arm_uc_error_t ARM_UCS_HttpSocket_GetFragment(arm_uc_uri_t* uri, 00118 arm_uc_buffer_t* buffer, 00119 uint32_t offset) 00120 { 00121 return arm_uc_socket_get(uri, buffer, offset, RQST_TYPE_GET_FRAG); 00122 }
Generated on Tue Jul 12 2022 19:12:11 by
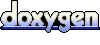